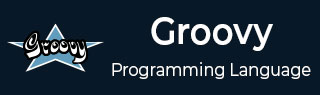
- Groovy Tutorial
- Groovy - Home
- Groovy - Overview
- Groovy - Environment
- Groovy - Basic Syntax
- Groovy - Data Types
- Groovy - Variables
- Groovy - Operators
- Groovy - Loops
- Groovy - Decision Making
- Groovy - Methods
- Groovy - File I/O
- Groovy - Optionals
- Groovy - Numbers
- Groovy - Strings
- Groovy - Ranges
- Groovy - Lists
- Groovy - Maps
- Groovy - Dates & Times
- Groovy - Regular Expressions
- Groovy - Exception Handling
- Groovy - Object Oriented
- Groovy - Generics
- Groovy - Traits
- Groovy - Closures
- Groovy - Annotations
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSLS
- Groovy - Database
- Groovy - Builders
- Groovy - Command Line
- Groovy - Unit Testing
- Groovy - Template Engines
- Groovy - Meta Object Programming
- Groovy Useful Resources
- Groovy - Quick Guide
- Groovy - Useful Resources
- Groovy - Discussion
Groovy - JMX
JMX is the defacto standard which is used for monitoring all applications which have anything to do with the Java virual environment. Given that Groovy sits directly on top of Java, Groovy can leverage the tremendous amount of work already done for JMX with Java.
Monitoring the JVM
One can use the standard classes available in java.lang.management for carrying out the monitoring of the JVM. The following code example shows how this can be done.
import java.lang.management.* def os = ManagementFactory.operatingSystemMXBean println """OPERATING SYSTEM: \tOS architecture = $os.arch \tOS name = $os.name \tOS version = $os.version \tOS processors = $os.availableProcessors """ def rt = ManagementFactory.runtimeMXBean println """RUNTIME: \tRuntime name = $rt.name \tRuntime spec name = $rt.specName \tRuntime vendor = $rt.specVendor \tRuntime spec version = $rt.specVersion \tRuntime management spec version = $rt.managementSpecVersion """ def mem = ManagementFactory.memoryMXBean def heapUsage = mem.heapMemoryUsage def nonHeapUsage = mem.nonHeapMemoryUsage println """MEMORY: HEAP STORAGE: \tMemory committed = $heapUsage.committed \tMemory init = $heapUsage.init \tMemory max = $heapUsage.max \tMemory used = $heapUsage.used NON-HEAP STORAGE: \tNon-heap memory committed = $nonHeapUsage.committed \tNon-heap memory init = $nonHeapUsage.init \tNon-heap memory max = $nonHeapUsage.max \tNon-heap memory used = $nonHeapUsage.used """ println "GARBAGE COLLECTION:" ManagementFactory.garbageCollectorMXBeans.each { gc -> println "\tname = $gc.name" println "\t\tcollection count = $gc.collectionCount" println "\t\tcollection time = $gc.collectionTime" String[] mpoolNames = gc.memoryPoolNames mpoolNames.each { mpoolName -> println "\t\tmpool name = $mpoolName" } }
When the code is executed, the output will vary depending on the system on which the code is run. A sample of the output is given below.
OPERATING SYSTEM: OS architecture = x86 OS name = Windows 7 OS version = 6.1 OS processors = 4 RUNTIME: Runtime name = 5144@Babuli-PC Runtime spec name = Java Virtual Machine Specification Runtime vendor = Oracle Corporation Runtime spec version = 1.7 Runtime management spec version = 1.2 MEMORY: HEAP STORAGE: Memory committed = 16252928 Memory init = 16777216 Memory max = 259522560 Memory used = 7355840 NON-HEAP STORAGE: Non-heap memory committed = 37715968 Non-heap memory init = 35815424 Non-heap memory max = 123731968 Non-heap memory used = 18532232 GARBAGE COLLECTION: name = Copy collection count = 15 collection time = 47 mpool name = Eden Space mpool name = Survivor Space name = MarkSweepCompact collection count = 0 collection time = 0 mpool name = Eden Space mpool name = Survivor Space mpool name = Tenured Gen mpool name = Perm Gen mpool name = Perm Gen [shared-ro] mpool name = Perm Gen [shared-rw]
Monitoring Tomcat
In order to monitor tomcat, the following parameter should be set when tomcat is started −
set JAVA_OPTS = -Dcom.sun.management.jmxremote Dcom.sun.management.jmxremote.port = 9004\ -Dcom.sun.management.jmxremote.authenticate=false Dcom.sun.management.jmxremote.ssl = false
The following code uses JMX to discover the available MBeans in the running Tomcat, determine which are the web modules and extract the processing time for each web module.
import groovy.swing.SwingBuilder import javax.management.ObjectName import javax.management.remote.JMXConnectorFactory as JmxFactory import javax.management.remote.JMXServiceURL as JmxUrl import javax.swing.WindowConstants as WC import org.jfree.chart.ChartFactory import org.jfree.data.category.DefaultCategoryDataset as Dataset import org.jfree.chart.plot.PlotOrientation as Orientation def serverUrl = 'service:jmx:rmi:///jndi/rmi://localhost:9004/jmxrmi' def server = JmxFactory.connect(new JmxUrl(serverUrl)).MBeanServerConnection def serverInfo = new GroovyMBean(server, 'Catalina:type = Server').serverInfo println "Connected to: $serverInfo" def query = new ObjectName('Catalina:*') String[] allNames = server.queryNames(query, null) def modules = allNames.findAll { name -> name.contains('j2eeType=WebModule') }.collect{ new GroovyMBean(server, it) } println "Found ${modules.size()} web modules. Processing ..." def dataset = new Dataset() modules.each { m -> println m.name() dataset.addValue m.processingTime, 0, m.path }