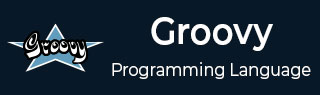
- Groovy Tutorial
- Groovy - Home
- Groovy - Overview
- Groovy - Environment
- Groovy - Basic Syntax
- Groovy - Data Types
- Groovy - Variables
- Groovy - Operators
- Groovy - Loops
- Groovy - Decision Making
- Groovy - Methods
- Groovy - File I/O
- Groovy - Optionals
- Groovy - Numbers
- Groovy - Strings
- Groovy - