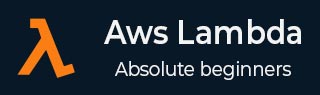
- AWS Lambda Tutorial
- AWS Lambda - Home
- AWS Lambda - Overview
- AWS Lambda - Environment Setup
- AWS Lambda - Introduction
- Building the Lambda function
- Function in NODEJS
- Function in Java
- Function in Python
- Function in Go
- Function in C#
- Configuring Lambda Function
- Creating & Deploying using AWS Console
- Creating & Deploying using AWS CLI
- Creating & Deploying using Serverless Framework
- AWS Executing & Invoking Lambda Function
- Deleting Lambda Function
- Working with Amazon API Gateway
- Lambda Function with Amazon S3
- Lambda Function with Amazon DynamoDB
- Lambda Function with Scheduled Events
- Lambda Function with Amazon SNS
- Lambda Function with CloudTrail
- Lambda Function with Amazon Kinesis
- Lambda Function with Custom User Applications
- AWS Lambda@Edge with CloudFront
- Monitoring and TroubleShooting using Cloudwatch
- AWS Lambda - Additional Example
- AWS Lambda Useful resources
- AWS Lambda - Quick Guide
- AWS Lambda - Useful resources
- AWS Lambda - Discussion
Monitoring and TroubleShooting using Cloudwatch
Functions created in AWS Lambda are monitored by Amazon CloudWatch. It helps in logging all the requests made to the Lambda function when it is triggered.
Consider that the following code is uploaded in AWS Lambda with function name as lambda and cloudwatch.
exports.handler = (event, context, callback) => { // TODO implement console.log("Lambda monitoring using amazon cloudwatch"); callback(null, 'Hello from Lambda'); };
When the function is tested or triggered, you should see an entry in Cloudwatch. For this purpose, go to AWS services and click CloudWatch.
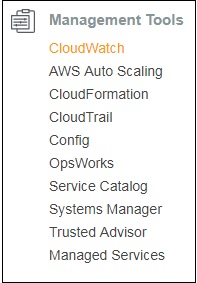
Select logs from left side.
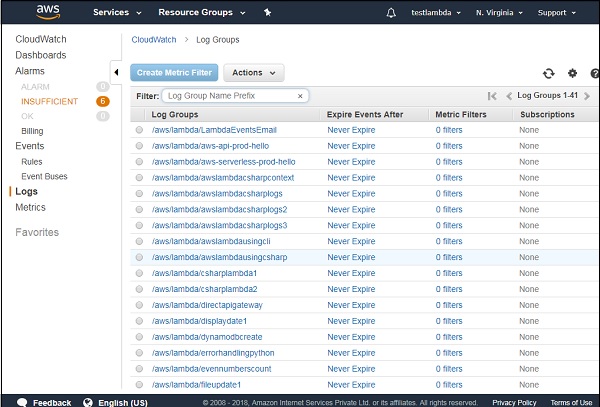
When you click Logs, it has the Log Groups of AWS Lambda function created in your account. Select anyAWS Lambda function and check the details. Here, we are referring to Lambda function with name:lambdaandcloudwatch. The logs added to the Lambda function are displayed here as shown below −
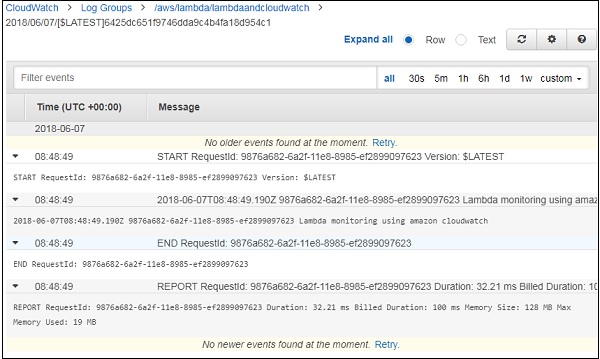
