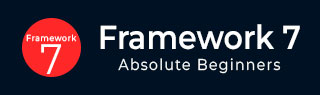
- Framework7 Tutorial
- Framework7 - Home
- Framework7 - Overview
- Framework7 - Environment
- Framework7 Components
- Framework7 - Layouts
- Framework7 - Navbars
- Framework7 - Toolbars
- Framework7 - Search Bar
- Framework7 - Status Bar
- Framework7 - Side Panels
- Framework7 - Content Block
- Framework7 - Layout Grid
- Framework7 - Overlays
- Framework7 - Preloaders
- Framework7 - Progress Bar
- Framework7 - List Views
- Framework7 - Accordion
- Framework7 - Cards
- Framework7 - Chips
- Framework7 - Buttons
- Framework7 - Action Button
- Framework7 - Forms
- Framework7 - Tabs
- Framework7 - Swiper Slider
- Framework7 - Photo Browser
- Framework7 - Autocomplete
- Framework7 - Picker
- Framework7 - Calendar
- Framework7 - Refresh
- Framework7 - Infinite Scroll
- Framework7 - Messages
- Framework7 - Message Bar
- Framework7 - Notifications
- Framework7 - Lazy Load
- Framework7 Styling
- Framework7 - Color Themes
- Framework7 - Hairlines
- Framework7 Templates
- Framework7 - Templates Overview
- Framework7 - Auto Compilation
- Framework7 - Template7 Pages
- Framework7 Fast Clicks
- Framework7 - Active State
- Framework7 - Tap Hold Event
- Framework7 - Touch Ripple
- Framework7 Useful Resources
- Framework7 - Quick Guide
- Framework7 - Useful Resources
- Framework7 - Discussion
Framework7 - Quick Guide
Framework7 - Overview
Framework7 is a free and open source framework for mobile HTML. It is used for developing hybrid mobile apps or web apps for iOS and Android devices.
Framework7 was introduced in the year 2014. The latest version 1.4.2 was released in February 2016 licensed under MIT.
Why Use Framework7?
- It is easier to develop apps for iOS and Android.
- The learning curve for Framework7 is very easy.
- It has many pre-styled widgets/components.
- It has built-in helper libraries.
Features
Framework7 is an open source and free to use framework.
Framework7 has easy and familiar jQuery syntax to get started without any delay.
To control click delay for touch UI's, Framework7 has built-in FastClick library.
Framework7 has built-in grid system layout for arranging your elements responsively.
Framework7 dynamically loads pages from templates via flexible router api.
Advantages
Framework7 is not dependent on any third party library even for DOM manipulation. Instead, it has its own custom DOM7.
Framework7 can also be used with Angular and React frameworks.
You can start creating apps once you know HTML, CSS and some basic JavaScript.
It supports faster development via Bower.
It is easy to develop apps for iOS and Android without learning it.
Disadvantages
Framework7 only supports platforms like iOS and Android.
The online community support for Framework7 framework is less compared to iOS and Andriod.
Framework7 - Environment
In this chapter, we will discuss about how to install and setup Framework7.
You can download the Framework7 in two ways −
Download from Framework7 Github repository
You can install the Framework7, using Bower as shown below −
bower install framework7
After successful installation of Framework7, you need to follow the below given steps to make use of Framework7 in your application −
Step 1 − You need to install gulp-cli to build development and dist versions of Framework7 by using the following command.
npm install gulp-cli
The cli stands for Command Line Utility for Gulp.
Step 2 − The Gulp must be installed globally by using the following command.
npm install --global gulp
Step 3 − Next, install the NodeJS package manager, which installs the node programs that makes it easier to specify and link dependencies. The following command is used to install the npm.
npm install
Step 4 − The development version of Framework7 can be built by using the following command.
npm build
Step 5 − Once you build the development version of Framework7, start building the app from dist/ folder by using the following command.
npm dist
Step 6 − Keep your app folder in the server and run the following command for navigation between pages of your app.
gulp server
Download Framework7 Library from CDNs
A CDN or Content Delivery Network is a network of servers designed to serve files to users. If you use a CDN link in your web page, it moves the responsibility of hosting files from your own servers to a series of external ones. This also offers an advantage that if a visitor to your webpage has already downloaded a copy of Framework7 from the same CDN, it won't have to be re-downloaded. You can include the following CDN files into the HTML document.
The following CDNs are used in an iOS App layout −
<link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" />
It is used to include Framework7 iOS CSS Library to your application.
<link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" />
It is used to include Framework7 iOS related color styles to your application.
The following CDNs are used in Android/Material App Layout −
<script src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script>
It is used to include Framework7 JS library to your application.
<script src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.material.min.css"></script>
It is used to include Framework7 Material styling to your application.
We are using the CDN versions of the library throughout this tutorial. We use AMPPS(AMPPS is a WAMP, MAMP and LAMP stack of Apache, MySQL, MongoDB, PHP, Perl & Python) server to execute all our examples.
Example
The following example demonstrates the use of simple application in the Framework7, which will display the alert box with the customized message when you click on the navigation bar.
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>My App</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> //you can control the background color of the Status bar <div class = "statusbar-overlay"></div> <div class = "panel-overlay"></div> <div class = "panel panel-right panel-reveal"> <div class = "content-block"> <p>Contents goes here...</p> </div> </div> <div class = "views"> <div class = "view view-main"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "center sliding">My App</div> <div class = "right"> <a href = "#" class = "link icon-only open-panel"> <i class = "icon icon-bars"></i> </a> </div> </div> </div> <div class = "pages navbar-through toolbar-through"> <div data-page = "index" class = "page"> <div class = "page-content"> <p>This is simple application...</p> <div class = "list-block"> <ul> <li> <a href = "envirmnt_about.html" class = ""> <div class = "item-content"> <div class = "item-inner"> <div class = "item-title">Blog</div> </div> </div> </a> </li> </ul> </div> </div> </div> </div> <div class = "toolbar"> <div class = "toolbar-inner"> <a href = "#" class = "link">First Link</a> <a href = "#" class = "link">Second Link</a> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> // here initialize the app var myApp = new Framework7(); // If your using custom DOM library, then save it to $$ variable var $$ = Dom7; // Add the view var mainView = myApp.addView('.view-main', { // enable the dynamic navbar for this view: dynamicNavbar: true }); //use the 'pageInit' event handler for all pages $$(document).on('pageInit', function (e) { //get page data from event data var page = e.detail.page; if (page.name === 'blog') { // you will get below message in alert box when page with data-page attribute is equal to "about" myApp.alert('Here its your About page'); } }) </script> </body> </html>
Next, create one more HTML page i.e. envirmnt_about.html as shown below −
envirmnt_about.html
<div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> <a href = "#" class = "back link"> <i class = "icon icon-back"></i> <span>Back</span> </a> </div> <div class = "center sliding">My Blog</div> <div class = "right"> <a href = "#" class = "link icon-only open-panel"> <i class = "icon icon-bars"></i> </a> </div> </div> </div> <div class = "pages"> <div data-page = "blog" class = "page"> <div class = "page-content"> <div class = "content-block"> <h2>My Blog</h2> <p>Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book.</p> </div> </div> </div> </div>
Output
Let us carry out the following steps to see how the above given code works −
Save the above HTML code as framework7_environment.html file in your server root folder.
Open this HTML file as http://localhost/framework7_environment.html and output is displayed as shown below.
When you click on the navigation bar, it will display the alert box with the customized message.
Framework7 - Layouts
Description
Framework7 provides different types of layouts for your application. It supports three types of Navbar/Toolbar layouts −
S.No | Layout Types & Description |
---|---|
1 | Static Layout
The static layout is most often used layout-type and includes navbar and toolbar, which can be a scrollable page content and each page contains its own navbar and toolbar. |
2 | Fixed Layout
Fixed layout includes its own navbar and toolbar, which can be visible on screen and cannot be scrollable on page. |
3 | Through Layout
In this layout, the navbar and the toolbar appears fixed for all pages within single view. |
4 | Mixed Layout
You can mix the different types of layouts in the single view. |
No Navbar/Toolbar
If you do not want to use navbar and toolbar, then do not include appropriate classes (navbar-fixed, navbar-through, toolbar-fixed, toolbar-through) to page/pages/view.
Framework7 - Navbars
Description
In this chapter, let us study about navbar. It is a usually placed at top of a screen containing title of the page and navigation elements.
Navbar consists of three parts each of which may contain any HTML content, but it is suggested you use these in the way given below −
Left − It is designed to place back link icons or single text link.
Center − It is used to display title of the page or tab links.
Right − This part is similar to the left part.
The following table demonstrates the use of navbar in detail −
S.No | Navbar Types & Description |
---|---|
1 | Basic navbar
A basic navbar can be created by using the navbar, navbar-inner, left, center and right classes. |
2 | Navbar with links
To use links in left and right part of your navbar, just add <a> tag with class link. |
3 | Multiple links
To use multiple links, just add few more <a class = "link"> to the part of your choice. |
4 | Links with text and icons
The links can be provided with icons and texts by adding classes for icons and wrapping the link text with <span> element. |
5 | Links with only icons
Navbar links can be provided with only icons by adding icon-only class to links. |
6 | Related app and view methods
On initializing the View, framework7 allows you to use methods available for navbar. |
7 | Hide navbar automatically
The navbar can be hidden/shown automatically for some Ajax loaded pages where navbar is not required. |
Framework7 - Toolbars
Description
Toolbar provides easy access to other pages by using navigation elements at the bottom of the screen.
You can use toolbar in two ways as specified in the table −
S.No | Toolbar types & Description |
---|---|
1 | Hide Toolbar
You can hide the toolbar automatically when you load the pages by using the no-toolbar class to loaded page. |
2 | Bottom Toolbar
Place the toolbar at the bottom of the page by using the toolbar-bottom class. |
Methods of Toolbar
The following available methods can be used with Toolbars −
S.No | Toolbar Methods & Description |
---|---|
1 | myApp.hideToolbar(toolbar) It hides the specified toolbar. |
2 | myApp.showToolbar(toolbar) It shows the specified toolbar. |
3 | view.hideToolbar() It hides the specified toolbar in the view. |
4 | view.showToolbar() It shows the specified toolbar in the view. |
Example
The following example demonstrates the use of toolbar layout in the Framework7.
First, we will create one HTML page called toolbar.html as shown below −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Toolbar Layout</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "center sliding">Toolbar Layout</div> </div> </div> <div class = "pages navbar-through"> <div data-page = "index" class = "page with-subnavbar"> <div class = "page-content"> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Suspendisse at nibh felis. Nunc consequat diam et tellus tempor gravida. Donec hendrerit aliquet risus, ut tempor purus dictum sit amet. Aenean sagittis interdum leo in molestie. Aliquam sodales in diam eu consectetur. Sed posuere a orci id imperdiet.</p> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Suspendisse at nibh felis. Nunc consequat diam et tellus tempor gravida. Donec hendrerit aliquet risus, ut tempor purus dictum sit amet. Aenean sagittis interdum leo in molestie. Aliquam sodales in diam eu consectetur. Sed posuere a orci id imperdiet.</p> </div> </div> </div> <div class = "toolbar"> <div class = "toolbar-inner"> <a href = "#" class = "link">Link 1</a> <a href = "#" class = "link">Link 2</a> <a href = "#" class = "link">Link 3</a> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> // here initialize the app var myApp = new Framework7(); // If your using custom DOM library, then save it to $$ variable var $$ = Dom7; // Add the view var mainView = myApp.addView('.view-main', { // enable the dynamic navbar for this view dynamicNavbar: true }); </script> </body> </html>
Now, initialize your app and views in the custom JS file toolbar.js.
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as toolbar.html file in your server root folder.
Open this HTML file as http://localhost/toolbar.html and the output is displayed as shown below.
Framework7 - Search Bar
Description
Framework 7 allows searching the elements by using the searchbar class.
