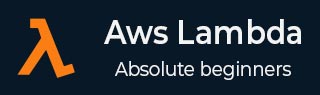
- AWS Lambda Tutorial
- AWS Lambda - Home
- AWS Lambda - Overview
- AWS Lambda - Environment Setup
- AWS Lambda - Introduction
- Building the Lambda function
- Function in NODEJS
- Function in Java
- Function in Python
- Function in Go
- Function in C#
- Configuring Lambda Function
- Creating & Deploying using AWS Console
- Creating & Deploying using AWS CLI
- Creating & Deploying using Serverless Framework
- AWS Executing & Invoking Lambda Function
- Deleting Lambda Function
- Working with Amazon API Gateway
- Lambda Function with Amazon S3
- Lambda Function with Amazon DynamoDB
- Lambda Function with Scheduled Events
- Lambda Function with Amazon SNS
- Lambda Function with CloudTrail
- Lambda Function with Amazon Kinesis
- Lambda Function with Custom User Applications
- AWS Lambda@Edge with CloudFront
- Monitoring and TroubleShooting using Cloudwatch
- AWS Lambda - Additional Example
- AWS Lambda Useful resources
- AWS Lambda - Quick Guide
- AWS Lambda - Useful resources
- AWS Lambda - Discussion
AWS Lambda – Function in Java
In this chapter, let us understand in detail how to create a simple AWS Lambda function in Java in detail.
Creating JAR file in Eclipse
Before proceeding to work on creating a lambda function in AWS, we need AWS toolkit support for Eclipse. For any guidance on installation of the same, you can refer to the Environment Setup chapter in this tutorial.
Once you are done with installation, follow the steps given here −
Step 1
Open Eclipse IDE and create a new project with AWS Lambda Java Project. Observe the screenshot given below for better understanding −
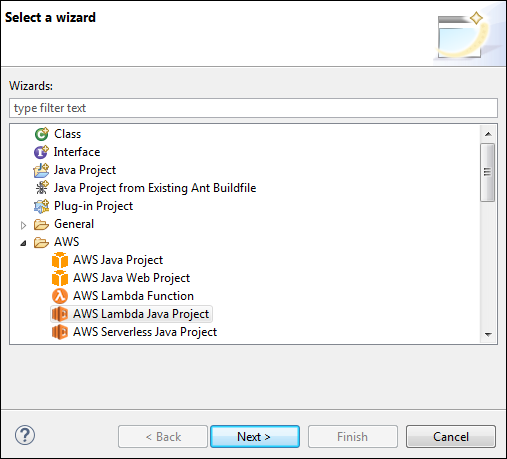
Step 2
Once you select Next, it will redirect you the screen shown below −
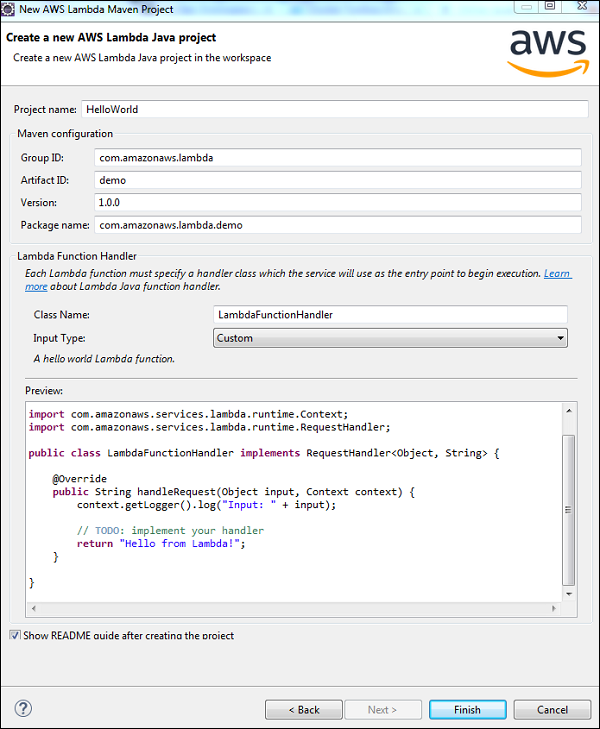
Step 3
Now, a default code is created for Input Type Custom. Once you click Finish button the project gets created as shown below −
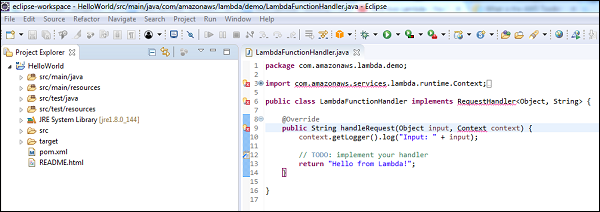
Step 4
Now, right click your project and export it. Select Java / JAR file from the Export wizard and click Next.
