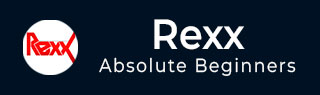
- Rexx Tutorial
- Rexx - Home
- Rexx - Overview
- Rexx - Environment
- Rexx - Installation
- Rexx - Installation of Plugin-Ins
- Rexx - Basic Syntax
- Rexx - Datatypes
- Rexx - Variables
- Rexx - Operators
- Rexx - Arrays
- Rexx - Loops
- Rexx - Decision Making
- Rexx - Numbers
- Rexx - Strings
- Rexx - Functions
- Rexx - Stacks
- Rexx - File I/O
- Rexx - Functions For Files
- Rexx - Subroutines
- Rexx - Built-In Functions
- Rexx - System Commands
- Rexx - XML
- Rexx - Regina
- Rexx - Parsing
- Rexx - Signals
- Rexx - Debugging
- Rexx - Error Handling
- Rexx - Object Oriented
- Rexx - Portability
- Rexx - Extended Functions
- Rexx - Instructions
- Rexx - Implementations
- Rexx - Netrexx
- Rexx - Brexx
- Rexx - Databases
- Handheld & Embedded
- Rexx - Performance
- Rexx - Best Programming Practices
- Rexx - Graphical User Interface
- Rexx - Reginald
- Rexx - Web Programming
- Rexx Useful Resources
- Rexx - Quick Guide
- Rexx - Useful Resources
- Rexx - Discussion
Rexx - File I/O
Rexx provides a number of methods when working with I/O. Rexx provides easier classes to provide the following functionalities for files.
- Reading files
- Writing to files
- Seeing whether a file is a file or directory
The functions available in Rexx for File I/O are based on both line input and character input and we will be looking at the functions available for both in detail.
Let’s explore some of the file operations Rexx has to offer. For the purposes of these examples, we are going to assume that there is a file called NewFile.txt which contains the following lines of text −
Example1
Example2
Example3
This file will be used for the read and write operations in the following examples. Here we will discuss regarding how to read the contents on a file in different ways.
Reading the Contents of a File a Line at a Time
The general operations on files are carried out by using the methods available in the Rexx library itself. The reading of files is the simplest of all operations in Rexx.
Let’s look at the function used to accomplish this.
linein
This method returns a line from the text file. The text file is the filename provided as the input parameter to the function.
Syntax −
linein(filename)
Parameter −
filename − This is the name of the file from where the line needs to be read.
Return Value − This method returns one line of the file at a time.
Example −
/* Main program */ line_str = linein(Example.txt) say line_str
The above code is pretty simple in the fact that the Example.txt file name is provided to the linein function. This function then reads a line of text and provides the result to the variable line_str.
Output − When we run the above program we will get the following result.
Example1
Reading the Contents of a File at One Time
In Rexx, reading all the contents of a file can be achieved with the help of the while statement. The while statement will read each line, one by one till the end of the file is reached.
An example on how this can be achieved is shown below.
/* Main program */ do while lines(Example.txt) > 0 line_str = linein(Example.txt) say line_str end
In the above program, the following things need to be noted −
The lines function reads the Example.txt file.
The while function is used to check if further lines exist in the Example.txt file.
For each line read from the file, the line_str variable holds the value of the current line. This is then sent to the console as output.
Output − When we run the above program we will get the following result.
Example1 Example2 Example3
Writing Contents to a File
Just like reading of files, Rexx also has the ability to write to files. Let’s look at the function which is used to accomplish this.
lineout
This method writes a line to a file. The file to which the line needs to be written to is provided as the parameter to the lineout statement.
Syntax −
lineout(filename)
Parameter −
filename − This is the name of the file from where the line needs to be written to.
Return Value − This method returns the status of the lineout function. The value returned is 0 if the line was successfully written else the value of 1 will be returned.
Example −
/* Main program */ out = lineout(Example.txt,"Example4")
Output − Whenever the above code is run, the line “Example4” will be written to the file Example.txt.