Search Bar Parameters
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | searchList It searches the CSS selector or HTML element of the list. |
string or HTML Element | - |
2 | searchIn You can search the list view elements of CSS selectors, also searches the elements by passing .item-title, .item-text classes. |
string | '.item-title' |
3 | found It searches the CSS selector or HTML element using "found" element. Further, it uses .searchbar-found element if there is no element specified. |
string or HTML Element | - |
4 | notfound It searches the CSS selector or HTML element using "not-found" element. Further, it uses .searchbar-not-found element if there is no element specified. |
string or HTML Element | - |
5 | overlay It searches the CSS selector or HTML element using "searchbar overlay" element and uses .searchbar-overlay element, if there is no element specified. |
string or HTML Element | - |
6 | ignore You can ignore the CSS selector for items by using the searchbar. |
string | '.searchbar-ignore' |
7 | customSearch When it is enabled, the searchbar will not search through any of list blocks which is specified by searchList and you will be allowed to use custom search functionality. |
boolean | false |
8 | removeDiacritics When searching for an element, remove the diacritics by enabling this parameter. |
boolean | false |
9 | hideDividers This parameter will hide item dividers and group title, if there are no items. |
boolean | true |
10 | hideGroups This parameter will hide the groups, if there are no items found in the list view groups. |
boolean | true |
Search Bar Callbacks
S.No | Callbacks & Description | Type | Default |
---|---|---|---|
1 | onSearch This method will fire callback function while doing search. |
function (s) | - |
2 | onEnable This method will fire callback function when Search Bar becomes active. |
function (s) | - |
3 | onDisable This method will fire callback function when Search Bar becomes inactive. |
function (s) | - |
4 | onClear This method will fire callback function when you click on the "clear" element. |
function (s) | - |
Search Bar Properties
S.No | Properties & Description |
---|---|
1 | mySearchbar.params Represents the initialized parameters passed with object. |
2 | mySearchbar.query Searches the current query. |
3 | mySearchbar.searchList Defines the search list block. |
4 | mySearchbar.container Defines the search bar container with HTML element. |
5 | mySearchbar.input Defines the search bar input with HTML element. |
6 | mySearchbar.active It defines whether search bar is enabled or disabled. |
Search Bar Methods
S.No | Methods & Description |
---|---|
1 | mySearchbar.search(query); This method searches the passed query. |
2 | mySearchbar.enable(); It enables the search bar. |
3 | mySearchbar.disable(); It disables the search bar. |
4 | mySearchbar.clear(); You can clear the query and search results. |
5 | mySearchbar.destroy(); It destroys the search bar instance. |
Search Bar JavaScript Events
S.No | Event & Description | Target |
---|---|---|
1 | search You can fire this event while searching elements. |
<div class="list-block"> |
2 | clearSearch This event will get fired when user clicks on the clearSearch element. |
<div class="list-block"> |
3 | enableSearch When Search Bar becomes enable, this event will get fired. |
<div class="list-block"> |
4 | disableSearch When Search Bar gets disabled, and user clicks on cancel button, or "search bar-overlay" element, this event will get fired. |
<div class="list-block"> |
Example
The following example demonstrates the use of search bar on scroll in the Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Search Bar Layout</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages navbar-fixed"> <div data-page = "home" class = "page"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "center sliding">Search Bar Layout</div> </div> </div> <form data-search-list = ".list-block-search" data-search-in = ".item-title" class = "searchbar searchbar-init"> <div class = "searchbar-input"> <input type = "search" placeholder = "Search"><a href = "#" class = "searchbar-clear"></a> </div> <a href = "#" class = "searchbar-cancel">Cancel</a> </form> <div class = "searchbar-overlay"></div> <div class = "page-content"> <div class = "content-block searchbar-not-found"> <div class = "content-block-inner">No element found...</div> </div> <div class = "list-block list-block-search searchbar-found"> <ul> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">India</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Argentina</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Belgium</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Brazil</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Canada</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Colombia</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Denmark</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Ecuador</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">France</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Germany</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Greece</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Haiti</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Hong Kong</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Iceland</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Ireland</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Jamaica</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Japan</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Kenya</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Kuwait</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Libya</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Liberia</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Malaysia</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Mauritius</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Mexico</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Namibia</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">New Zealand</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Oman</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Paraguay</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Philippines</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Russia</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Singapore</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">South Africa</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Thailand</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">United Kingdom</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Vatican City</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Zimbabwe</div> </div> </li> </ul> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var mainView = myApp.addView('.view-main'); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code search_bar.html file in your server root folder.
Open this HTML file as http://localhost/search_bar.html and the output is displayed as shown below.
If the element contained in the list is entered in the search bar, it displays that particular element from the list.
If the element other than the elements contained in the list is entered, it displays no element found.
Framework7 - Status Bar
Description
The iOS 7+ allows you to build full screen apps which could create an issue when your status bar overlaps your app. Framework7 solves this problem by detecting whether your app is in full screen mode or not. If your app is in full screen mode then, the Framework7 will automatically adds with-statusbar-overlay class to <html> (or removes if app is not in full screen mode) and you need to add statusbar-overlay class in <body> as shown in the following code −
<html class = "with-statusbar-overlay"> ... <body> <div class = "statusbar-overlay"></div> ...
By default, <div> will always be hidden and fixed on top of your screen. It will only be visible when the app is in full screen mode and with-statusbar-overlay class is added to <html>.
Example
The following example demonstrates the use of status bar in the Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>My App</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "statusbar-overlay"></div> <div class = "panel-overlay"></div> <div class = "panel panel-right panel-reveal"> <div class = "content-block"> <p>Contents goes here...</p> </div> </div> <div class = "views"> <div class = "view view-main"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "center sliding">My App</div> <div class = "right"> <a href = "#" class = "link icon-only open-panel"><i class = "icon icon-bars"></i></a> </div> </div> </div> <div class = "pages navbar-through toolbar-through"> <div data-page = "index" class = "page"> <div class = "page-content"> <p>This is simple application...</p> <p>page contents goes here!!!</p> </div> </div> </div> <div class = "toolbar"> <div class = "toolbar-inner"> <a href = "#" class = "link">First Link</a> <a href = "#" class = "link">Second Link</a> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> // here initialize the app var myApp = new Framework7(); // If your using custom DOM library, then save it to $$ variable var $$ = Dom7; // Add the view var mainView = myApp.addView('.view-main', { // enable the dynamic navbar for this view: dynamicNavbar: true }); //use the 'pageInit' event handler for all pages $$(document).on('pageInit', function (e) { //get page data from event data var page = e.detail.page; }) </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given html code as status_bar.html file in your server root folder.
Open this HTML file as http://localhost/status_bar.html and the output is displayed as shown below.
The example shows the use of the statusbar-overlay, which allows you to build full screen apps when your status bar overlaps the app.
Framework7 - Side Panels
Description
The side panel moves to the left or right side of the screen to display the content. Framework7 allows you to include up to 2 panels (right side panel and left side panel) to your app. You need to add panels in the beginning of the <body> and then choose the opening effect by applying the following listed classes −
panel-reveal − This will make the whole app's content move out.
panel-cover − This will make the panel to overlay on app's content.
For instance, the following code shows how to use the above classes −
<body> <!-- First add Panel's overlay which will overlays app while panel is opened --> <div class = "panel-overlay"></div> <!-- Left panel --> <div class = "panel panel-left panel-cover"> panel's content </div> <!-- Right panel --> <div class = "panel panel-right panel-reveal"> panel's content </div> </body>
The following table shows the panel types supported by Framework77 −
S.No | Type & Description |
---|---|
1 | Open and Close Panels
Once you add panel and effects, we need to add functionality to open and close the panels. |
2 | Panel Events
To detect how a user interacts with the panel, you can use panel events. |
3 | Open Panels With Swipe
Framework7 provides you the feature to open panel with swipe gesture. |
4 | Panel is Opened?
We can determine whether panel is opened or not by using the with-panel[position]-[effect] rule. |
Framework7 - Content Block
Description
Content blocks can be used to add extra content with different format.
Example
The following example demonstrates the use of content block in Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Content Block</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> </div> <div class = "center">Content Block</div> <div class = "right"> </div> </div> </div> <div class = "page-content"> <p>This is out side of content block!!!</p> <div class = "content-block"> <p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo.</p> </div> <div class = "content-block"> <div class = "content-block-inner">This is another text block wrapped with "content-block-inner"</div> </div> <div class = "content-block-title">Content Block Title</div> <div class = "content-block"> <p>Praesent nec imperdiet diam. Maecenas vel lectus porttitor, consectetur magna nec, viverra sem. Aliquam sed risus dolor. Morbi tincidunt ut libero id sodales. Integer blandit varius nisi quis consectetur.</p> </div> <div class = "content-block-title">This is another long content block title</div> <div class = "content-block"> <div class = "content-block-inner"> <p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo.</p> </div> </div> <div class = "content-block-title">Content Block Inset</div> <div class = "content-block inset"> <div class = "content-block-inner"> <p>Donec et nulla auctor massa pharetra adipiscing ut sit amet sem. Suspendisse molestie velit vitae mattis tincidunt. Ut sit amet quam mollis, vulputate turpis vel, sagittis felis. </p> </div> </div> <div class = "content-block-title">Content Block Tablet Inset</div> <div class = "content-block tablet-inset"> <div class = "content-block-inner"> <p>Donec et nulla auctor massa pharetra adipiscing ut sit amet sem. Suspendisse molestie velit vitae mattis tincidunt. Ut sit amet quam mollis, vulputate turpis vel, sagittis felis. </p> </div> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var mainView = myApp.addView('.view-main'); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as content_block.html file in your server root folder.
Open this HTML file as http://localhost/content_block.html and the output is displayed as shown below.
The code adds extra formatting and required spacing for the text content.
Framework7 - Layout Grid
Description
Framework7 provides different types of grid types to place the content as per user need.
The layout grid provides different types of columns size as described in the following table −
S.No | Class | Table Class | Width |
---|---|---|---|
1 | col-5 | tablet-5 | 5% |
2 | col-10 | tablet-10 | 10% |
3 | col-15 | tablet-15 | 15% |
4 | col-20 | tablet-20 | 20% |
5 | col-25 | tablet-25 | 25% |
6 | col-30 | tablet-30 | 30% |
7 | col-33 | tablet-33 | 33.3% |
8 | col-35 | tablet-35 | 35% |
9 | col-40 | tablet-40 | 40% |
10 | col-45 | tablet-45 | 45% |
11 | col-50 | tablet-50 | 50% |
12 | col-55 | tablet-55 | 55% |
13 | col-60 | tablet-60 | 60% |
14 | col-65 | tablet-65 | 65% |
15 | col-66 | tablet-66 | 66.6% |
16 | col-70 | tablet-70 | 70% |
17 | col-75 | tablet-75 | 75% |
18 | col-80 | tablet-80 | 80% |
19 | col-85 | tablet-85 | 85% |
20 | col-90 | tablet-90 | 90% |
21 | col-95 | tablet-95 | 95% |
21 | col-100 | tablet-100 | 100% |
22 | col-auto | tablet-auto | Equal width |
Example
The following example provides the grid layout for placing your content as you need in Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Layout Grid</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> <style> div[class* = "col-"] { background: #fff; text-align: center; color: #000; border: 1px solid #D8D8D8; } .row { margin-bottom: 10px; } </style> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "center sliding">Layout Grid</div> </div> </div> <div class = "pages"> <div data-page = "index" class = "page navbar-fixed"> <div class = "page-content"> <div class = "content-block-title">Columns with gutter</div> <div class = "content-block"> <div class = "row"> <div class = "col-50">col 1</div> <div class = "col-50">col 2</div> </div> <div class = "row"> <div class = "col-25">col 1</div> <div class = "col-25">col 2</div> <div class = "col-25">col 3</div> <div class = "col-25">col 4</div> </div> <div class = "row"> <div class = "col-33">col 1</div> <div class = "col-33">col 2</div> <div class = "col-33">col 3</div> </div> <div class = "content-block-title">Columns without gutter</div> <div class = "content-block"> <div class = "row no-gutter"> <div class = "col-50">col 1</div> <div class = "col-50">col 2</div> </div> <div class = "row no-gutter"> <div class = "col-25">col 1</div> <div class = "col-25">col 2</div> <div class = "col-25">col 3</div> <div class = "col-25">col 4</div> </div> <div class = "row no-gutter"> <div class = "col-33">col 1</div> <div class = "col-33">col 2</div> <div class = "col-33">col 3</div> </div> </div> <div class = "content-block-title">Nested Columns</div> <div class = "content-block"> <div class = "row"> <div class = "col-50"> col 1 <div class = "row"> <div class = "col-40">col 2</div> <div class = "col-60">col 3</div> </div> </div> <div class = "col-50"> col 1 <div class = "row"> <div class = "col-75">col 2</div> <div class = "col-25">col 3</div> </div> </div> </div> </div> <div class = "content-block-title">Columns With Different Equal Width</div> <div class = "content-block"> <div class = "row"> <div class = "col-100 tablet-50">col 1</div> <div class = "col-100 tablet-50">col 2</div> </div> <div class = "row"> <div class = "col-50 tablet-25">col 1</div> <div class = "col-50 tablet-25">col 2</div> <div class = "col-50 tablet-25">col 3</div> <div class = "col-50 tablet-25">col 4</div> </div> <div class = "row"> <div class = "col-100 tablet-40">col 1</div> <div class = "col-50 tablet-60">col 2</div> <div class = "col-50 tablet-60">col 3</div> <div class = "col-100 tablet-40">col 4</div> </div> </div> <div class = "content-block-title">Columns With Equal Width</div> <div class = "content-block"> <div class = "row"> <div class = "col-auto">col-1</div> <div class = "col-auto">col-2</div> <div class = "col-auto">col-3</div> <div class = "col-auto">col-4</div> </div> <div class = "row no-gutter"> <div class = "col-auto">col-1</div> <div class = "col-auto">col-2</div> <div class = "col-auto">col-3</div> <div class = "col-auto">col-4</div> </div> </div> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var mainView = myApp.addView('.view-main'); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as layout_grid.html file in your server root folder.
Open this HTML file as http://localhost/layout_grid.html the output is displayed as shown below.
This code provides different types of grid types to place the content as per the user need.
Framework7 - Overlays
Description
Framework7 provides different types of overlays to work with the applications smoothly. The following table lists some of the Framework7 overlays −
S.No | Overlay Type & Description |
---|---|
1 | Modal
Modal is a small window that display the content from separate sources without leaving the parent window. |
2 | Popup
Popup is a popup box which displays the content when the user clicks on the element. |
3 | Popover
To manage the presentation of temporary content, the popover component can be used. |
4 | Action Sheet
The Action Sheet is used to present the user with a set of possibilities for how to handle a given task. |
5 | Login Screen
Overlay login screen is used for displaying the login screen format which can be used in page or popup or as a standalone overlay. |
6 | Picker Modal
Picker modal is used to pick some custom content which is similar to the calender picker. |
Framework7 - Preloaders
Description
Preloader in Framework7 is made with Scalable Vector Graphic (SVG) and animated with CSS, which makes it easily resizable. Preloader is available in two colors −
- the default is light background
- another one is dark background
You can use the preloader class in your HTML as shown below −
Example
The following example demonstrates the use of preloader in the Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Panel Events</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> </div> <div class = "center">Framework7 Preloader</div> <div class = "right"> </div> </div> </div> <div class = "page-content"> <div class = "content-block row"> <div class = "col-25"><span class = "preloader"></span><br>Default Preloader</div> <div class = "col-25 col-dark"><span class = "preloader preloader-white"></span><br>White Preloader</div> <div class = "col-25"><span style = "width:42px; height:42px" class = "preloader"></span><br>Big Preloader</div> <div class = "col-25 col-dark"><span style = "width:42px; height:42px" class = "preloader preloader-white"></span><br>White Preloader</div> </div> </div> </div> </div> </div> </div> <style>.col-25{padding:5px;text-align:center;}.col-dark{background:#222;}</style> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as preloader.html file in your server root folder.
Open this HTML file as http://localhost/preloader.html and the output is displayed as shown below.
This code displays the preloader indicator, which is made with SVG and animated with CSS.
