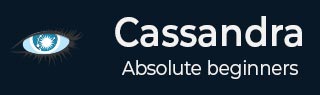
- Cassandra Tutorial
- Cassandra - Home
- Cassandra - Introduction
- Cassandra - Architecture
- Cassandra - Data Model
- Cassandra - Installation
- Cassandra - Referenced Api
- Cassandra - Cqlsh
- Cassandra - Shell Commands
- Cassandra Keyspace Operations
- Cassandra - Create Keyspace
- Cassandra - Alter Keyspace
- Cassandra - Drop Keyspace
- Cassandra Table Operations
- Cassandra - Create Table
- Cassandra - Alter Table
- Cassandra - Drop Table
- Cassandra - Truncate Table
- Cassandra - Create Index
- Cassandra - Drop Index
- Cassandra - Batch
- Cassandra CURD Operations
- Cassandra - Create Data
- Cassandra - Update Data
- Cassandra - Read Data
- Cassandra - Delete Data
- Cassandra CQL Types
- Cassandra - CQL Datatypes
- Cassandra - CQL Collections
- CQL User Defined Datatypes
- Cassandra Useful Resources
- Cassandra - Quick Guide
- Cassandra - Useful Resources
- Cassandra - Discussion
Cassandra - Update Data
Updating Data in a Table
UPDATE is the command used to update data in a table. The following keywords are used while updating data in a table −
Where − This clause is used to select the row to be updated.
Set − Set the value using this keyword.
Must − Includes all the columns composing the primary key.
While updating rows, if a given row is unavailable, then UPDATE creates a fresh row. Given below is the syntax of UPDATE command −
UPDATE <tablename> SET <column name> = <new value> <column name> = <value>.... WHERE <condition>
Example
Assume there is a table named emp. This table stores the details of employees of a certain company, and it has the following details −
emp_id | emp_name | emp_city | emp_phone | emp_sal |
---|---|---|---|---|
1 | ram | Hyderabad | 9848022338 | 50000 |
2 | robin | Hyderabad | 9848022339 | 40000 |
3 | rahman | Chennai | 9848022330 | 45000 |
Let us now update emp_city of robin to Delhi, and his salary to 50000. Given below is the query to perform the required updates.
cqlsh:tutorialspoint> UPDATE emp SET emp_city='Delhi',emp_sal=50000 WHERE emp_id=2;
Verification
Use SELECT statement to verify whether the data has been updated or not. If you verify the emp table using SELECT statement, it will produce the following output.
cqlsh:tutorialspoint> select * from emp; emp_id | emp_city | emp_name | emp_phone | emp_sal --------+-----------+----------+------------+--------- 1 | Hyderabad | ram | 9848022338 | 50000 2 | Delhi | robin | 9848022339 | 50000 3 | Chennai | rahman | 9848022330 | 45000 (3 rows)
Here you can observe the table data has got updated.
Updating Data using Java API
You can update data in a table using the execute() method of Session class. Follow the steps given below to update data in a table using Java API.
Step1: Create a Cluster Object
Create an instance of Cluster.builder class of com.datastax.driver.core package as shown below.
//Creating Cluster.Builder object Cluster.Builder builder1 = Cluster.builder();
Add a contact point (IP address of the node) using the addContactPoint() method of Cluster.Builder object. This method returns Cluster.Builder.
//Adding contact point to the Cluster.Builder object Cluster.Builder builder2 = build.addContactPoint("127.0.0.1");
Using the new builder object, create a cluster object. To do so, you have a method called build() in the Cluster.Builder class. Use the following code to create the cluster object.
//Building a cluster Cluster cluster = builder.build();
You can build the cluster object using a single line of code as shown below.
Cluster cluster = Cluster.builder().addContactPoint("127.0.0.1").build();
Step 2: Create a Session Object
Create an instance of Session object using the connect() method of Cluster class as shown below.
Session session = cluster.connect( );
This method creates a new session and initializes it. If you already have a keyspace, then you can set it to the existing one by passing the KeySpace name in string format to this method as shown below.
Session session = cluster.connect(“ Your keyspace name”);
Here we are using the KeySpace named tp. Therefore, create the session object as shown below.
Session session = cluster.connect(“tp”);
Step 3: Execute Query
You can execute CQL queries using the execute() method of Session class. Pass the query either in string format or as a Statement class object to the execute() method. Whatever you pass to this method in string format will be executed on the cqlsh.
In the following example, we are updating the emp table. You have to store the query in a string variable and pass it to the execute() method as shown below:
String query = “ UPDATE emp SET emp_city='Delhi',emp_sal=50000 WHERE emp_id = 2;” ;
Given below is the complete program to update data in a table using Java API.
import com.datastax.driver.core.Cluster; import com.datastax.driver.core.Session; public class Update_Data { public static void main(String args[]){ //query String query = " UPDATE emp SET emp_city='Delhi',emp_sal=50000" //Creating Cluster object Cluster cluster = Cluster.builder().addContactPoint("127.0.0.1").build(); //Creating Session object Session session = cluster.connect("tp"); //Executing the query session.execute(query); System.out.println("Data updated"); } }
Save the above program with the class name followed by .java, browse to the location where it is saved. Compile and execute the program as shown below.
$javac Update_Data.java $java Update_Data
Under normal conditions, it should produce the following output −
Data updated