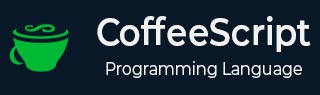
- CoffeeScript Tutorial
- CoffeeScript - Home
- CoffeeScript - Overview
- CoffeeScript - Environment
- CoffeeScript - command-line utility
- CoffeeScript - Syntax
- CoffeeScript - Data Types
- CoffeeScript - Variables
- CoffeeScript - Operators and Aliases
- CoffeeScript - Conditionals
- CoffeeScript - Loops
- CoffeeScript - Comprehensions
- CoffeeScript - Functions
- CoffeeScript Object Oriented
- CoffeeScript - Strings
- CoffeeScript - Arrays
- CoffeeScript - Objects
- CoffeeScript - Ranges
- CoffeeScript - Splat
- CoffeeScript - Date
- CoffeeScript - Math
- CoffeeScript - Exception Handling
- CoffeeScript - Regular Expressions
- CoffeeScript - Classes and Inheritance
- CoffeeScript Advanced
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript - MongoDB
- CoffeeScript - SQLite
- CoffeeScript Useful Resources
- CoffeeScript - Quick Guide
- CoffeeScript - Useful Resources
- CoffeeScript - Discussion
CoffeeScript - Syntax
In the previous chapter, we have seen how to install CoffeeScript. In this chapter, let us check out the syntax of CoffeeScript.
The syntax of CoffeeScript is more graceful when compared to the syntax of JavaScript. It avoids the troublesome features like curly braces, semicolons, and variable decelerations.
CoffeeScript Statements
Unlike many other programming languages like C, C++, and Java, the statements in CoffeeScript do not end with semicolons (;). Instead of that, every new line is considered as a separate statement by the CoffeeScript compiler.
Example
Here is an example of a CoffeeScript statement.
name = "Javed" age = 26
In the same way, we can write two statements in a single line by separating them using semicolon as shown below.
name = "Javed";age = 26
CoffeeScript Variables (No var Keyword)
In JavaScript, we declare a variable using the var keyword before creating it, as shown below.
var name = "Javed" var age = 20
While creating variables in CoffeeScript, there is no need to declare them using the var keyword. We can directly create a variable just by assigning a value to it as shown below.
name = "Javed" age = 20
No Parentheses
In general, we use parenthesis while declaring the function, calling it, and also to separate the code blocks to avoid ambiguity. In CoffeeScript, there is no need to use parentheses, and while creating functions, we use an arrow mark (->) instead of parentheses as shown below.
myfunction = -> alert "Hello"
Still, we have to use parentheses in certain scenarios. While calling functions without parameters, we will use parentheses. For example, if we have a function named my_function in CoffeeScript, then we have to call it as shown below.
my_function()
In the same way, we can also separate the ambiguous code using parentheses. If you observe the following example, without braces, the result is 2233 and with braces, it will be 45.
alert "The result is "+(22+33)
No Curly Braces
In JavaScript, for the block codes such as functions, loops, and conditional statements, we use curly braces. In CoffeeScript, there is no need to use curly braces. Instead, we have to maintain proper indentations (white spaces) within the body. This is the feature which is inspired from the Python language.
Following is an example of a function in CoffeeScript. Here you can observe that instead of curly braces, we have used three whitespaces as indentation to separate the body of the function.
myfunction = -> name = "John" alert "Hello"+name
CoffeeScript Comments
In any programming language, we use comments to write description about the code we have written. These comments are not considered as the part of the programs. The comments in CoffeeScript are similar to the comments of Ruby language. CoffeeScript provides two types of comments as follows −
Single-line Comments
Whenever we want to comment a single line in CoffeeScript, we just need to place a hash tag before it as shown below.
# This is the single line comment in CoffeeScript
Every single line that follows a hash tag (#) is considered as a comment by the CoffeeScript compiler and it compiles the rest of the code in the given file except the comments.
Multiline Comments
Whenever we want to comment more than one line in CoffeeScript (multiple lines), we can do that by wrapping those lines within a pair of triple hash tags as shown below.
### These are the multi line comments in CoffeeScript We can write as many number of lines as we want within the pair of triple hash tags. ###
CoffeeScript Reserved keywords
A list of all the reserved words in CoffeeScript are given in the following table. They cannot be used as CoffeeScript variables, functions, methods, loop labels, or any object names.
case default function var void with const let enum export import native __hasProp __extends __slice __bind __indexOf implements |
else interface package private protected public static yield true false null this new delete typeof in arguments eval |
instanceof return throw break continue debugger if else switch for while do try catch finally class extends super |
undefined then unless until loop of by when and or is isnt not yes no on off |