Framework7 - Progress Bar
Description
The progress bars can be used to show loading of assets or progress of a task to the users. You can specify the progress bar by using the progressbar class. When the user does not know how long the loading process will be there for the request, you can use progressbar-infinite class.
Progress Bar JavaScript API
The progress bar can be used along with the JavaScript API to specify show, hide and progress properties by using the following methods −
S.No | Methods | Description & Parameters |
---|---|---|
1 | myApp.setProgressbar (container , progress, speed) | It sets the progress bar for the progress of a task.
|
2 | myApp.hideProgressbar (contain er) | It hides the progress bar.
|
3 | myApp.showProgressbar (contai ner, progress, color) | It displays the progress bar.
|
Example
The following example displays an animated determinate and indeterminate progress bars to indicate activity in Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Progress Bar</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.material.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.material.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "center">Progress Bar</div> </div> </div> <div class = "page-content"> <div class = "content-block-title">Determinate Progress Bar</div> <div class = "content-block"> <div class = "content-block-inner"> <p>Inline determinate progress bar:</p> <div class = "progressbar-inline"> <p><span data-progress = "10" class = "progressbar"></span></p> <p class = "buttons-row"> <a href = "#" data-progress = "25" class = "button button-raised">25%</a> <a href = "#" data-progress = "50" class = "button button-raised">50%</a> <a href = "#" data-progress = "75" class = "button button-raised">75%</a> <a href = "#" data-progress = "100" class = "button button-raised">100%</a> </p> </div> <p>Loads and hides the determinate progress bar:</p> <div class = "progressbar-load-hide"> <p><a href = "#" class = "button button-raised">Start Loading</a></p> </div> <p>Displays the determinate progress bar on top:</p> <p class = "progressbar-overlay"><a href = "#" class = "button button-raised">Start Loading</a></p> </div> </div> <div class = "content-block-title">Infinite Progress Bar</div> <div class = "content-block"> <div class = "content-block-inner"> <p>Inline infinite progress bar:</p> <p><span class = "progressbar-infinite"></span></p> <p>Displays the infinite progress bar in multiple colors:</p> <p><span class = "progressbar-infinite color-multi"></span></p> <p>Displays the infinite progress bar on top:</p> <p class = "progressbar-infinite-overlay"><a href = "#" class = "button button-raised">Start Loading</a></p> <p>Displays the infinite progress bar in multiple colors on top:</p> <p class = "progressbar-infinite-multi-overlay"><a href = "#" class = "button button-raised">Start Loading</a></p> </div> </div> <div class = "content-block-title">Different types of colored progress bars:</div> <div class = "list-block"> <ul> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "5" class = "progressbar color-red"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "10" class = "progressbar color-pink"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "20" class = "progressbar color-deeppurple"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "30" class = "progressbar color-blue"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "40" class = "progressbar color-cyan"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "50" class = "progressbar color-green"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "60" class = "progressbar color-lime"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "70" class = "progressbar color-amber"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "80" class = "progressbar color-deeporange"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "90" class = "progressbar color-gray"></div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div data-progress = "100" class = "progressbar color-black"></div> </div> </li> </ul> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7({ material: true }); var $$ = Dom7; $$('.progressbar-inline .button').on('click', function () { var progress = $$(this).attr('data-progress'); var progressbar = $$('.progressbar-inline .progressbar'); myApp.setProgressbar(progressbar, progress); }); $$('.progressbar-load-hide .button').on('click', function () { var container = $$('.progressbar-load-hide p:first-child'); //it doesn't load if another progresbar is loading if (container.children('.progressbar').length) return; myApp.showProgressbar(container, 0); var progress = 0; function simulateLoading() { setTimeout(function () { var progressBefore = progress; progress += Math.random() * 20; myApp.setProgressbar(container, progress); if (progressBefore < 100) { simulateLoading(); } else myApp.hideProgressbar(container); }, Math.random() * 200 + 200); } simulateLoading(); }); $$('.progressbar-overlay .button').on('click', function () { var container = $$('body'); if (container.children('.progressbar, .progressbar-infinite').length) return; myApp.showProgressbar(container, 0, 'orange'); var progress = 0; function simulateLoading() { setTimeout(function () { var progressBefore = progress; progress += Math.random() * 20; myApp.setProgressbar(container, progress); if (progressBefore < 100) { simulateLoading(); } //hides the progressbar else myApp.hideProgressbar(container); }, Math.random() * 200 + 200); } simulateLoading(); }); $$('.progressbar-infinite-overlay .button').on('click', function () { var container = $$('body'); if (container.children('.progressbar, .progressbar-infinite').length) return; myApp.showProgressbar(container, 'yellow'); setTimeout(function () { myApp.hideProgressbar(); }, 3000); }); $$('.progressbar-infinite-multi-overlay .button').on('click', function () { var container = $$('body'); if (container.children('.progressbar, .progressbar-infinite').length) return; myApp.showProgressbar(container, 'multi'); setTimeout(function () { myApp.hideProgressbar(); }, 3000); }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as progress_bar.html file in your server root folder.
Open this HTML file as http://localhost/progress_bar.html and the output is displayed as shown below.
The example displays the progress bar, which indicates how long an operation will take to complete the process and displays the different types of progress bars to indicate activity.
Framework7 - List Views
Description
List views are powerful UI components that present data in a scrollable list of multiple rows. The Framework7 provides different types of List View to work with your application. The following table lists some of the Framework7 List View −
S.No | Types & Description |
---|---|
1 | List View
List views are powerful user interface components, which present data in a scrollable list containing multiple rows. |
2 | Contacts List
The contacts list is a type of list view, which can be used to display the list of people contacts. |
3 | Media List View
The Media list view is used to display complicated data structures like products, services, users information. |
4 | Swipeout
The swipeout allows you to reveal hidden menu actions by swiping over the list elements. |
5 | Sortable List
The sortable list is a type of list view, which sorts the list view elements. |
6 | Virtual List
Virtual list a type of list view, which includes lists of large number of data elements without dropping their performance. |
Framework7 - Accordion
Description
The accordion is a graphical control element displayed as a stacked list of items. Each accordion can be expanded or stretched to reveal the content associated with that accordion.
Collapsible Layout
When you are using single separate collapsible element, you need to omit the accordion-list wrapper element.
Following is a structure of collapsible layout −
<!-- Single collapsible element ------> <div class = "accordion-item"> <div class = "accordion-item-toggle"></div> <div class = "accordion-item-content"></div> </div> <!-- Separate collapsible element --> <div class = "accordion-item"> <div class = "accordion-item-toggle"></div> <div class = "accordion-item-content"></div> </div>
The following classes are used for accordion in Framework7 −
S.No | Classes & Description |
---|---|
1 | accordion-list It is an optional class, which contains a group of accordion items list. |
2 | accordion-item It is a required class for single accordion item. |
3 | accordion-item-toggle It is a required class used to expand accordion item content. |
4 | accordion-item-content It is a required class used for hidden accordion item content. |
5 | accordion-item-expanded It is a single expanded accordion item. |
Accordion JavaScript API
JavaScript API methods are used to open and close the accordion programmatically.
It contains the following JavaScript API methods −
myApp.accordionOpen(item) − Used to open accordion.
myApp.accordionClose(item) − Used to close accordion.
myApp.accordionToggle(item) − Used to toggle accordion.
All methods contains parameter called item which is HTML or string element of accordion-item.
Accordion Events
Accordion contains four events as listed in the following table −
S.No | Event | Target & Description |
---|---|---|
1 | open | Accordion item When you open an animation, this event will get fired. |
2 | opened | Accordion item When the opening of an animation is completed, this event will get fired. |
3 | close | Accordion item When you close an animation, this event will get fired. |
4 | closed | Accordion item When the closing of an animation is completed, this event will get fired. |
Accordion List View
In accordion list view, you can use item-link element instead of accordion-toggle.
<div class = "list-block accordion-list"> <ul> <li class = "accordion-item"> <a href = "" class = "item-link item-content"> <div class = "item-inner"> <div class = "item-title">1st Item</div> </div> </a> <div class = "accordion-item-content">Content for 1st Item...</div> </li> <li class = "accordion-item"> <a href = "" class = "item-link item-content"> <div class = "item-inner"> <div class = "item-title">2nd Item</div> </div> </a> <div class = "accordion-item-content">Content for 2nd Item...</div> </li> </ul> </div>
Example
The following example demonstrates the use of accordion in the Framework7 −
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, minimum-scale=1, user-scalable=no, minimal-ui"> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <title>Accordion</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css"> </head> <body> <div class="views"> <div class="view view-main"> <div class="pages"> <div data-page="home" class="page navbar-fixed"> <div class="navbar"> <div class="navbar-inner"> <div class="left"> </div> <div class="center">Accordion</div> <div class="right"> </div> </div> </div> <div class="page-content"> <div class="content-block-title">List of Programming Lagauges</div> <div class="list-block accordion-list"> <ul> <li class="accordion-item"> <a href="#" class="item-content item-link"> <div class="item-inner"> <div class="item-title">C</div> </div> </a> <div class="accordion-item-content"> <div class="content-block"> <p>C is a general-purpose, procedural, imperative computer programming language developed in 1972 by Dennis M. Ritchie at the Bell Telephone Laboratories to develop the UNIX operating system. C is the most widely used computer language. It keeps fluctuating at number one scale of popularity along with Java programming language, which is also equally popular and most widely used among modern software programmers.</p> </div> </div> </li> <li class="accordion-item"> <a href="#" class="item-content item-link"> <div class="item-inner"> <div class="item-title">C++</div> </div> </a> <div class="accordion-item-content"> <div class="content-block"> <p>C++ is a middle-level programming language developed by Bjarne Stroustrup starting in 1979 at Bell Labs. C++ runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX. This reference will take you through simple and practical approach while learning C++ Programming language. </p> </div> </div> </li> <li class="accordion-item"> <a href="#" class="item-content item-link"> <div class="item-inner"> <div class="item-title">Java</div> </div> </a> <div class="accordion-item-content"> <div class="content-block"> <p>Java is a high-level programming language originally developed by Sun Microsystems and released in 1995. Java runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX. This tutorial gives a complete understanding of Java. This reference will take you through simple and practical approach while learning Java Programming language. </p> </div> </div> </li> </ul> </div> <div class="content-block-title">Separate Collapsibles</div> <div class="list-block"> <ul> <li class="accordion-item"> <a href="#" class="item-content item-link"> <div class="item-inner"> <div class="item-title">C</div> </div> </a> <div class="accordion-item-content"> <div class="content-block"> <p >C is a general-purpose, procedural, imperative computer programming language developed in 1972 by Dennis M. Ritchie at the Bell Telephone Laboratories to develop the UNIX operating system. C is the most widely used computer language. It keeps fluctuating at number one scale of popularity along with Java programming language, which is also equally popular and most widely used among modern software programmers.</p> </div> </div> </li> <li class="accordion-item"> <a href="#" class="item-content item-link"> <div class="item-inner"> <div class="item-title">C++</div> </div> </a> <div class="accordion-item-content"> <div class="content-block"> <p>C++ is a middle-level programming language developed by Bjarne Stroustrup starting in 1979 at Bell Labs. C++ runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX. This reference will take you through simple and practical approach while learning C++ Programming language. </p> </div> </div> </li> <li class="accordion-item"> <a href="#" class="item-content item-link"> <div class="item-inner"> <div class="item-title">Java</div> </div> </a> <div class="accordion-item-content"> <div class="content-block"> <p>Java is a high-level programming language originally developed by Sun Microsystems and released in 1995. Java runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX. This tutorial gives a complete understanding of Java. This reference will take you through simple and practical approach while learning Java Programming language. </p> </div> </div> </li> </ul> </div> <div class="content-block-title">Custom Accordion</div> <div class="content-block accordion-list custom-accordion"> <div class="accordion-item"> <div class="accordion-item-toggle"><i class="icon icon-plus">+</i><i class="icon icon-minus">-</i><span>C</span></div> <div class="accordion-item-content"> <p>C is a general-purpose, procedural, imperative computer programming language developed in 1972 by Dennis M. Ritchie at the Bell Telephone Laboratories to develop the UNIX operating system. C is the most widely used computer language. It keeps fluctuating at number one scale of popularity along with Java programming language, which is also equally popular and most widely used among modern software programmers.</p> </div> </div> <div class="accordion-item"> <div class="accordion-item-toggle"><i class="icon icon-plus">+</i><i class="icon icon-minus">-</i><span>C++</span></div> <div class="accordion-item-content"> <p>C++ is a middle-level programming language developed by Bjarne Stroustrup starting in 1979 at Bell Labs. C++ runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX. This reference will take you through simple and practical approach while learning C++ Programming language. </p> </div> </div> <div class="accordion-item"> <div class="accordion-item-toggle"><i class="icon icon-plus">+</i><i class="icon icon-minus">-</i><span>Java</span></div> <div class="accordion-item-content"> <p>Java is a high-level programming language originally developed by Sun Microsystems and released in 1995. Java runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX. This tutorial gives a complete understanding of Java. This reference will take you through simple and practical approach while learning Java Programming language. </p> </div> </div> </div> </div> </div> </div> </div> </div> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <style>.custom-accordion{padding-left:0;padding-right:0;}.custom-accordion .accordion-item-toggle{padding:0px 15px;height:44px;line-height:44px;font-size:17px;color:#000;border-bottom:1px solid rgba(0,0,0,0.15);cursor:pointer;}.custom-accordion .accordion-item-toggle:active{background:rgba(0,0,0,0.15);}.custom-accordion .accordion-item-toggle span{display:inline-block;margin-left:15px;}.custom-accordion .accordion-item:last-child .accordion-item-toggle{border-bottom:none;}.custom-accordion .icon-plus,.custom-accordion .icon-minus{display:inline-block;width:22px;height:22px;border:1px solid #000;border-radius:100%;line-height:20px;text-align:center;}.custom-accordion .icon-minus{display:none;}.custom-accordion .accordion-item-expanded .icon-minus{display:inline-block;}.custom-accordion .accordion-item-expanded .icon-plus{display:none;}.custom-accordion .accordion-item-content{padding:0px 15px;}</style> <script> // here initialize the app var myApp = new Framework7(); // If your using custom DOM library, then save it to $$ variable var $$ = Dom7; // Add the view var mainView = myApp.addView('.view-main', { // enable the dynamic navbar for this view: dynamicNavbar: true }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as accordion.html file in your server root folder.
