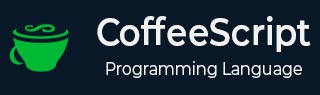
- CoffeeScript Tutorial
- CoffeeScript - Home
- CoffeeScript - Overview
- CoffeeScript - Environment
- CoffeeScript - command-line utility
- CoffeeScript - Syntax
- CoffeeScript - Data Types
- CoffeeScript - Variables
- CoffeeScript - Operators and Aliases
- CoffeeScript - Conditionals
- CoffeeScript - Loops
- CoffeeScript - Comprehensions
- CoffeeScript - Functions
- CoffeeScript Object Oriented
- CoffeeScript - Strings
- CoffeeScript - Arrays
- CoffeeScript - Objects
- CoffeeScript - Ranges
- CoffeeScript - Splat
- CoffeeScript - Date
- CoffeeScript - Math
- CoffeeScript - Exception Handling
- CoffeeScript - Regular Expressions
- CoffeeScript - Classes and Inheritance
- CoffeeScript Advanced
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript - MongoDB
- CoffeeScript - SQLite
- CoffeeScript Useful Resources
- CoffeeScript - Quick Guide
- CoffeeScript - Useful Resources
- CoffeeScript - Discussion
CoffeeScript - Date
The Date object is a data-type built into the JavaScript language. Date objects are created as new Date( ).
Once a Date object is created, a number of methods allow you to operate on it. Most methods simply allow you to get and set the year, month, day, hour, minute, second, and millisecond fields of the object, using either local time or UTC (universal, or GMT) time.
The ECMAScript standard requires the Date object to be able to represent any date and time, to millisecond precision, within 100 million days before or after 1/1/1970. This is a range of plus or minus 273,785 years, so JavaScript can represent date and time till the year 275755.
Similar to other JavaScript objects we can also use the date object in our CoffeeScript code.
Date Methods
Following is the list of methods of the Date object of JavaScript. Click on the name of these methods to get an example demonstrating their usage in CoffeeScript.
S.No. | Method & Description |
---|---|
1 | getDate()
Returns the day of the month for the specified date according to local time. |
2 | getDay()
Returns the day of the week for the specified date according to local time. |
3 | getFullYear()
Returns the year of the specified date according to local time. |
4 | getHours()
Returns the hour in the specified date according to local time. |
5 | getMilliseconds()
Returns the milliseconds in the specified date according to local time. |
6 | getMinutes()
Returns the minutes in the specified date according to local time. |
7 | getMonth()
Returns the month in the specified date according to local time. |
8 | getSeconds()
Returns the seconds in the specified date according to local time. |
9 | getTime()
Returns the numeric value of the specified date as the number of milliseconds since January 1, 1970, 00:00:00 UTC. |
10 | getTimezoneOffset()
Returns the time-zone offset in minutes for the current locale. |
11 | getUTCDate()
Returns the day (date) of the month in the specified date according to universal time. |
12 | getUTCDay()
Returns the day of the week in the specified date according to universal time. |
13 | getUTCFullYear()
Returns the year in the specified date according to universal time. |
14 | getUTCHours()
Returns the hours in the specified date according to universal time. |
15 | getUTCMinutes()
Returns the milliseconds in the specified date according to universal time. |
16 | getUTCMilliseconds()
Returns the minutes in the specified date according to universal time. |
17 | getUTCMonth()
Returns the month in the specified date according to universal time. |
18 | getUTCSeconds()
Returns the seconds in the specified date according to universal time. |
19 | getYear()
Deprecated - Returns the year in the specified date according to local time. Use getFullYear instead. |
20 | setDate()
Sets the day of the month for a specified date according to local time. |
21 | setFullYear()
Sets the full year for a specified date according to local time. |
22 | setHours()
Sets the hours for a specified date according to local time. |
23 | setMilliseconds()
Sets the milliseconds for a specified date according to local time. |
24 | setMinutes()
Sets the minutes for a specified date according to local time. |
25 | setMonth()
Sets the month for a specified date according to local time. |
26 | setSeconds()
Sets the seconds for a specified date according to local time. |
27 | setTime()
Sets the Date object to the time represented by a number of milliseconds since January 1, 1970, 00:00:00 UTC. |
28 | setUTCDate()
Sets the day of the month for a specified date according to universal time. |
29 | setUTCFullYear()
Sets the full year for a specified date according to universal time. |
30 | setUTCHours()
Sets the hour for a specified date according to universal time. |
31 | setUTCMilliseconds()
Sets the milliseconds for a specified date according to universal time. |
32 | setUTCMinutes()
Sets the minutes for a specified date according to universal time. |
33 | setUTCMonth()
Sets the month for a specified date according to universal time. |
34 | setUTCSeconds()
Sets the seconds for a specified date according to universal time. |
35 | setYear()
Deprecated - Sets the year for a specified date according to local time. Use setFullYear instead. |
36 | toDateString()
Returns the "date" portion of the Date as a human-readable string. |
37 | toLocaleDateString()
Returns the "date" portion of the Date as a string, using the current locale's conventions. |
38 | toLocaleString()
Converts a date to a string, using the current locale's conventions. |
39 | toLocaleTimeString()
Returns the "time" portion of the Date as a string, using the current locale's conventions. |
40 | toTimeString()
Returns the "time" portion of the Date as a human-readable string. |
41 | toUTCString()
Converts a date to a string, using the universal time convention. |