
- React Native Tutorial
- React Native - Home
- Core Concepts
- React Native - Overview
- React Native - Environment Setup
- React Native - App
- React Native - State
- React Native - Props
- React Native - Styling
- React Native - Flexbox
- React Native - ListView
- React Native - Text Input
- React Native - ScrollView
- React Native - Images
- React Native - HTTP
- React Native - Buttons
- React Native - Animations
- React Native - Debugging
- React Native - Router
- React Native - Running IOS
- React Native - Running Android
- Components and APIs
- React Native - View
- React Native - WebView
- React Native - Modal
- React Native - ActivityIndicator
- React Native - Picker
- React Native - Status Bar
- React Native - Switch
- React Native - Text
- React Native - Alert
- React Native - Geolocation
- React Native - AsyncStorage
- React Native Useful Resources
- React Native - Quick Guide
- React Native - Useful Resources
- React Native - Discussion
React Native - HTTP
In this chapter, we will show you how to use fetch for handling network requests.
App.js
import React from 'react'; import HttpExample from './http_example.js' const App = () => { return ( <HttpExample /> ) } export default App
Using Fetch
We will use the componentDidMount lifecycle method to load the data from server as soon as the component is mounted. This function will send GET request to the server, return JSON data, log output to console and update our state.
http_example.js
import React, { Component } from 'react' import { View, Text } from 'react-native' class HttpExample extends Component { state = { data: '' } componentDidMount = () => { fetch('https://jsonplaceholder.typicode.com/posts/1', { method: 'GET' }) .then((response) => response.json()) .then((responseJson) => { console.log(responseJson); this.setState({ data: responseJson }) }) .catch((error) => { console.error(error); }); } render() { return ( <View> <Text> {this.state.data.body} </Text> </View> ) } } export default HttpExample
Output
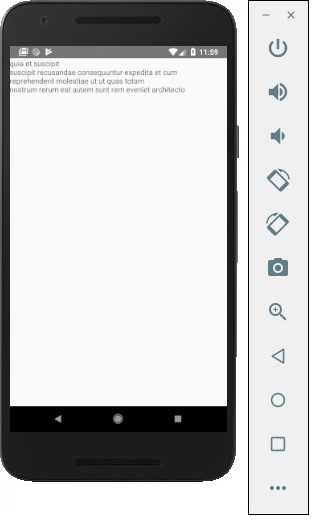
Advertisements