Open this HTML file as http://localhost/accordion.html and the output is displayed as shown below.
The example provides collapsible layout, which can be expanded to display the content associated with the accordion.
Framework7 - Cards
Description
Cards contain organized information related to a single subject like a photo, link, and text. The following table shows Framework7 card types −
S.No | Types & Description |
---|---|
1 | Card HTML Layout
The basic card HTML layout uses card classes to arrange its items. |
2 | List View With Cards
You can use cards as list view elements by adding cards-list class to <div class="list-block">. |
Framework7 - Chips
Description
Chip is a small block of entity, which can contain a photo, small string of title, and short information.
Chips HTML Layout
The following code shows the basic chip HTML layout used in Framework7 −
<div class = "chip"> <div class = "chip-media"> <img src = "http://lorempixel.com/100/100/people/9/"> </div> <div class = "chip-label">Jane Doe</div> <a href = "#" class = "chip-delete"></a> </div>
The above HTML layout contains many classes as listed below −
chips − It is the chip container.
chip-media − This is the chip media element that can contain images, avatar or icon. It is optional.
card-label − It is the chip text label.
card-delete − It is the optional delete icon link of a chip.
Example
The following example represents the entities such as albums, card elements etc. along with a photo and brief information −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Chips HTML Layout</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.material.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.material.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div class = "page navbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "center">Chips HTML Layout</div> </div> </div> <div class = "page-content"> <div class = "content-block-title">Chips With Text</div> <div class = "content-block"> <div class = "chip"> <div class = "chip-label">Chip one</div> </div> <div class = "chip"> <div class = "chip-label">Chip two</div> </div> </div> <div class = "content-block-title">Chips with icons</div> <div class = "content-block"> <div class = "chip"> <div class = "chip-media bg-blue"><i class = "icon icon-form-calendar"></i></div> <div class = "chip-label">Set Date</div> </div> <div class = "chip"> <div class = "chip-media bg-purple"><i class = "icon icon-form-email"></i></div> <div class = "chip-label">Sent Mail</div> </div> </div> <div class = "content-block-title">Contact Chips</div> <div class = "content-block"> <div class = "chip"> <div class = "chip-media"><img src = "/framework7/images/pic.jpg"></div> <div class = "chip-label">James Willsmith</div> </div> <div class = "chip"> <div class = "chip-media"><img src = "/framework7/images/pic2.jpg"></div> <div class = "chip-label">Sunil Narayan</div> </div> <div class = "chip"> <div class = "chip-media bg-pink">R</div> <div class = "chip-label">Raghav</div> </div> <div class = "chip"> <div class = "chip-media bg-teal">S</div> <div class = "chip-label">Sharma</div> </div> <div class = "chip"> <div class = "chip-media bg-red">Z</div> <div class = "chip-label">Zien</div> </div> </div> <div class = "content-block-title">Deletable Chips</div> <div class = "content-block"> <div class = "chip"> <div class = "chip-label">Chip one</div> <a href = "#" class = "chip-delete"></a> </div> <div class = "chip"> <div class = "chip-media bg-teal">S</div> <div class = "chip-label">Sharma</div> <a href = "#" class = "chip-delete"></a> </div> <div class = "chip"> <div class = "chip-media bg-purple"><i class = "icon icon-form-email"></i></div> <div class = "chip-label">Sent</div> <a href = "#" class = "chip-delete"></a> </div> <div class = "chip"> <div class = "chip-media"><img src = "/framework7/images/pic.jpg"></div> <div class = "chip-label">James Willsmith</div> <a href = "#" class = "chip-delete"></a> </div> <div class = "chip"> <div class = "chip-label">Chip two</div> <a href = "#" class = "chip-delete"></a> </div> <div class = "chip"> <div class = "chip-media bg-green">R</div> <div class = "chip-label">Raghav</div> <a href = "#" class = "chip-delete"></a> </div> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <style> </style> <script> var myApp = new Framework7 ({ material: true }); var $$ = Dom7; $$('.chip-delete').on('click', function (e) { e.preventDefault(); var chip = $$(this).parents('.chip'); myApp.confirm('Do you want to delete this Chip?', function () { chip.remove(); }); }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as cards_html_layout.html file in your server root folder.
Open this HTML file as http://localhost/cards_html_layout.html and the output is displayed as shown below.
The example represents the complex entities in small blocks such as albums, card elements, posted image, which contains photo, title string and brief information.
Framework7 - Buttons
Description
Framework7 provides a group of ready to use buttons by just adding appropriate classes to links or input buttons.
S.No | Types & Description |
---|---|
1 | iOS Theme Buttons
Framework7 provides many iOS theme buttons, which can be used by applying appropriate classes. |
2 | Material Theme Buttons
The material theme provides many buttons to use in your application by using appropriate classes. |
Framework7 - Action Button
Description
Framework7 provides floating action button for a promoted action. They appear as a floating circled icon over the UI. It has the motion behaviors that contain morphing, launching, and transferring an anchor point.
Floating action button is only supported in Material theme.
The following table lists the action buttons types used in Framework7 −
S.No | Types & Description |
---|---|
1 | Floating Action Button Layout
It is very simple; you just need to place it as a child of page or view. |
2 | Morph To Popover
If you want to open popover on clicking the floating action button then you can use floating-button-to-popover class. |
3 | Speed Dial
You can invoke related actions upon clicking the floating action button by using speed dial. |
Framework7 - Forms
Description
Forms are used for interacting with the users and collects data from the web users using text fields, checkboxes, radio buttons etc.
Framework7 provides different types of form elements to work with the applications smoothly as specified in the table below −
S.No | Overlay Type & Description |
---|---|
1 | Form Elements
Form elements are used to make good looking form layouts. |
2 | Checkboxes and Radios
The list view extention allows you to create checkbox and radio inputs in Framework7. |
3 | Smart Select
Smart select is an easy way of changing form selects to dynamic pages by using groups of checkboxes and radio inputs. |
4 | Disabled Elements
The elements can be disabled by applying the disabled class to the element or adding disabled attributes for form element. |
5 | Form Data
Framework7 has very few useful methods, which makes working with forms easy. |
6 | Form Storage
It is easy with the form storage to store and parse forms data automatically on Ajax loaded pages. |
7 | Ajax Form Submit
Framework7 allows you to send data automatically using Ajax. |
Framework7 - Tabs
Description
Tabs are sets of logically grouped content that allow us to quickly flip between them to and saves the space like accordions.
Tabs Layout
The following code defines the layout for the tabs −
<!-- Tabs wrapper, shoud have "tabs" class.It is a required element --> <div class = "tabs"> <!-- The tab, should have "tab" class and unique id attribute --> <!-- The tab is active by default - "active" class --> <div class = "tab active" id = "tab1"> ... The content for Tab 1 goes here ... </div> <!-- The second tab, should have "tab" class and unique id attribute --> <div class = "tab" id = "tab2"> ... The content for Tab 2 goes here ... </div> </div>
Where −
<div class = "tabs"> − It is a required wrapper for all tabs. If we miss this, tabs will not work at all.
-
<div class = "tab"> − It is a single tab, which should have unique id attribute.
-
<div class = "tab active"> − It is a single active tab, which uses additional active class to make tab visible (active).
Switching Between Tabs
You can use some controller in tabs layout, so that the user can switch between them.
For this, you need to create links (<a> tags) with tab-link class and href attribute equal to the id attribute of target tab −
<!-- Here the link is used to activates 1st tab, has the same href attribute (#tab1) as the id attribute of 1st tab (tab1) --> <a href = "#tab1" class = "tab-link active">Tab 1</a> <!-- Here the link is used to activates 2st tab, has the same href attribute (#tab2) as the id attribute of 2st tab (tab2) --> <a href = "#tab2" class = "tab-link">Tab 2</a> <a href = "#tab3" class = "tab-link">Tab 3</a>
Switch Multiple Tabs
If you are using single tab link to switch between multiple tabs, then you can use classes instead of using ID's and data-tab attribute.
<!-- For Top Tabs --> <div class = "tabs tabs-top"> <div class = "tab tab1 active">...</div> <div class = "tab tab2">...</div> <div class = "tab tab3">...</div> </div> <!-- For Bottom Tabs --> <div class = "tabs tabs-bottom"> <div class = "tab tab1 active">...</div> <div class = "tab tab2">...</div> <div class = "tab tab3">...</div> </div> <!-- For Tabs links --> <div class = "tab-links"> <!-- Links are switch top and bottom tabs to .tab1 --> <a href = "#" class = "tab-link" data-tab = ".tab1">Tab 1</a> <!-- Links are switch top and bottom tabs to .tab2 --> <a href = "#" class = "tab-link" data-tab = ".tab2">Tab 2</a> <!-- Links are switch top and bottom tabs to .tab3 --> <a href = "#" class = "tab-link" data-tab = ".tab3">Tab 3</a> </div>
The data-tab attribute of tab-link contains CSS selector of target tab/tabs.
We can use different ways of tabs, these are specified in the following table −
S.No | Tabs Types & Description |
---|---|
1 | Inline Tabs
Inline tabs are sets of grouped in inline that allow you to quickly flip between them. |
2 | Switch Tabs From Navbar
We can place tabs in navigation bar that allow you to flip between them. |
3 | Switch Views From Tab Bar
Single tab can be used to switch between views with its own navigation and layout. |
4 | Animated Tabs
You can use simple transition (animation) to switch tabs. |
5 | Swipeable Tabs
You can create swipeable tabs with simple transition by using the tabs-swipeable-wrap class for the tabs. |
6 | Tabs JavaScript Events
JavaScript events can be used when you are working with JavaScript code for the tabs. |
7 | Show Tab Using JavaScript
You can switch or show the tab using JavaScript methods. |
Framework7 - Swiper Slider
Description
The swiper slider is the most powerful and modern touch slider and comes into Framework7 with lots of features.
The following HTML layout shows the swiper slider −
<!-- Container class for slider --> <div class = "swiper-container"> <!-- Wrapper class for slider --> <div class = "swiper-wrapper"> <!-- Slides --> <div class = "swiper-slide">Slide 1</div> <div class = "swiper-slide">Slide 2</div> <div class = "swiper-slide">Slide 3</div> ... other slides ... </div> <!-- Define pagination, if it is required --> <div class = "swiper-pagination"></div> </div>
The following classes are used for swiper slider −
swiper-container − It is a required element for main slider container and it is used for slides and paginations.
Swiper-wrapper − It is a required element for additional wrapper slides.
swiper-slide − It is a single slide element and it could contain any HTML inside.
swiper-pagination − It is optional for pagination bullets and those are created automatically.
You can initialize the swiper with JavaScript using its related methods −
myApp.swiper(swiperContainer,parameters)
OR
new Swiper(swiperContainer, parameters)
Both the methods are used to initialize the slider with options.
The above given methods contain the following parameters −
swiperContainer − It is HTMLElement or string of a swiper container and it is required.
parameters − These are optional elements containing object with swiper parameters.
For example −
var mySwiper = app.swiper('.swiper-container', { speed: 400, spaceBetween: 100 });
It is possible to access a swiper's instance and the swiper property of the slider's container has the following HTML element −
var mySwiper = $$('.swiper-container')[0].swiper; // Here you can use all slider methods like: mySwiper.slideNext();
You can see the different ways and types of swiper in the following table −
S.No | Swiper Types & Description |
---|---|
1 | Default Swiper With Pagination
It is a modern touch slider and the swiper swipes horizontally, by default. |
2 | Vertical Swiper
This one also works as a default swiper but it swipes vertically. |
3 | With Space Between Slides
This swiper is used to give space between two slides. |
4 | Multiple Swipers
This swiper uses more than one swipers on a single page. |
5 | Nested Swipers
It is the combination of vertical and horizontal slides. |
6 | Custom Controls
It is used for custom controls to choose or swipe any slide. |
7 | Lazy Loading
It can be used for multimedia file, which takes time to load. |
Framework7 - Photo Browser
Description
The Photo browser is similar to iOS photo browser component to display group of images, which can be zoomed and panned. To slide between images, photo browser uses Swiper Slider.
The following table shows the photo browser types used in framework7 −
S.No | Photo browser types & Description |
---|---|
1 | Create Photo Browser Instance
Photo browser can be created and initialized using JavaScript only. |
2 | Photo Browser Parameters
Framework7 provides a list of parameters, which are used with photo browser. |
3 | Photo Browser Methods & Properties
You will get an initialized instance variable to use photo browser methods and properties once you initialize photo browser. |
4 | Photos Array
During initializing photo browser, you need to pass array with photos/videos in photos parameter. |
5 | Photo Browser Templates
Framework7 provides you many photo browser templates, which you can pass on photo browser initialization. |
Framework7 - Autocomplete
Description
Autocomplete is a Framework7's mobile friendly and touch optimized component, which can be as dropdown or in standalone way. You can create and initialize Autocomplete instance by using the JavaScript method −
myApp.autocomplete(parameters)
Where parameters are required objects used to initialize the Autocomplete instance.