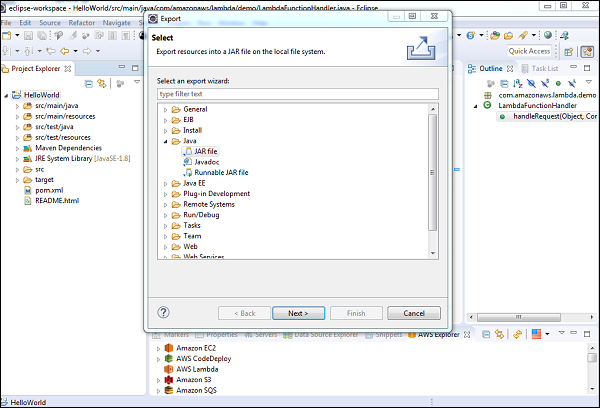
Step 5
Now, if you click Next, you will be prompted save the file in the destination folder which will be asked when you click on next.
Once the file is saved, go back to AWS Console and create the AWS Lambda function for Java.
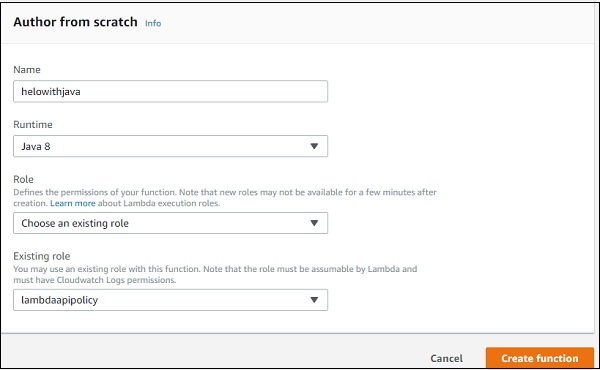
Step 6
Now, upload the .jar file that we created using the Upload button as shown in the screenshot given below −
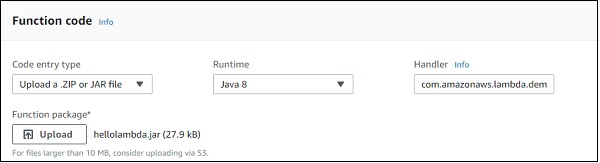
Handler Details for Java
Handler is package name and class name. Look at the following example to understand handler in detail −
Example
package com.amazonaws.lambda.demo; import com.amazonaws.services.lambda.runtime.Context; import com.amazonaws.services.lambda.runtime.RequestHandler; public class LambdaFunctionHandler implements RequestHandler
Observe that from the above code, the handler will be com.amazonaws.lambda.demo.LambdaFunctionHandler
Now, let us test the changes and see the output −
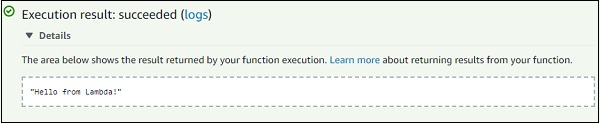
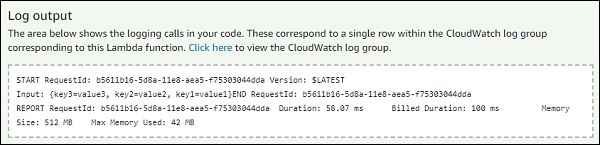
Context Object in Java
Interaction with AWS Lambda execution is done using the context. It provides following methods to be used inside Java −
Sr.No | Context Methods & Description |
---|---|
1 | getMemoryLimitInMB() this will give the memory limit you specified while creating lambda function. |
2 | getFunctionName() this will give the name of the lambda function. |
3 | getFunctionVersion() this will give the version of the lambda function running. |
4 | getInvokedFunctionArn() this will give the ARN used to invoke the function. |
5 | getAwsRequestId() this will give the aws request id. This id gets created for the lambda function and it is unique. The id can be used with aws support incase if you face any issues. |
6 | getLogGroupName() this will give the aws cloudwatch group name linked with aws lambda function created. It will be null if the iam user is not having permission for cloudwatch logging. |
7 | getClientContext() this will give details about the app and device when used with aws mobile sdk. It will give details like version name and code, client id, title, app package name. It can be null. |