Now, let us add S3 trigger to the Lambda function and see the logs details in CloudWatch as shown below −
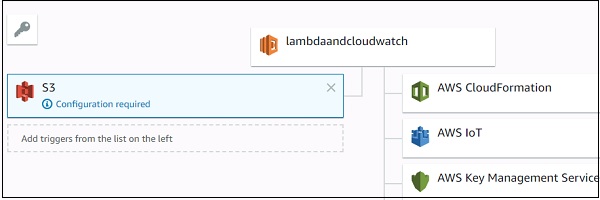
Let us update AWS Lambda code to display the file uploaded and bucket name as shown in the code given below −
exports.handler = (event, context, callback) => { // TODO implement console.log("Lambda monitoring using amazon cloudwatch"); const bucket = event.Records[0].s3.bucket.name; const filename = event.Records[0].s3.object.key; const message = `File is uploaded in - ${bucket} -> ${filename}`; console.log(message); callback(null, 'Hello from Lambda'); };
Now, add file in s3storetestlambdaEventbucket as shown −
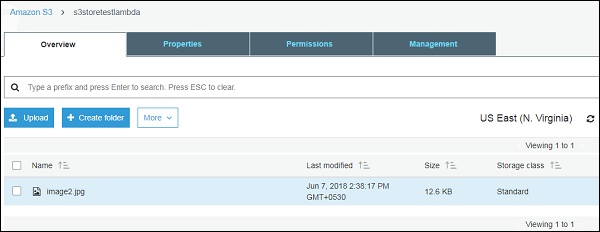
When the file is uploaded, AWS Lambda functions will get triggered and the console log messages from Lambda code are displayed in CloudWatch as shown below −
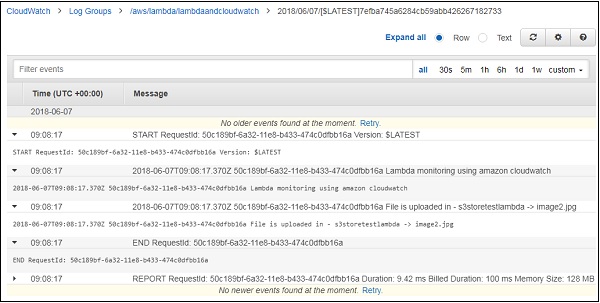
If there is any error, CloudWatch gives the error details as shown below −
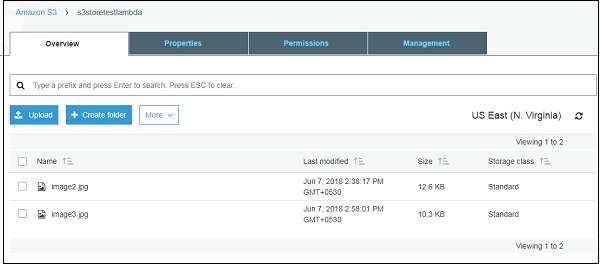
Note that we have referred to the bucket name wrongly in AWS Lambda code as shown −
exports.handler = (event, context, callback) => { // TODO implement console.log("Lambda monitoring using amazon cloudwatch"); const bucket = event.Records[0].bucket.name; const filename = event.Records[0].s3.object.key; const message = `File is uploaded in - ${bucket} -> ${filename}`; console.log(message); callback(null, 'Hello from Lambda'); };
The bucket name reference from the event is wrong. Thus, we should see an error displayed in CloudWatch as shown below −
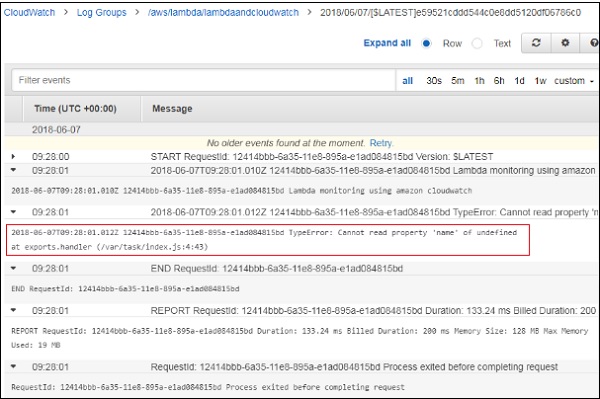
CloudWatch Metrics
The details of the Lambda function execution can be seen in the metrics. Click Metrics displayed in the left side.
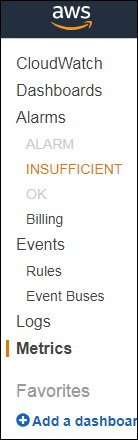
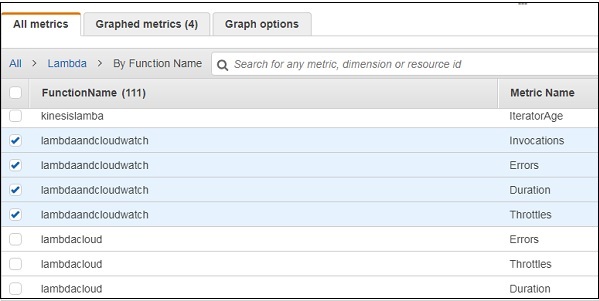
The graph details for the lambda function lambdaandcloudwatch are as shown below −
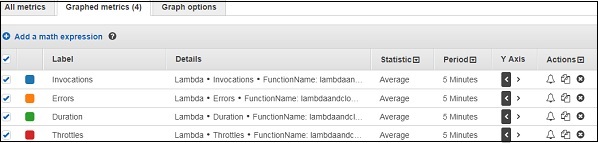
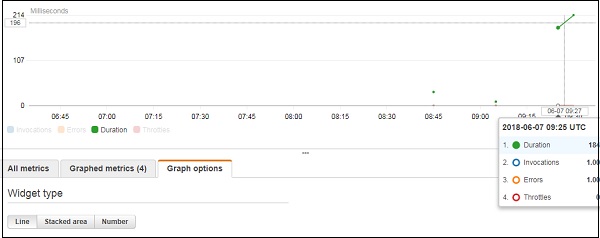
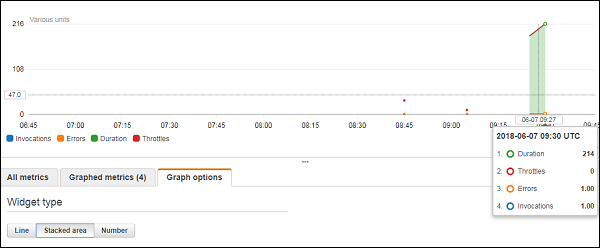
It gives details such as the duration for which the Lambda function is executed, number of times it is invoked and the errors from the Lambda function.