Autocomplete Parameters
The following table lists the available Autocomplete parameters in Framework7 −
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | openIn It defines how to open an Autocomplete which could be used as dropdown, popup or page. |
string | page |
2 | source It uses autocomplete instance, search query and renders function to pass matched items with array. |
function (autocomplete, query, render) | - |
3 | valueProperty It specifies the item value of matched item object's key. |
string | id |
4 | limit It displays the limited number of items in autocomplete per query. |
number | - |
5 | preloader Preloader can be used to specify autocomplete layout by setting it to true. |
boolean | false |
6 | preloaderColor It specifies the preloader color. By default, the color is "black". |
string | - |
7 | value Defines the array with default selected values. |
array | - |
8 | textProperty It specifies the item value of matched item object's key, which can be used as a title of displayed options. |
string | text |
Standalone Autocomplete Parameters
The following table lists the available Standalone Autocomplete parameters in Framework7 −
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | opener It is string or HTML element parameter, which will open the standalone autocomplete page. |
string or HTMLElement | - |
2 | popupCloseText It is used to close the autocomplete popup. |
string | 'Close' |
3 | backText It provides the back link when autocomplete is opened as page. |
string | 'Back' |
4 | pageTitle It specifies the autocomplete page title. |
string | - |
5 | searchbarPlaceholderText It specifies the search bar placeholder text. |
string | 'Search' |
6 | searchbarCancelText It defines the search bar cancel button text. |
string | 'cancel' |
7 | notFoundText It displays the text when there is no matched element found. |
string | 'Nothing found' |
8 | multiple It allows to select multiple selection by setting it to true. |
boolean | false |
9 | navbarTheme It specifies the color theme for navbar. |
string | - |
10 | backOnSelect When the user picks value, the autocomplete will be closed by setting it to true. |
boolean | false |
11 | formTheme It specifies the color theme for form. |
string | - |
Dropdown Autocomplete Parameters
The following table lists the available Dropdown Autocomplete parameters in Framework7 −
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | input It is string or HTML element used for text input. |
string or HTMLElement | - |
2 | dropdownPlaceholderText It specifies the dropdown placeholder text. |
string | - |
3 | updateInputValueOnSelect You can update the input value on select by setting it to true. |
boolean | true |
4 | expandInput You can expand the text input in List View to make full screen wide during dropdown visible by setting item-input it to true. |
boolean | false |
Autocomplete Callbacks Functions
The below table lists available Dropdown Autocomplete parameters in Framework7 −
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | onChange Whenever the autocomplete value is changed, this callback function will be executed. |
function (autocomplete, value) | - |
2 | onOpen Whenever autocomplete is opened, this callback function will be executed. |
function (autocomplete) | - |
3 | onClose Whenever autocomplete is closed, this callback function will be executed. |
function (autocomplete) | - |
Autocomplete Templates
The following table lists the available Dropdown Autocomplete parameters in Framework7 −
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | navbarTemplate It is standalone autocomplete navbar template. |
string | - |
2 | itemTemplate It is standalone template7 form item. |
string | - |
3 | dropdownTemplate It is template7 dropdown template. |
string | - |
4 | dropdownItemTemplate It is template7 dropdown list item. |
string | - |
5 | dropdownPlaceholderTemplate It is template7 dropdown placeholder item. |
string | - |
Default templates
Following are the default template code snippets for the above defined templates parameters −
navbarTemplate
<div class = "navbar {{#if navbarTheme}}theme-{{navbarTheme}}{{/if}}"> <div class = "navbar-inner"> <div class = "left sliding"> {{#if material}} <a href = "#" class = "link {{#if inPopup}}close-popup{{else}}back{{/if}} icon-only"> <i class = "icon icon-back"></i> </a> {{else}} <a href = "#" class = "link {{#if inPopup}}close-popup{{else}}back{{/if}}"> <i class = "icon icon-back"></i> {{#if inPopup}} <span>{{popupCloseText}}</span> {{else}} <span>{{backText}}</span> {{/if}} </a> {{/if}} </div> <div class = "center sliding">{{pageTitle}}</div> {{#if preloader}} <div class = "right"> <div class = "autocomplete-preloader preloader {{#if preloaderColor}} preloader-{{preloaderColor}} {{/if}}"> </div> </div> {{/if}} </div> </div>
itemTemplate
<li> <label class = "label-{{inputType}} item-content"> <input type = "{{inputType}}" name = "{{inputName}}" value = "{{value}}" {{#if selected}}checked{{/if}}> {{#if material}} <div class = "item-media"> <i class = "icon icon-form-{{inputType}}"></i> </div> <div class = "item-inner"> <div class = "item-title">{{text}}</div> </div> {{else}} {{#if checkbox}} <div class = "item-media"> <i class = "icon icon-form-checkbox"></i> </div> {{/if}} <div class = "item-inner"> <div class = "item-title">{{text}}</div> </div> {{/if}} </label> </li>
dropdownTemplate
<div class = "autocomplete-dropdown"> <div class = "autocomplete-dropdown-inner"> <div class = "list-block"> <ul></ul> </div> </div> {{#if preloader}} <div class = "autocomplete-preloader preloader {{#if preloaderColor}} preloader-{{preloaderColor}} {{/if}}"> {{#if material}} {{materialPreloaderHtml}} {{/if}} </div> {{/if}} </div>
dropdownItemTemplate
<li> <label class = "{{#unless placeholder}}label-radio{{/unless}} item-content" data-value = "{{value}}"> <div class = "item-inner"> <div class = "item-title">{{text}}</div> </div> </label> </li>
dropdownPlaceholderTemplate
<li class = "autocomplete-dropdown-placeholder"> <div class = "item-content"> <div class = "item-inner"> <div class = "item-title">{{text}}</div> </div> </label> </li>
Autocomplete Methods
The following table specifies Autocomplete methods available in Framework7 −
S.No | Methods & Description | 1 | myAutocomplete.params Defines the initialization parameters that are passes with object. |
---|---|
2 | myAutocomplete.value It defines the array with selected values. |
3 | myAutocomplete.opened It opens the Autocomplete if it is set to true. |
4 | myAutocomplete.dropdown It specifies an instance of Autocomplete dropdown. |
5 | myAutocomplete.popup It specifies an instance of Autocomplete popup. |
6 | myAutocomplete.page It specifies an instance of Autocomplete page. |
7 | myAutocomplete.pageData It defines Autocomplete page data. |
8 | myAutocomplete.searchbar It defines Autocomplete searchbar instance. |
Autocomplete Properties
The following table specifies Autocomplete methods available in Framework7 −
S.No | Properties & Description |
---|---|
1 | myAutocomplete.open() It opens the Autocomplete, which could be used as dropdown, popup or page. |
2 | myAutocomplete.close() It closes the Autocomplete. |
3 | myAutocomplete.showPreloader() It shows the Autocomplete preloader. |
4 | myAutocomplete.hidePreloader() It hides the Autocomplete preloader. |
5 | myAutocomplete.destroy() It ruins the Autocomplete preloader instance and removes all events. |
Example
The following example demonstrates the use of autocomplete parameters hiding in the Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Autocomplete</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> </div> <div class = "center">Autcomplete</div> <div class = "right"> </div> </div> </div> <div class = "page-content"> <div class = "content-block-title">Simple Dropdown Autocomplete</div> <div class = "list-block"> <ul> <li class = "item-content"> <div class = "item-title label">Country</div> <div class = "item-input"> <input type = "text" placeholder = "Country" id = "autocomplete-dropdown"> </div> </li> </ul> </div> <div class = "content-block-title">Dropdown With Input Expand</div> <div class = "list-block"> <ul> <li class = "item-content"> <div class = "item-title label">Country</div> <div class = "item-input"> <input type = "text" placeholder = "Country" id = "autocomplete-dropdown-expand"> </div> </li> </ul> </div> <div class = "content-block-title">Dropdown With All Values</div> <div class = "list-block"> <ul> <li class = "item-content"> <div class = "item-title label">Country</div> <div class = "item-input"> <input type = "text" placeholder = "Country" id = "autocomplete-dropdown-all"> </div> </li> </ul> </div> <div class = "content-block-title">Dropdown With Placeholder</div> <div class = "list-block"> <ul> <li class = "item-content"> <div class = "item-title label">Country</div> <div class = "item-input"> <input type = "text" placeholder = "Country" id = "autocomplete-dropdown-placeholder"> </div> </li> </ul> </div> <div class = "content-block-title">Simple Standalone Autocomplete</div> <div class = "list-block"> <ul> <li> <a href = "#" id = "autocomplete-standalone" class = "item-link item-content autocomplete-opener"> <input type = "hidden"> <div class = "item-inner"> <div class = "item-title">Favorite Country</div> <div class = "item-after"></div> </div> </a> </li> </ul> </div> <div class = "content-block-title">Popup Standalone Autocomplete</div> <div class = "list-block"> <ul> <li> <a href = "#" id = "autocomplete-standalone-popup" class = "item-link item-content autocomplete-opener"> <input type = "hidden"> <div class = "item-inner"> <div class = "item-title">Favorite Country</div> <div class = "item-after"></div> </div> </a> </li> </ul> </div> <div class = "content-block-title">Multiple Values Standalone Autocomplete</div> <div class = "list-block"> <ul> <li> <a href = "#" id = "autocomplete-standalone-multiple" class = "item-link item-content autocomplete-opener"> <input type = "hidden"> <div class = "item-inner"> <div class = "item-title">Favorite Countries</div> <div class = "item-after"></div> </div> </a> </li> </ul> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var $$ = Dom7; var mainView = myApp.addView('.view-main'); // Countries data array var countries = ('India Africa Australia NewZealand England WestIndies Scotland Zimbabwe Srilanka Bangladesh').split(' '); // Simple Dropdown var autocompleteDropdownSimple = myApp.autocomplete ({ input: '#autocomplete-dropdown', openIn: 'dropdown', source: function (autocomplete, query, render) { var results = []; if (query.length === 0) { render(results); return; } // You can find matched items for (var i = 0; i < countries.length; i++) { if (countries[i].toLowerCase().indexOf(query.toLowerCase()) >= 0) results.push(countries[i]); } // Display the items by passing array with result items render(results); } }); // Dropdown with input expand var autocompleteDropdownExpand = myApp.autocomplete ({ input: '#autocomplete-dropdown-expand', openIn: 'dropdown', expandInput: true, // expandInput used as item-input in List View will be expanded to full screen wide //during dropdown source: function (autocomplete, query, render) { var results = []; if (query.length === 0) { render(results); return; } // Find matched items for (var i = 0; i < countries.length; i++) { if (countries[i].toLowerCase().indexOf(query.toLowerCase()) >= 0) results.push(countries[i]); } // Display the items by passing array with result items render(results); } }); // Dropdown with all values var autocompleteDropdownAll = myApp.autocomplete ({ input: '#autocomplete-dropdown-all', openIn: 'dropdown', source: function (autocomplete, query, render) { var results = []; // You can find matched items for (var i = 0; i < countries.length; i++) { if (countries[i].toLowerCase().indexOf(query.toLowerCase()) >= 0) results.push(countries[i]); } // Display the items by passing array with result items render(results); } }); // Dropdown with placeholder var autocompleteDropdownPlaceholder = myApp.autocomplete ({ input: '#autocomplete-dropdown-placeholder', openIn: 'dropdown', dropdownPlaceholderText: 'Type as "India"', source: function (autocomplete, query, render) { var results = []; if (query.length === 0) { render(results); return; } // You can find matched items for (var i = 0; i < countries.length; i++) { if (countries[i].toLowerCase().indexOf(query.toLowerCase()) >= 0) results.push(countries[i]); } // Display the items by passing array with result items render(results); } }); // Simple Standalone var autocompleteStandaloneSimple = myApp.autocomplete ({ openIn: 'page', //open in page opener: $$('#autocomplete-standalone'), //link that opens autocomplete backOnSelect: true, //go back after we select something source: function (autocomplete, query, render) { var results = []; if (query.length === 0) { render(results); return; } // You can find matched items for (var i = 0; i < countries.length; i++) { if (countries[i].toLowerCase().indexOf(query.toLowerCase()) >= 0) results.push(countries[i]); } // Display the items by passing array with result items render(results); }, onChange: function (autocomplete, value) { // Here add the item text value to item-after $$('#autocomplete-standalone').find('.item-after').text(value[0]); // You can add item value to input value $$('#autocomplete-standalone').find('input').val(value[0]); } }); // Standalone Popup var autocompleteStandalonePopup = myApp.autocomplete ({ openIn: 'popup', // Opens the Autocomplete in page opener: $$('#autocomplete-standalone-popup'), // It will open standalone autocomplete popup backOnSelect: true, //After selecting item, then go back to page source: function (autocomplete, query, render) { var results = []; if (query.length === 0) { render(results); return; } // You can find matched items for (var i = 0; i < countries.length; i++) { if (countries[i].toLowerCase().indexOf(query.toLowerCase()) >= 0) results.push(countries[i]); } // Display the items by passing array with result items render(results); }, onChange: function (autocomplete, value) { // Here add the item text value to item-after $$('#autocomplete-standalone-popup').find('.item-after').text(value[0]); // You can add item value to input value $$('#autocomplete-standalone-popup').find('input').val(value[0]); } }); // Multiple Standalone var autocompleteStandaloneMultiple = myApp.autocomplete ({ openIn: 'page', //Opens the Autocomplete in page opener: $$('#autocomplete-standalone-multiple'), //link that opens autocomplete multiple: true, //Allow multiple values source: function (autocomplete, query, render) { var results = []; if (query.length === 0) { render(results); return; } // You can find matched items for (var i = 0; i < countries.length; i++) { if (countries[i].toLowerCase().indexOf(query.toLowerCase()) >= 0) results.push(countries[i]); } // Display the items by passing array with result items render(results); }, onChange: function (autocomplete, value) { // Here add the item text value to item-after $$('#autocomplete-standalone-multiple').find('.item-after').text(value.join(', ')); // You can add item value to input value $$('#autocomplete-standalone-multiple').find('input').val(value.join(', ')); } }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as autocomplete.html file in your server root folder.
Open this HTML file as http://localhost/autocomplete.html and the output is displayed as shown below.
The example provides autocomplete of values in simple dropdown, dropdown with all values, dropdown with placeholder, standalone autocomplete etc.
Framework7 - Picker
Description
Picker looks like iOS native picker and it is a powerful component that allows you to pick any values from list and also used to create custom overlay pickers. You can use Picker as inline component or as overlay. The overlay picker will be automatically converted to Popover on tablets (iPad).
Using the following App’s method, you can create and initialize the JavaScript method −
myApp.picker(parameters)
Where parameters are required objects, used to initialized the picker instance and it is a required method.
