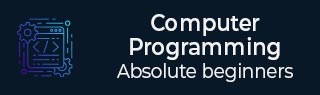
- Computer Programming Tutorial
- Computer Programming - Home
- Computer Programming - Overview
- Computer Programming - Basics
- Programming - Environment
- Programming - Basic Syntax
- Programming - Data Types
- Computer Programming - Variables
- Computer Programming - Keywords
- Computer Programming - Operators
- Computer Programming - Decisions
- Computer Programming - Loops
- Computer Programming - Numbers
- Programming - Characters
- Computer Programming - Arrays
- Computer Programming - Strings
- Computer Programming - Functions
- Computer Programming - File I/O
- Computer Programming - Summary
- Computer Programming Resources
- Programming - Quick Guide
- Computer Programming - Resources
- Programming - Discussion
Computer Programming - File I/O
Computer Files
A computer file is used to store data in digital format like plain text, image data, or any other content. Computer files can be organized inside different directories. Files are used to keep digital data, whereas directories are used to keep files.
Computer files can be considered as the digital counterpart of paper documents. While programming, you keep your source code in text files with different extensions, for example, C programming files end with the extension .c, Java programming files with .java, and Python files with .py.
File Input/Output
Usually, you create files using text editors such as notepad, MS Word, MS Excel or MS Powerpoint, etc. However, many times, we need to create files using computer programs as well. We can modify an existing file using a computer program.
File input means data that is written into a file and file output means data that is read from a file. Actually, input and output terms are more related to screen input and output. When we display a result on the screen, it is called output. Similarly, if we provide some input to our program from the command prompt, then it is called input.
For now, it is enough to remember that writing into a file is file input and reading something from a file is file output.
File Operation Modes
Before we start working with any file using a computer program, either we need to create a new file if it does not exist or open an already existing file. In either case, we can open a file in the following modes −
Read-Only Mode − If you are going to just read an existing file and you do not want to write any further content in the file, then you will open the file in read-only mode. Almost all the programming languages provide syntax to open files in read-only mode.
Write-Only Mode − If you are going to write into either an existing file or a newly created file but you do not want to read any written content from that file, then you will open the file in write-only mode. All the programming languages provide syntax to open files in write-only mode.
Read & Write Mode − If you are going to read as well as write into the same file, then you will open file in read & write mode.
Append Mode − When you open a file for writing, it allows you to start writing from the beginning of the file; however it will overwrite existing content, if any. Suppose we don’t want to overwrite any existing content, then we open the file in append mode. Append mode is ultimately a write mode, which allows content to be appended at the end of the file. Almost all the programming languages provide syntax to open files in append mode.
In the following sections, we will learn how to open a fresh new file, how to write into it, and later, how to read and append more content into the same file.
Opening Files
You can use the fopen() function to create a new file or to open an existing file. This call will initialize an object of the type FILE, which contains all the information necessary to control the stream. Here is the prototype, i.e., signature of this function call −
FILE *fopen( const char * filename, const char * mode );
Here, filename is string literal, which you will use to name your file and access mode can have one of the following values −
Sr.No | Mode & Description |
---|---|
1 |
r Opens an existing text file for reading purpose. |
2 |
w Opens a text file for writing. If it does not exist, then a new file is created. Here, your program will start writing content from the beginning of the file. |
3 |
a Opens a text file for writing in appending mode. If it does not exist, then a new file is created. Here, your program will start appending content in the existing file content. |
4 | r+ Opens a text file for reading and writing both. |
5 |
w+ Opens a text file for both reading and writing. It first truncates the file to zero length, if it exists; otherwise creates the file if it does not exist. |
6 |
a+ Opens a text file for both reading and writing. It creates a file, if it does not exist. The reading will start from the beginning, but writing can only be appended. |
Closing a File
To close a file, use the fclose( ) function. The prototype of this function is −
int fclose( FILE *fp );
The fclose( ) function returns zero on success, or EOF, special character, if there is an error in closing the file. This function actually flushes any data still pending in the buffer to the file, closes the file, and releases any memory used for the file. The EOF is a constant defined in the header file stdio.h.
There are various functions provided by C standard library to read and write a file character by character or in the form of a fixed length string. Let us see a few of them in the next section.
Writing a File
Given below is the simplest function to write individual characters to a stream −
int fputc( int c, FILE *fp );
The function fputc() writes the character value of the argument c to the output stream referenced by fp. It returns the written character written on success, otherwise EOF if there is an error. You can use the following functions to write a null-terminated string to a stream −
int fputs( const char *s, FILE *fp );
The function fputs() writes the string s into the file referenced by fp. It returns a non-negative value on success, otherwise EOF is returned in case of any error. You can also use the function int fprintf(FILE *fp,const char *format, ...) to write a string into a file. Try the following example −
#include <stdio.h> int main() { FILE *fp; fp = fopen("/tmp/test.txt", "w+"); fprintf(fp, "This is testing for fprintf...\n"); fputs("This is testing for fputs...\n", fp); fclose(fp); }
When the above code is compiled and executed, it creates a new file test.txt in /tmp directory and writes two lines using two different functions. Let us read this file in the next section.
Reading a File
Given below is the simplest function to read a text file character by character −
int fgetc( FILE * fp );
The fgetc() function reads a character from the input file referenced by fp. The return value is the character read; or in case of any error, it returns EOF. The following function allows you to read a string from a stream −
char *fgets( char *buf, int n, FILE *fp );
The function fgets() reads up to n - 1 characters from the input stream referenced by fp. It copies the read string into the buffer buf, appending a null character to terminate the string.
If this function encounters a newline character '\n' or EOF before they have read the maximum number of characters, then it returns only the characters read up to that point including the new line character. You can also use int fscanf(FILE *fp, const char *format, ...) to read strings from a file, but it stops reading after encountering the first space character.
#include <stdio.h> main() { FILE *fp; char buff[255]; fp = fopen("/tmp/test.txt", "r"); fscanf(fp, "%s", buff); printf("1 : %s\n", buff ); fgets(buff, 255, (FILE*)fp); printf("2: %s\n", buff ); fgets(buff, 255, (FILE*)fp); printf("3: %s\n", buff ); fclose(fp); }
When the above code is compiled and executed, it reads the file created in the previous section and produces the following result −
1 : This 2 : is testing for fprintf... 3 : This is testing for fputs...
Let's analyze what happened here. First, the fscanf() method reads This because after that, it encountered a space. The second call is for fgets(), which reads the remaining line till it encountered end of line. Finally, the last call fgets() reads the second line completely.
File I/O in Java
Java provides even richer set of functions to handle File I/O. For more on this topic, we suggest you to check our Java Tutorials.
Here, we will see a simple Java program, which is equivalent to the C program explained above. This program will open a text file, write a few text lines into it, and close the file. Finally, the same file is opened and then read from an already created file. You can try to execute the following program to see the output −
import java.io.*; public class DemoJava { public static void main(String []args) throws IOException { File file = new File("/tmp/java.txt"); // Create a File file.createNewFile(); // Creates a FileWriter Object using file object FileWriter writer = new FileWriter(file); // Writes the content to the file writer.write("This is testing for Java write...\n"); writer.write("This is second line...\n"); // Flush the memory and close the file writer.flush(); writer.close(); // Creates a FileReader Object FileReader reader = new FileReader(file); char [] a = new char[100]; // Read file content in the array reader.read(a); System.out.println( a ); // Close the file reader.close(); } }
When the above program is executed, it produces the following result −
This is testing for Java write... This is second line...
File I/O in Python
The following program shows the same functionality to open a new file, write some content into it, and finally, read the same file −
# Create a new file fo = open("/tmp/python.txt", "w") # Writes the content to the file fo.write( "This is testing for Python write...\n"); fo.write( "This is second line...\n"); # Close the file fo.close() # Open existing file fo = open("/tmp/python.txt", "r") # Read file content in a variable str = fo.read(100); print str # Close opened file fo.close()
When the above code is executed, it produces the following result −
This is testing for Python write... This is second line...