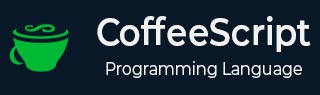
- CoffeeScript Tutorial
- CoffeeScript - Home
- CoffeeScript - Overview
- CoffeeScript - Environment
- CoffeeScript - command-line utility
- CoffeeScript - Syntax
- CoffeeScript - Data Types
- CoffeeScript - Variables
- CoffeeScript - Operators and Aliases
- CoffeeScript - Conditionals
- CoffeeScript - Loops
- CoffeeScript - Comprehensions
- CoffeeScript - Functions
- CoffeeScript Object Oriented
- CoffeeScript - Strings
- CoffeeScript - Arrays
- CoffeeScript - Objects
- CoffeeScript - Ranges
- CoffeeScript - Splat
- CoffeeScript - Date
- CoffeeScript - Math
- CoffeeScript - Exception Handling
- CoffeeScript - Regular Expressions
- CoffeeScript - Classes and Inheritance
- CoffeeScript Advanced
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript - MongoDB
- CoffeeScript - SQLite
- CoffeeScript Useful Resources
- CoffeeScript - Quick Guide
- CoffeeScript - Useful Resources
- CoffeeScript - Discussion
CoffeeScript - Assignment operators
CoffeeScript supports the following assignment operators −
Sr.No | Operator and Description | Example |
---|---|---|
1 | = (Simple Assignment ) Assigns values from the right side operand to the left side operand |
C = A + B will assign the value of A + B into C |
2 | += (Add and Assignment) It adds the right operand to the left operand and assigns the result to the left operand. |
C += A is equivalent to C = C + A |
3 | -= (Subtract and Assignment) It subtracts the right operand from the left operand and assigns the result to the left operand. |
C -= A is equivalent to C = C - A |
4 | *= (Multiply and Assignment) It multiplies the right operand with the left operand and assigns the result to the left operand. |
C *= A is equivalent to C = C * A |
5 | /= (Divide and Assignment) It divides the left operand with the right operand and assigns the result to the left operand. |
C /= A is equivalent to C = C / A |
6 | %= (Modules and Assignment) It takes modulus using two operands and assigns the result to the left operand. |
C %= A is equivalent to C = C % A |
Note − Same logic applies to Bitwise operators so they will become like <<=, >>=, >>=, &=, |= and ^=.
Example
The following example demonstrates the usage of assignment operators in CoffeeScript. Save this code in a file with name assignment _example.coffee
a = 33 b = 10 console.log "The value of a after the operation (a = b) is " result = a = b console.log result console.log "The value of a after the operation (a += b) is " result = a += b console.log result console.log "The value of a after the operation (a -= b) is " result = a -= b console.log result console.log "The value of a after the operation (a *= b) is " result = a *= b console.log result console.log "The value of a after the operation (a /= b) is " result = a /= b console.log result console.log "The value of a after the operation (a %= b) is " result = a %= b console.log result
Open the command prompt and compile the .coffee file as shown below.
c:/> coffee -c assignment _example.coffee
On compiling, it gives you the following JavaScript.
// Generated by CoffeeScript 1.10.0 (function() { var a, b, result; a = 33; b = 10; console.log("The value of a after the operation (a = b) is "); result = a = b; console.log(result); console.log("The value of a after the operation (a += b) is "); result = a += b; console.log(result); console.log("The value of a after the operation (a -= b) is "); result = a -= b; console.log(result); console.log("The value of a after the operation (a *= b) is "); result = a *= b; console.log(result); console.log("The value of a after the operation (a /= b) is "); result = a /= b; console.log(result); console.log("The value of a after the operation (a %= b) is "); result = a %= b; console.log(result); }).call(this);
Now, open the command prompt again and run the CoffeeScript file as shown below.
c:/> coffee assignment _example.coffee
On executing, the CoffeeScript file produces the following output.
The value of a after the operation (a = b) is 10 The value of a after the operation (a += b) is 20 The value of a after the operation (a -= b) is 10 The value of a after the operation (a *= b) is 100 The value of a after the operation (a /= b) is 10 The value of a after the operation (a %= b) is 0