Picker Parameters
The following table specifies the available parameters in the pickers −
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | container String with CSS selector or HTMLElement used to generate Picker HTML for an inline pickers. |
string or HTMLElement | - |
2 | input The related input element placed with the string with CSS selector or HTMLElement. |
string or HTMLElement | - |
3 | scrollToInput It is used to scroll viewport (page-content) of input, whenever picker is opened. |
boolean | true |
4 | inputReadOnly Used to set the "readonly" attribute on specified input. |
boolean | true |
5 | convertToPopover It is used to convert the picker modal to Popover on large screens like iPad. |
boolean | true |
6 | onlyOnPopover You can open the picker in Popover by enabling it. |
boolean | true |
7 | cssClass To picker modal, you can use additional CSS class name. |
string | - |
8 | closeByOutsideClick You can close the picker by clicking outside of picker or input element by enabling the method. |
boolean | false |
9 | toolbar It is used to enable the picker modal toolbar. |
boolean | true |
10 | toolbarCloseText Used for Done/Close toolbar button. |
string | 'Done' |
11 | toolbarTemplate It is toolbar HTML Template, by default it is HTML string with the following template − <div class = "toolbar"> <div class = "toolbar-inner"> <div class = "left"></div> <div class = "right"> <a href = "#" class = "link close-picker"> {{closeText}} </a> </div> </div> </div> |
string | - |
Specific Picker Parameters
The following table lists the available specific picker parameters −
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | rotateEffect It enables 3D rotate effect in picker. |
boolean | false |
2 | momentumRatio When you release picker after fast touch and move, it produces more momentum. |
number | 7 |
3 | updateValuesOnMomentum Used to update pickers and input values during momentum. |
boolean | false |
4 | updateValuesOnTouchmove Used to update pickers and input values during touch move. |
boolean | true | 5 | value The array has its initial values and also each array item represents the value of related column. |
array | - |
6 | formatValue The function is used to format the input value, and it should return new/formatted string value. The values and displayValues are array of related column. |
function (p, values, displayValues) | - |
7 | cols Every array item represents an object with column parameters. |
array | - |
Picker Parameter Callbacks
The following table shows the lists of callback functions available in pickers −
S.No | Callbacks & Description | Type | Default |
---|---|---|---|
1 | onChange The callback function will be executed whenever the picker value changed and the values and displayValues are arrays of related column. |
function (p, values, displayValues) | - |
2 | onOpen The callback function will be executed whenever picker is opened. |
function (p) | - |
3 | onClose The callback function will be executed whenever picker is closed. |
function (p) | - |
Column Parameters
At the moment of configuration of Picker, we need to pass cols parameter. It is a represented as array, where each item is object with column parameters −
S.No | Parameters & Description | Type | Default |
---|---|---|---|
1 | values You can specify the string columns values with an array. |
array | - |
2 | displayValues It has array with string columns values and it will display value from values parameter, When it not specified. |
array | - |
3 | cssClass The CSS class name used to set on column HTML container. |
string | - |
4 | textAlign It is used to set text alignment of column values and it could be "left", "center" or "right". |
string | - | 5 | width Width is calculated automatically, by default. If you need to fix column widths in picker with dependent columns that should be in px. |
number | - |
6 | divider It is used for column that should be visual divider, it doesn't have any values. |
boolean | false |
7 | content It is used to specified divider-column (divider:true) with content of the column. |
string | - |
Column Callbacks Parameter
S.No | Callbacks & Description | Type | Default |
---|---|---|---|
1 | onChange Whenever the column value will change at that time callback function will execute. The value and displayValue represent current column value and displayValue. |
function (p, value, displayValue) | - |
Picker Properties
The Picker variable has many properties those are given in the following table −
S.No | Properties & Description |
---|---|
1 | myPicker.params You can initialize passed parameters with object. |
2 | myPicker.value The selected value for each column is represented by an array of item. |
3 | myPicker.displayValue The selected display value for each column is represented by an array of item. |
4 | myPicker.opened When picker is opened, it sets to true value. |
5 | myPicker.inline When picker is inline, it sets to true value. |
6 | myPicker.cols The Picker columns has its own methods and properties. |
7 | myPicker.container The Dom7 instance is used for HTML container. |
Picker Methods
The picker variable has helpful methods, which are given in the following table −
S.No | Methods & Description |
---|---|
1 | myPicker.setValue(values, duration) Use to set new picker value. The values are in array where each item represents value for each column. duration - It is transition duration in ms. |
2 | myPicker.open() Use to open Picker. |
3 | myPicker.close() Use to close Picker. |
4 | myPicker.destroy() Use to destroy Picker instance and remove all events. |
Column Properties
Each column in myPicker.cols array also has its own useful properties, which are given in the following table −
//Get first column var col = myPicker.cols[0];
S.No | Properties & Description |
---|---|
1 | col.container You can create an instance with column HTML container. |
2 | col.items You can create an instance with column items HTML elements. |
3 | col.value Used to select the current column value. |
4 | col.displayValue Used to select the current column value of display. |
5 | col.activeIndex Give the current index number, which is selected/active item. |
Column Methods
The useful column methods are given in the following table −
S.No | Methods & Description |
---|---|
1 | col.setValue(value, duration) Used to set a new value for the current column. The value must be a new value, and duration is transition duration given in ms. |
2 | col.replaceValues(values, displayValues) Used to replace the column values and displayValues with new ones. |
It is used to access Picker's instance from its HTML container, whenever you initialize Picker as inline Picker.
var myPicker = $$('.picker-inline')[0].f7Picker;
There are different types of Pickers as specified in the following table −
S.No | Tabs Types & Description |
---|---|
1 | Picker With Single Value
It is a powerful component that allows you to pick any values from list. |
2 | Two Values and 3D-Rotate Effect
In the picker you can use for 3D rotate effect. |
3 | Dependent Values
Values are dependent on each other for specified element. |
4 | Custom Toolbar
You can use one or more picker on single page for customize. |
5 | Inline Picker / Date-time
You can select number of values in the inline picker.Like date has date, month, year and time has hours, minutes, seconds. |
Framework7 - Calendar
Description
The Calendar component allows you to handle dates easily and can be used as an inline or as an overlay component. The overlay calendar will be converted to popover on tablets automatically.
Calendar can be created and initialized only by using JavaScript. You need to use the related App's method as shown below −
myApp.calendar(parameters) − This method returns initialized calendar instance. It accepts an object as paramaters.
The following table shows the calendar usage in Framework7 −
S.No | Calendar Usage & Description |
---|---|
1 | Calendar Parameters
Framework7 provides a list of parameters, which are used with calendar. |
2 | Calendar Methods & Properties
You will get an initialized instance variable to use the calendar methods and properties, once you initialize the calendar. |
3 | Access to Calendar's Instance
It is possible to access the calendar instance from its HTML container when you initialize the calendar as inline. |
Framework7 - Refresh
Description
It is a special component used to refresh (reload) the page contents by pulling it.
The following code shows how to refresh the page content −
<div class = "page"> <!-- Page content should have additional "pull-to-refresh-content" class --> <div class = "page-content pull-to-refresh-content" data-ptr-distance = "55"> <!-- Default pull to refresh layer--> <div class = "pull-to-refresh-layer"> <div class = "preloader"></div> <div class = "pull-to-refresh-arrow"></div> </div> <!-- usual content below --> <div class = "list-block"> ... </div> </div>
The following classes are used in refresh −
page-content − It has an additional pull-to-refresh-content class and its required to enable pull to refresh.
pull-to-refresh-layer − It is a hidden layer, which is used to pull to refresh visual element and it is just a preloader and an arrow.
data-ptr-distance = "55" − This is additional attribute that allows you to set custom 'pull to refresh' trigger distance and its default value is 44px.
Pull To Refresh Events
In ‘Pull to Refresh’ there are some JavaScript events, which are given in the following table −
S.No | Event & Description | Target |
---|---|---|
1 | pullstart It will be triggered whenever you start pulling to refresh content. |
Pull To Refresh content. <div class = "pull-to-refresh-content"> |
2 | pullmove It is triggered when you are pulling to refresh content. |
Pull To Refresh content. <div class="pull-to-refresh-content"> |
3 | pullend It will be triggered whenever you release pull to refresh content. |
Pull To Refresh content. <div class="pull-to-refresh-content"> |
4 | refresh This event will be triggered when the pull to refresh enters in the "refreshing" state. |
Pull To Refresh content. <div class="pull-to-refresh-content"> |
5 | refreshdone It will be triggered after it is refreshed and it goes back to the initial state. This will be done after calling pullToRefreshDone method. |
Pull To Refresh content. <div class="pull-to-refresh-content"> |
There are App's methods that can be used with Pull to Refresh.
S.No | App's Methods & Description |
---|---|
1 | myApp.pullToRefreshDone(ptrContent) It is used to reset pull-to-refresh content. |
2 | myApp.pullToRefreshTrigger(ptrContent) It is used to trigger to refresh on specified pull to refresh content. |
3 | myApp.destroyPullToRefresh(ptrContent) It is used to destroy/disable pull to refresh on specified pull to refresh content. |
4 | myApp.initPullToRefresh(ptrContent) It is used to initialize/enable pull to refresh content. |
Where ptrContent is used to HTMLElement or string to pull-to-refresh-content to reset/trigger or disable/enable.
Example
The following example demonstrates the use of refresh component that initiates the refreshing of a page contents −
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, minimum-scale=1, user-scalable=no, minimal-ui"> <meta name="apple-mobile-web-app-capable" content="yes"> <meta name="apple-mobile-web-app-status-bar-style" content="black"> <title>Pull To Refresh</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css"> </head> <div class="views"> <div class="view view-main"> <div class="pages"> <div data-page="home" class="page navbar-fixed"> <div class="navbar"> <div class="navbar-inner"> <div class="left"> </div> <div class="center">Pull To Refresh</div> <div class="right"> </div> </div> </div> <div class="page-content pull-to-refresh-content"> <div class="pull-to-refresh-layer"> <div class="preloader"></div> <div class="pull-to-refresh-arrow"></div> </div> <div class="list-block media-list"> <ul> <li class="item-content"> <div class="item-media"><img src="/framework7/images/apple.png" width="44"></div> <div class="item-inner"> <div class="item-title-row"> <div class="item-title">apple</div> </div> </div> </li> <li class="item-content"> <div class="item-media"><img src="/framework7/images/froots_img.jpg" width="44"></div> <div class="item-inner"> <div class="item-title-row"> <div class="item-title">strawberry</div> </div> </div> </li> <li class="item-content"> <div class="item-media"><img src="/framework7/images/mango.jpg" width="44"></div> <div class="item-inner"> <div class="item-title-row"> <div class="item-title">Mango</div> </div> </div> </li> </ul> <div class="list-block-label"> <p>Just pull page down to let the magic happen.</p> </div> </div> </div> </div> </div> </div> </div> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var $$ = Dom7; // Dummy Content var fruits = ['mango', 'orange', 'watermelon', 'banana']; // Pull to refresh content var ptrContent = $$('.pull-to-refresh-content'); // Add 'refresh' listener on it ptrContent.on('refresh', function (e) { // Emulate 2s loading setTimeout(function () { var picURL = 'images/Fruit.jpg' + Math.round(Math.random() * 100); var fruit = fruits[Math.floor(Math.random() * fruits.length)]; var itemHTML = '<li class="item-content">' + '<div class="item-media"><img src="/framework7/images/Fruit.jpg" width="44"/></div>' + '<div class="item-inner">' + '<div class="item-title-row">' + '<div class="item-title">' + fruit + '</div>' + '</div>' + '</div>' + '</li>'; // Prepend new list element ptrContent.find('ul').prepend(itemHTML); // When loading done, we need to reset it myApp.pullToRefreshDone(); }, 2000); }); </script> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as pull_to_refresh.html file in your server root folder.
Open this HTML file as http://localhost/pull_to_refresh.html and the output is displayed as shown below.
When the user pulls down, the page will be refreshed with the contents.
Framework7 - Infinite Scroll
Description
The Infinite Scroll allows you to load additional content and performs the required actions when the page is near to the bottom.
The following HTML layout shows the infinite scroll −
<div class = "page"> <div class = "page-content infinite-scroll" data-distance = "100"> ... </div> </div>
The above layout contains the following classes −
page-content infinite-scroll − It is used for infinite scroll container.
data-distance − This attribute allows you to configure distance from the bottom of page (in px) to trigger infinite scroll event and its default value is 50px.
If you want to use infinite scroll on top the page, you need to add additional "infinite-scroll-top" class to "page-content" −
<div class = "page"> <div class = "page-content infinite-scroll infinite-scroll-top"> ... </div> </div>
Infinite Scroll Events
infinite − It is used to trigger when page scroll reaches specified distance to the bottom. It will be target by div class = "page-content infinite-scroll".
There are two App's methods that can be used with infinite scroll container −
To add infinite scroll event listener to the specified HTML container, use the following method −
myApp.attachInfiniteScroll(container)
You can remove the infinite scroll event listener from the specified HTML container by using the following method −
myApp.detachInfiniteScroll(container)
Where parameters are required options used as HTMLElement or string for infinite scroll container.
