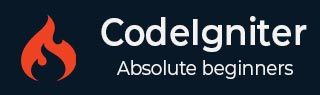
- CodeIgniter Tutorial
- CodeIgniter - Home
- CodeIgniter - Overview
- CodeIgniter - Installing CodeIgniter
- CodeIgniter - Application Architecture
- CodeIgniter - MVC Framework
- CodeIgniter - Basic Concepts
- CodeIgniter - Configuration
- CodeIgniter - Working with Database
- CodeIgniter - Libraries
- CodeIgniter - Error Handling
- CodeIgniter - File Uploading
- CodeIgniter - Sending Email
- CodeIgniter - Form Validation
- CodeIgniter - Session Management
- CodeIgniter - Flashdata
- CodeIgniter - Tempdata
- CodeIgniter - Cookie Management
- CodeIgniter - Common Functions
- CodeIgniter - Page Caching
- CodeIgniter - Page Redirection
- CodeIgniter - Application Profiling
- CodeIgniter - Benchmarking
- CodeIgniter - Adding JS and CSS
- CodeIgniter - Internationalization
- CodeIgniter - Security
- CodeIgniter Useful Resources
- CodeIgniter - Quick Guide
- CodeIgniter - Useful Resources
- CodeIgniter - Discussion
CodeIgniter - Page Redirection
While building web application, we often need to redirect the user from one page to another page. CodeIgniter makes this job easy for us. The redirect() function is used for this purpose.
Syntax |
redirect($uri = '', $method = 'auto', $code = NULL) |
Parameters |
|
Return type |
void |
The first argument can have two types of URI. We can pass full site URL or URI segments to the controller you want to direct.
The second optional parameter can have any of the three values from auto, location or refresh. The default is auto.
The third optional parameter is only available with location redirects and it allows you to send specific HTTP response code.
Example
Create a controller called Redirect_controller.php and save it in application/controller/Redirect_controller.php
<?php class Redirect_controller extends CI_Controller { public function index() { /*Load the URL helper*/ $this->load->helper('url'); /*Redirect the user to some site*/ redirect('https://www.tutorialspoint.com'); } public function computer_graphics() { /*Load the URL helper*/ $this->load->helper('url'); redirect('https://www.tutorialspoint.com/computer_graphics/index.htm'); } public function version2() { /*Load the URL helper*/ $this->load->helper('url'); /*Redirect the user to some internal controller’s method*/ redirect('redirect/computer_graphics'); } } ?>
Change the routes.php file in application/config/routes.php to add route for the above controller and add the following line at the end of the file.
$route['redirect'] = 'Redirect_controller'; $route['redirect/version2'] = 'Redirect_controller/version2'; $route['redirect/computer_graphics'] = 'Redirect_controller/computer_graphics';
Type the following URL in the browser, to execute the example.
http://yoursite.com/index.php/redirect
The above URL will redirect you to the tutorialspoint.com website and if you visit the following URL, then it will redirect you to the computer graphics tutorial at tutorialspoint.com.
http://yoursite.com/index.php/redirect/computer_graphics