8 | getIdentity() this will give details about the amazon cognito identity when used with aws mobile sdk. It can be null. |
9 | getRemainingTimeInMillis() this will give the remaining time execution in milliseconds when the function is terminated after the specified timeout. |
10 | getLogger() this will give the lambda logger linked with the context object. |
Now, let us update the code given above and observe the output for some of the methods listed above. Observe the Example code given below for a better understanding −
package com.amazonaws.lambda.demo; import com.amazonaws.services.lambda.runtime.Context; import com.amazonaws.services.lambda.runtime.RequestHandler; public class LambdaFunctionHandler implements RequestHandler<Object, String> { @Override public String handleRequest(Object input, Context context) { context.getLogger().log("Input: " + input); System.out.println("AWS Lambda function name: " + context.getFunctionName()); System.out.println("Memory Allocated: " + context.getMemoryLimitInMB()); System.out.println("Time remaining in milliseconds: " + context.getRemainingTimeInMillis()); System.out.println("Cloudwatch group name " + context.getLogGroupName()); System.out.println("AWS Lambda Request Id " + context.getAwsRequestId()); // TODO: implement your handler return "Hello from Lambda!"; } }
Once you run the code given above, you can find the output as given below −
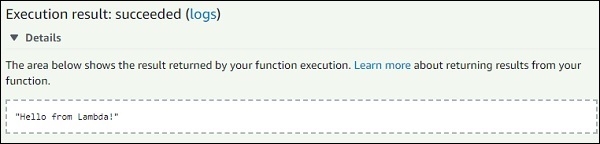
Logs for context
You can observe the following output when you are viewing your log output −
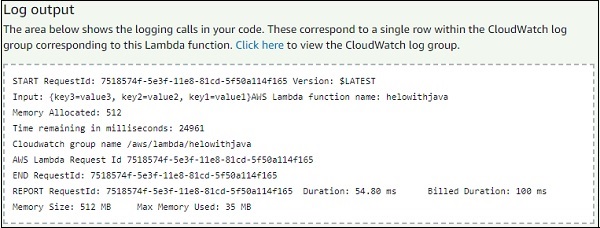
The memory allocated for the Lambda function is 512MB. The time allocated is 25 seconds. The time remaining as displayed above is 24961, which is in milliseconds. So 25000 - 24961 which equals to 39 milliseconds is used for the execution of the Lambda function. Note that Cloudwatch group name and request id are also displayed as shown above.
Note that we have used the following command to print logs in Java −
System.out.println (“log message”)
The same is available in CloudWatch. For this, go to AWS services, select CloudWatchservices and click Logs.
Now, if you select the Lambda function, it will display the logs date wise as shown below −