Example
The following example demonstrates the infinite scroll that loads the additional content when the page scroll is near to the bottom −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name="apple-mobile-web-app-capable" content="yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>infinite_scroll</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> </div> <div class = "center sliding">Infinite Scroll</div> <div class = "right"> </div> </div> </div> <div class = "pages navbar-through"> <div data-page = "home" class = "page"> <div class = "page-content infinite-scroll"> <div class = "list-block"> <ul> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 1</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 2</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 3</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 4</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 5</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 6</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 7</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 8</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 9</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 10</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 11</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 12</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 13</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 14</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 15</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 16</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 17</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 18</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 19</div> </div> </li> <li class = "item-content"> <div class = "item-inner"> <div class = "item-title">Item 20</div> </div> </li> </ul> </div> <div class = "infinite-scroll-preloader"> <div class = "preloader"></div> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <style> .infinite-scroll-preloader { margin-top:-20px; margin-bottom:10px; text-align:center; } .infinite-scroll-preloader .preloader { width:34px; height:34px; } </style> <script> var myApp = new Framework7(); var $$ = Dom7; // Loading flag var loading = false; // Last loaded index var lastIndex = $$('.list-block li').length; // Max items to load var maxItems = 60; // Append items per load var itemsPerLoad = 20; // Attach 'infinite' event handler $$('.infinite-scroll').on('infinite', function () { // Exit, if loading in progress if (loading) return; // Set loading flag loading = true; // Emulate 1s loading setTimeout(function () { // Reset loading flag loading = false; if (lastIndex >= maxItems) { // Nothing more to load, detach infinite scroll events to prevent unnecessary loadings myApp.detachInfiniteScroll($$('.infinite-scroll')); // Remove preloader $$('.infinite-scroll-preloader').remove(); return; } // Generate new items HTML var html = ''; for (var i = lastIndex + 1; i <= lastIndex + itemsPerLoad; i++) { html += '<li class = "item-content"> <div class = "item-inner"> <div class = "item-title"> Item ' + i + ' </div> </div> </li>'; } // Append new items $$('.list-block ul').append(html); // Update last loaded index lastIndex = $$('.list-block li').length; }, 1000); }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as infinite_scroll.html file in your server root folder.
Open this HTML file as http://localhost/infinite_scroll.html and the output is displayed as shown below.
The example allows loading of additional content when your page scroll reaches specified distance to the bottom.
Framework7 - Messages
Description
Messages are component of Framework7, which gives visualization of comments and messaging system in the application.
Messages Layout
The framework7 has the following messages layout structure −
<div class = "page"> <div class = "page-content messages-content"> <div class = "messages"> <!-- Defines the date stamp --> <div class = "messages-date">Monday, Apr 26 <span>10:30</span></div> <!-- Displays the sent message and by default, it will be in green color on right side --> <div class = "message message-sent"> <!-- Define the text with bubble type --> <div class = "message-text">Hello</div> </div> <!-- Displays the another sent message --> <div class = "message message-sent"> <!-- Define the text with bubble type --> <div class = "message-text">How are you?</div> </div> <!-- Displays the received message and by default, it will be in grey color on left side --> <div class = "message message-with-avatar message-received"> <!-- Provides sender name --> <div class = "message-name">Smith</div> <!-- Define the text with bubble type --> <div class = "message-text">I am fine, thanks</div> <!-- Defines the another date stamp --> <div class = "messages-date">Tuesday, April 28 <span>11:16</span></div> </div> </div> </div>
The layout contains the following classes in different areas −
Messages Page Layout
The following table shows the classes of messages page layout with description.
S.No | Classes & Description |
---|---|
1 | messages-content It is a required additional class added to "page-content" and used for messages wrapper. |
2 | messages It is a required element for messages bubbles. |
3 | messages-date It uses date stamp container to display date and time of message sent or received. |
4 | message It is a single message to be displayed. |
Single Message Inner Parts
The following table shows the classes of simple message inner parts with description.
S.No | Classes & Description |
---|---|
1 | message-name It provides the sender name. |
2 | message-text Define the text with bubble type. |
3 | message-avatar It specifies the sender avatar. |
4 | message-label It specifies the text label below the bubble. |
Additional classes for Single Message Container
The following table shows additional classes for single message container description.
S.No | Classes & Description |
---|---|
1 | message-sent It specifies that message was sent by the user and is displayed with green background color on the right side. |
2 | message-received It is used for displaying the single message indicating that, the message was received by user and stays on the left side with grey background color. |
3 | message-pic It is an additional class for displaying image with a single message. |
4 | message-with-avatar It is an additional class for displaying a single message (received or sent) with avatar. |
5 | message-with-tail You can add a bubble tail for single message (received or sent). |
Additional Available Classes for Single Message
The following table shows the available classes for a single message with description.
S.No | Classes & Description |
---|---|
1 | message-hide-name It is an additional class for hiding message name for a single message (received or sent). |
2 | message-hide-avatar It is an additional class for hiding message avatar for a single message (received or sent). |
3 | message-hide-label It is an additional class for hiding message label for a single message (received or sent). |
4 | message-last You can use this class to indicate the last received or sent message in current conversation by one sender for a single message (received or sent). |
5 | message-first You can use this class to indicate first received or first sent message in current conversation by one sender for a single message (received or sent). |
Initializing Messages with JavaScript
You can initialize the messages with JavaScript by using the following method −
myApp.messages(messagesContainer, parameters)
The method takes two options −
messagesContainer − It is a required HTML element or string that includes messages container HTML element.
parameters − It specifies an object with messages parameters.
Messages Parameters
The following table shows the parameters of messages with description.
S.No | Parameter & Description | Type | Default |
---|---|---|---|
1 | autoLayout It adds additional required classes to each message by enabling it. |
boolean | true |
2 | newMessagesFirst You can display message on top instead of displaying on bottom by enabling it. |
boolean | false |
3 | messages It displays an array of messages in which each message should be represented as object with message parameters. |
array | - |
4 | messageTemplate It displays the single message template. |
string | - |
Messages Properties
The following table shows the properties of messages with description.
S.No | Property & Description |
---|---|
1 | myMessages.params You can initialize the passed parameters with object. |
2 | myMessages.container Defines the DOM7 element with a message bar HTML container. |
Messages Methods
The following table shows the methods of messages with description.
S.No | Method & Description |
---|---|
1 | myMessages.addMessage(messageParameters, method, animate); The message can be added to the beginning or to the end by using the method parameter. It has the following parameters −
|
2 | myMessages.appendMessage(messageParameters, animate); It adds a message to the end of message container. |
3 | myMessages.prependMessage(messageParameters, animate); It adds a message to the beginning of message container. |
4 | myMessages.addMessages(messages, method, animate); You can add multiple messages at one time. It has the following parameter −
|
5 | myMessages.removeMessage(message); It is used to remove the message. It has the following parameter −
|
6 | myMessages.removeMessages(messages); You can remove the multiple messages. It has the following parameter −
|
7 | myMessages.scrollMessages(); You can scroll messages from top to bottom and vice versa depending on the first parameter of new message. |
8 | myMessages.layout(); Auto layout can be applied to the messages. |
9 | myMessages.clean(); It is used to clean the messages. |
10 | myMessages.destroy(); It is used to destroy the messages. |
Single Message Parameters
The following table shows the parameters for a single message with description.
S.No | Parameter & Description | Type | Default |
---|---|---|---|
1 | text It defines the message text, which could be a HTML string. |
string | - |
2 | name It specifies the sender name. |
string | - |
3 | avatar It specifies the sender avatar URL string. |
string | - |
4 | time It specifies the time string of the message like '9:45 AM', '18:35'. |
string | - |
5 | type It provides type of message whether it could be sent or recieved message. |
string | sent |
6 | label It defines the label of the message. |
string | - |
7 | day It gives the day string of the message like 'sunday', 'monday', 'yesterday' etc. |
string | - |
Initializing Messages with HTML
You can initialize the messages with HTML without JavaScript by using the additional messages-init class to messages and use the data- attributes to pass the required parameters as shown in the code snippet given below −
... <div class = "page-content messages-content"> <!-- Initialize the messages using additional "messages-init" class--> <div class = "messages messages-init" data-auto-layout = "true" data-new-messages-first = "false"> ... </div> </div> ...
Example
The following example demonstrates the use of messages in the Framework7 −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Messages</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed toolbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> </div> <div class = "center">Messages</div> <div class = "right"> </div> </div> </div> <div class = "toolbar messagebar"> <div class = "toolbar-inner"> <textarea placeholder = "Message"></textarea><a href = "#" class = "link">Send</a> </div> </div> <div class = "page-content messages-content"> <div class = "messages"> <div class = "messages-date">Friday, Apr 26 <span>10:30</span></div> <div class = "message message-sent"> <div class = "message-text">Hello</div> </div> <div class = "message message-sent"> <div class = "message-text">Happy Birthday</div> </div> <div class = "message message-received"> <div class = "message-name">Smith</div> <div class = "message-text">Thank you</div> <div style = "background-image:url(/framework7/images/person.png)" class = "message-avatar"></div> </div> <div class = "messages-date">Saturday, Apr 27 <span>9:30</span></div> <div class = "message message-sent"> <div class = "message-text">Good Morning...</div> </div> <div class = "message message-sent"> <div class = "message-text"><img src = "/framework7/images/gm.jpg"></div> <div class = "message-label">Delivered</div> </div> <div class = "message message-received"> <div class = "message-name">Smith</div> <div class = "message-text">Very Good Morning...</div> <div style = "background-image:url(/framework7/images/person.png)" class = "message-avatar"></div> </div> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var $$ = Dom7; // It indicates conversation flag var conversationStarted = false; // Here initiliaze the messages var myMessages = myApp.messages('.messages', { autoLayout:true }); // Initiliaze the messagebar var myMessagebar = myApp.messagebar('.messagebar'); // Displays the text after clicking the button $$('.messagebar .link').on('click', function () { // specifies the message text var messageText = myMessagebar.value().trim(); // If there is no message, then exit from there if (messageText.length === 0) return; // Specifies the empty messagebar myMessagebar.clear() // Defines the random message type var messageType = (['sent', 'received'])[Math.round(Math.random())]; // Provides the avatar and name for the received message var avatar, name; if(messageType === 'received') { name = 'Smith'; } // It adds the message myMessages.addMessage ({ // It provides the message text text: messageText, // It displays the random message type type: messageType, // Specifies the avatar and name of the sender avatar: avatar, name: name, // Displays the day, date and time of the message day: !conversationStarted ? 'Today' : false, time: !conversationStarted ? (new Date()).getHours() + ':' + (new Date()).getMinutes() : false }) // Here you can update the conversation flag conversationStarted = true; }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as messages.html file in your server root folder.
Open this HTML file as http://localhost/messages.html and the output is displayed as shown below.
When you type a message into the message box and click the Send button, it specifies that your message has been sent and is displayed with green background color on the right side.
The message which you recieve, appears on the left side with the grey background along with the sender name.
Framework7 - Message Bar
Description
Framework7 provides special resizable toolbar to work with the messaging system in the application.
The following code shows the message bar layout −
<div clas = "toolbar messagebar"> <div clas = "toolbar-inner"> <textarea placeholder = "Message"></textarea> <a href = "#" clas = "link">Send</a> </div> </div>
In the message bar, the inside of the "page" is very important and is at the right of "messages-content" −
<div class = "page toolbar-fixed"> <!-- messagebar --> <div class = "toolbar messagebar"> <div class = "toolbar-inner"> <textarea placeholder = "Message"></textarea> <a href = "#" class = "link">Send</a> </div> </div> <!-- messages-content --> <div class = "page-content messages-content"> <div class = "messages"> ... messages </div> </div> </div>
You can use the following method for initializing the message bar with JavaScript −
myApp.messagesbar(messagesbarContainer, parameters)
The method has two options −
messagesbarContainer − It is a required HTML element or string that includes messagebar container HTML element.
parameters − It specifies an object with messagebar parameters.
For example −
var myMessagebar = app.messagebar('.messagebar', { maxHeight: 200 });
Messagebar Parameter
maxHeight − It is used to set maximum height of textarea and will be resized depending on the amount of its text. The type of parameter is number and the default value is null.
Messagebar Properties
The following table shows the messagebar properties −
S.No | Properties & Description |
---|---|
1 | myMessagebar.params You can specify object with passed initialization parameters. |
2 | myMessagebar.container You can specify dom7 element with messagebar container HTML element. |
3 | myMessagebar.textarea You can specify dom7 element with messagebar textarea HTML element. |
Messagebar Methods
The following table shows the messagebar methods −
S.No | Methods & Description |
---|---|
1 | myMessagebar.value(newValue); It sets messagebar textarea value/text and returns messagebar textarea value, if newValue is not specified. |
2 | myMessagebar.clear(); It clears the textarea and updates/resets the size. |
3 | myMessagebar.destroy(); It destroy messagebar instance. |
Initialize Messagebar with HTML
You can initialize the messagebar using HTML without JavaScript methods and properties by adding the messagebar-init class to the .messagebar and you can pass the required parameters using data- attributes.
The following code specifies the initialization of messagebar with HTML −
<div class = "toolbar messagebar messagebar-init" data-max-height = "200"> <div class = "toolbar-inner"> <textarea placeholder = "Message"></textarea> <a href = "#" class = "link">Send</a> </div> </div>
Access to Messagebar's Instance
It is possible to access the message bar instance, if you initialize it by using HTML; it is achieved by using the f7 Message bar property of its container element.
var myMessagebar = $$('.messagebar')[0].f7Messagebar; // Now you can use it myMessagebar.value('Hello world');
You can see the example of Messagebar, which is explained in this link
Framework7 - Notifications
Description
Notifications are used to show the required messages, which appear like Push (or Local) iOS notifications.
The following table demonstrates the use of notifications in details −
S.No | Notifications usage & Description |
---|---|
1 | Notifications JavaScript API
The notifications can also be added or closed with JavaScript by using the related app methods. |
2 | Notifications Layout
Framework7 notifications will be added by using JavaScript. |
3 | Example iOS
Framework7 allows you to use different types of notifications in your iOS layout. |
4 | Example Material
Framework7 notifications can also be used in material layout. |
Framework7 - Lazy Load
Description
Lazy loading delays your image loading process on a given page. Lazy loading improves scrolling performance, speeds page load and saves traffic.
Lazy load elements and images must be inside of scrollable <div class = "page-content"> to work properly.
The following table demonstrates the use of lazy load −
S.No | Lazy load usage & Description |
---|---|
1 | Usage
The lazy load can be applied to images, background images and with fade-in effect. |
2 | Init Lazy Load Manually
After initializing a page, if you add lazy load images manually then, lazy load will not work and you need to use methods to initialize it. |
It is possible to trigger image loading manually by using lazy event on lazy image/element as shown below −
$$('img.lazy').trigger('lazy'); $$('div.lazy').trigger('lazy');
Example
The following example demonstrates the use of lazy loading in Framework7 −
<!DOCTYPE html> <html class = "with-statusbar-overlay"> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Lazy Load</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> </div> <div class = "center">Lazy Load</div> <div class = "right"> </div> </div> </div> <div class = "page-content"> <div class = "content-block"> <div class = "content-block-inner"> <p> <img data-src = "/framework7/images/pic4.jpg" width = "100%" class = "lazy lazy-fadeIn"></p> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur.</p> <p> <img data-src = "/framework7/images/pic5.jpg" width = "100%" class = "lazy lazy-fadeIn"></p> <p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt.</p> <p> <img data-src = "/framework7/images/background.jpg" width = "100%" class = "lazy lazy-fadeIn"></p> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur.</p> <p> <img data-src = "/framework7/images/pic6.jpg" width = "100%" class = "lazy lazy-fadeIn"></p> <p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt.</p> <p> <img data-src = "/framework7/images/pic7.jpg" width = "100%" class = "lazy lazy-fadeIn"></p> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur.</p> <p> <img data-src = "/framework7/images/pic8.jpg" width = "100%" class = "lazy lazy-fadeIn"></p> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Praesent laoreet nisl eget neque blandit lobortis. Sed sagittis risus id vestibulum finibus. Cras vestibulum sem et massa hendrerit maximus. Vestibulum suscipit tristique iaculis. Nam vitae risus non eros auctor tincidunt quis vel nulla. Sed volutpat, libero ac blandit vehicula, est sem gravida lectus, sed imperdiet sapien risus ut neque.</p> <p><b>Using as background image:</b></p> <div data-background = "/framework7/images/pic7.jpg" style = "background: #aaa; height:60vw; background-size-cover" class = "lazy lazy-fadeIn"> </div> <p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt.</p> </div> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script>var myApp = new Framework7();</script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as framework7_lazy_load.html file in your server root folder.
Open this HTML file as http://localhost/framework7_lazy_load.html and the output is displayed as shown below.
The example specifies the lazy load of images where images will get loaded on the page when you scroll them down.
Framework7 - Color Themes
Description
Framework7 provides different color themes for your applications.
A color theme provides different types of theme colors used to work with the applications smoothly as specified in the table below −
S.No | Theme Type & Description |
---|---|
1 | iOS Theme Colors
You can use 10 different default iOS color themes for the application. |
2 | Material Theme Colors
Framework7 provides 22 different default material color themes for the application. |
Applying Color Themes
Framework7 allows you to apply color themes on different elements such as page, list-block, navbar, buttons-row etc by using the theme-[color] class to the parent element.
Example
... </body> <div class = "page theme-gray"> ... </div> <div class = "list-block theme-blue"> ... </div> <div class = "navbar theme-green"> ... </div> <div class = "buttons-row theme-red"> ... </div>
Layout Themes
You can use default layout theme for your application by using two themes white and dark. The themes can be applied by using the layout-[theme] class to the parent element.
Example
... </body> <div class = "navbar layout-white"> ... </div> <div class = "buttons-row layout-dark"> ... </div>
Helper Classes
Framework7 provides additional helper classes, which can be used outside or without themes as listed below −
color-[color] − It can be used to change text color of block or color of button, link, icon etc.
bg-[color] − It sets the predefined background color on the block or element.
border-[color] − It sets the predefined border color on the block or element.
Framework7 - Hairlines
Description
Hairline is a class that adds 1px border around the images by using the border class. With the release of 1.x, hairlines revised the working with :after and :before pseudo elements instead of using CSS borders.
Hairlines contains the following rules −
:after − This pseudo element is used for bottom and right hairlines.
:before − This pseudo element is used for top and left hairlines.
The following code snippet shows the use of :after element.
.navbar:after { background-color: red; }
The following code snippet removes the bottom hairline toolbar −
.navbar:after { display:none; } .toolbar:before { display:none; }
"no-border" class
The hairline will be removed by using no-border class and it is supported on Navbar, toolbar, card and its elements.
The following code is used to remove hairline from navbar −
<div class = "navbar no-border"> ... </div>
Framework7 - Templates Overview
Description
Template7 is a lightweight, mobile-first JavaScript engine, which represents Ajax and dynamic pages as Template7 templates with specified context and does not require any additional scripts. Template7 is associated with Framework7 as a default, lightweight template engine, which works faster for applications.
Performance
The process of compiling string to JS function is the slowest segment of template7. Hence, you do not need to compile the template multiple times, only once is sufficient.
//Here initialize the app var myApp = new Framework7(); // After initializing compile templates on app var searchTemplate = $('script#search-template').html(); var compiledSearchTemplate = Template7.compile(searchTemplate); var listTemplate = $('script#list-template').html(); var compiledListTemplate = Template7.compile(listTemplate); // Execute the compiled templates with required context using onPageInit() method myApp.onPageInit('search', function (page) { // Execute the compiled templates with required content var html = compiledSearchTemplate({/*...some data...*/}); // Do stuff with html });
Template7 is a lightweight template engine used as a standalone library without Framework7. The Template7 files can be installed using two ways −
You can download from Template7 github repository.
You can install it using the following command via Bower −
Or
bower install template7
Framework7 - Auto Compilation
Description
In Template7 you can compile your templates automatically by specifying special attributes in a <script> tag.
The following code shows the auto compilation layout −
<script type = "text/template7" id = "myTemplate"> <p>Hello, my name is {{name}} and i am {{age}} years old</p> </script>
You can use the following attributes for initializing the auto compilation −
type = "text/template7" − It is used to tell Template7 to auto compile and it is a required script type.
id = "myTemplate" − Template is accessible through the id and it is a required template id.
For automatic compilation, you need to enable app initialization by passing the following parameter −
var myApp = new Framework7 ({ //It is used to compile templates on app init in Framework7 precompileTemplates: true, });
Template7.templates / myApp.templates
The automatically compiled templates can be accessed as properties of Template7.templates after initializing the app. It is also known as myApp.templates where myApp is an initialized instance of the app.
You can use the following templates in our index.html file −
<script type = "text/template7" id = "personTemplate"> <p>Hello, my name is {{name}} and i am {{age}} years old</p> <p>I work as {{position}} at {{company}}</p> </script> <script type = "text/template7" id = "carTemplate"> <p>I have a great car, it is {{vendor}} {{model}}, made in {{year}} year.</p> <p>It has {{power}} hp engine with {{speed}} km/h maximum speed.</p> </script>
You can also access templates in JavaScript after app initialization −
var myApp = new Framework7 ({ //Tell Framework7 to compile templates on app init precompileTemplates: true }); // Render person template to HTML, its template is already compiled and accessible as //Template7.templates.personTemplate var personHTML = Template7.templates.personTemplate ({ name: 'King Amit', age: 27, position: 'Developer', company: 'AngularJs' }); // Compile car template to HTML, its template is already compiled and accessible as //Template7.templates.carTemplate var carHTML = Template7.templates.carTemplate({ vendor: 'Honda', model: 'city', power: 1200hp, speed: 300 });
Framework7 - Template7 Pages
Description
Template7 is a mobile-first JavaScript template engine with handlebars.js like syntax. It is ultra lightweight and blazing-fast default template engine in Framework7.
First, we need to pass the following parameter on app initialization that renders all Ajax and dynamic pages as Template7 template −
var myApp = new Framework7 ({ template7Pages: true // enable Template7 rendering for Ajax and Dynamic pages });
S.No | Template7 Pages Usage & Description |
---|---|
1 | Templates/Pages Data
You can pass the required data/context for specific pages by specifying all pages data in template7Data parameter, sent on initializing an App. |
2 | Pass Custom Context
Framework7 allows you to pass custom context to any dynamic page or any loaded Ajax. |
3 | Load Templates Directly
You can render and load templates on fly as dynamic pages. |
4 | URL Query
If you are using Template7 for rendering Ajax pages, its context always will be extended with special property url_query. |
Example
The following example provides the links, which displays the user information such as user details, user likes, etc. when you click on those links.
index.html
<!DOCTYPE html> <html> <head> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Framework7</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "center sliding">Template7 Pages</div> </div> </div> <div class = "pages navbar-through toolbar-through"> <div data-page = "index" class = "page"> <div class = "page-content"> <div class = "list-block"> <ul> <li> //plain data objects allow to pass custom context to loaded page using 'data-context-name' attribute <a href = "#" data-template = "about" data-context-name = "about" class = "item-link item-content"> <div class = "item-inner"> //provides link as 'About' <div class = "item-title">About</div> </div> </a> </li> <li> //a context name for this link we pass context path from template7Data root <a href = "/framework7/src/likes.html" class = "item-link item-content"> <div class = "item-inner"> //provides link as 'Likes' <div class = "item-title">Likes</div> </div> </a> </li> </ul> </div> </div> </div> </div> </div> </div> <script type = "text/template7" id = "about"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left sliding"> <a href = "#" class = "back link"> <i class = "icon icon-back"></i><span>Back</span></a> </div> <div class = "center sliding">About Me</div> <div class = "right"> <a href = "#" class = "link icon-only open-panel"> <i class = "icon icon-bars"></i></a> </div> </div> </div> <div class = "pages"> <div data-page = "about" class = "page"> <div class = "page-content"> <div class = "content-block"> <div class = "content-block-inner"> //displays the details of the user by using the 'my-app.js' file <p>Hello, i am <b>{{firstname}} {{lastname}}</b>, <b>{{age}}</b> years old and working as <b>{{position}}</b> at <b>{{company}}</b>.</p> </div> </div> </div> </div> </div> </script> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script type = "text/javascript" src = "/framework7/src/js/my-app.js"> </script> </body> </html>
my-app.js
// Initialize your app var myApp = new Framework7 ({ animateNavBackIcon: true, // Enable templates auto precompilation precompileTemplates: true, // Enabled rendering pages using Template7 template7Pages: true, // Specify Template7 data for pages template7Data: { //provides the url for different page with data-page = "likes" 'url:likes.html': { likes: [ { title: 'Nelson Mandela', description: 'Champion of Freedom' }, { title: 'Srinivasa Ramanujan', description: 'The Man Who Knew Infinity' }, { title: 'James Cameron', description: 'Famous Filmmaker' } ] }, about: { firstname: 'William ', lastname: 'Root', age: 27, position: 'Developer', company: 'TechShell', } } }); // Add main View var mainView = myApp.addView('.view-main', { // Enable dynamic Navbar dynamicNavbar: true });
likes.html
<div class = "navbar"> <div class = "navbar-inner"> <div class = "left sliding"> <a href = "#" class = "back link"> <i class = "icon icon-back"></i><span>Back</span></a> </div> <div class = "center sliding">Likes</div> <div class = "right"> <a href = "#" class = "link icon-only open-panel"> <i class = "icon icon-bars"></i></a> </div> </div> </div> <div class = "pages"> <div data-page = "likes" class = "page"> <div class = "page-content"> <div class = "content-block-title">My Likes</div> <div class = "list-block media-list"> //iterate through likes <ul> {{#each likes}} <li class = "item-content"> <div class = "item-inner"> <div class = "item-title-row"> //displays the title and description by using the 'my-app.js' file <div class = "item-title">{{title}}</div> </div> <div class = "item-subtitle">{{description}}</div> </div> </li> {{/each}} </ul> </div> </div> </div> </div>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as index.html file in your server root folder.
Open this HTML file as http://localhost/index.html and the output is displayed as shown below.
The example provides the links, which displays the user information such as user details, user likes when you click on those links.
Framework7 - Active State
Description
When you tap the links and buttons, they are highlighted. This is done by active state in Framework7.
- It behaves like a native app, not like a web app..
- It has a built-in Fast clicks library and it should be enabled.
- The active-state class is the same as the CSS :active selector.
- Active state is enabled by adding watch-active-state class to <html> element.
The following code is used for active state in CSS style −
/* Usual state */ .my-button { color: red; } /* Active/tapped state */ .my-button.active-state { color: blue; }
The following code shows the fallback compatibility, when Active state or Fast clicks is disabled −
/* Usual state */ .my-button { color: red; } /* Active/tapped state */ .my-button.active-state { color: blue; } /* Fallback, when active state is disabled */ html:not(.watch-active-state) .my-button:active { color: blue; }
Framework7 - Tap Hold Event
Description
The Tap hold event is used to trigger (enable) after a sustained and complete the touch event so only, it is called tap hold event. The Tab Hold is a built-in Fast Clicks library.
The following parameters are used to disable or enable and configured by default −
S.No | Parameter & Description | Type | Default |
---|---|---|---|
1 | tapHold To enable tap hold events when it is set to true. |
boolean | false |
2 | tapHoldDelay It specifies the duration of holding the tap before triggering taphold event on the target element. |
number | 750 |
3 | tapHoldPreventClicks The tap hold event will not be fired after clicking the event. |
boolean | true |
The following code is used for enable tap hold events −
var myApp = new Framework7 ({ tapHold: true //enable tap hold events }); var $$ = Dom7; $$('.some-link').on('taphold', function () { myApp.alert('Tap hold fired!'); });
Framework7 - Touch Ripple
Description
Touch Ripple is an effect that is supported only in Framework7 material theme. By default, it is enabled in material theme and you can disable it by using the materialRipple:false parameter.
Ripple Elements
You can enable the ripple elements to match some CSS selectors such as −
- ripple
- a.link
- a.item-link
- .button
- .tab-link
- .label-radio
- .label-checkbox etc.
These are specified in the materialRippleElements parameter. You need to enable the touch ripple, add the element's selector to materialRippleElements parameter to make use of ripple effect, or just use the ripple class.
Ripple Wave Color
The color of the ripple can be changed on the element by adding the ripple-[color] class to the element.
For instance −
<a href = "#" class = "button ripple-orange">Ripple Button</a>
or you can define the ripple wave color by using the CSS as shown below −
.button .ripple-wave { background-color: #FFFF00; }
Disable Ripple Elements
You can disable the ripple for some specified elements by adding the no-ripple class to those elements. For instance −
<a href = "#" class = "button no-ripple">Ripple Button</a>