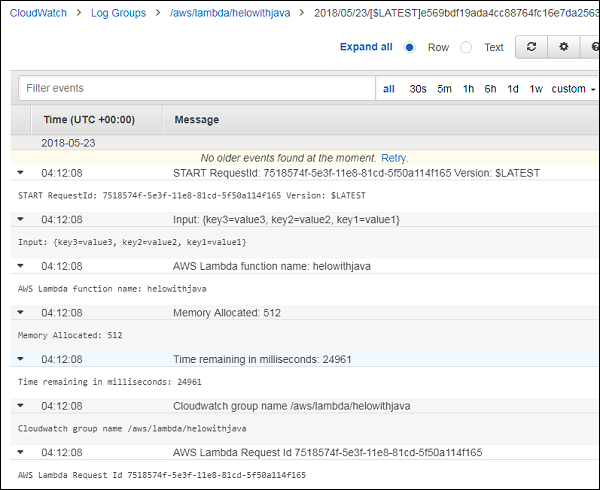
Logging in Java
You can also use Lambdalogger in Java to log the data. Observe the following example that shows the same −
Example
package com.amazonaws.lambda.demo; import com.amazonaws.services.lambda.runtime.Context; import com.amazonaws.services.lambda.runtime.RequestHandler; import com.amazonaws.services.lambda.runtime.LambdaLogger; public class LambdaFunctionHandler implements RequestHandler<Object, String> { @Override public String handleRequest(Object input, Context context) { LambdaLogger logger = context.getLogger(); logger.log("Input: " + input); logger.log("AWS Lambda function name: " + context.getFunctionName()+"\n"); logger.log("Memory Allocated: " + context.getMemoryLimitInMB()+"\n"); logger.log("Time remaining in milliseconds: " + context.getRemainingTimeInMillis()+"\n"); logger.log("Cloudwatch group name " + context.getLogGroupName()+"\n"); logger.log("AWS Lambda Request Id " + context.getAwsRequestId()+"\n"); // TODO: implement your handler return "Hello from Lambda!"; } }
The code shown above will give you the following output −
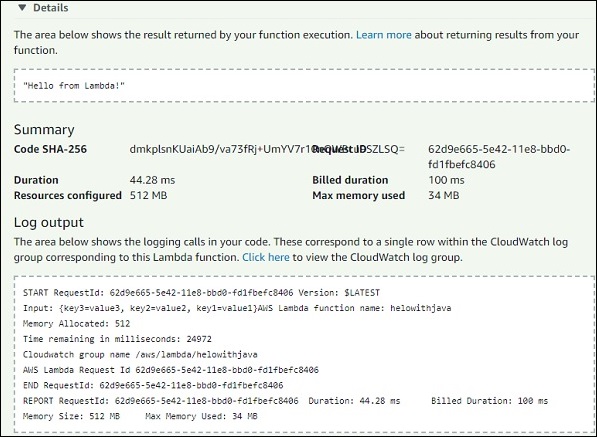
The output in CloudWatch will be as shown below −
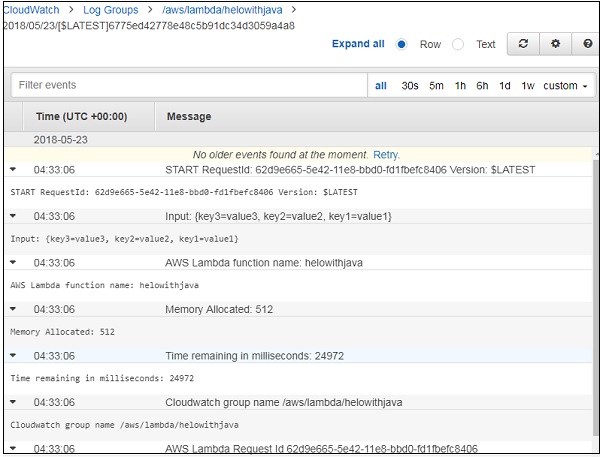
Error handling in Java for Lambda Function
This section will explain how to handle errors in Java for Lambda function. Observe the following code that shows the same −
package com.amazonaws.lambda.errorhandling; import com.amazonaws.services.lambda.runtime.Context; import com.amazonaws.services.lambda.runtime.RequestHandler; public class LambdaFunctionHandler implements RequestHandler<Object, String> { @Override public String handleRequest(Object input, Context context) { throw new RuntimeException("Error from aws lambda"); } }
Note that the error details are displayed in json format with errorMessage Error from AWS Lambda. Also, the ErrorType and stackTrace gives more details about the error.
The output and the corresponding log output of the code given above will be as shown in the following screenshots given below −
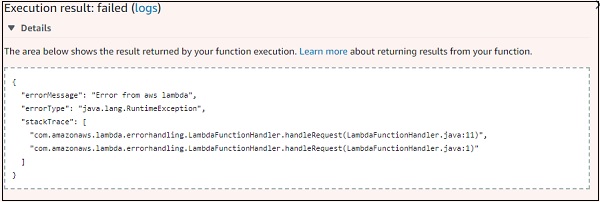
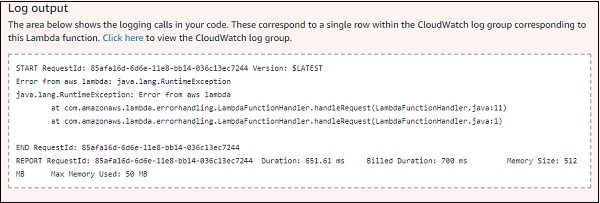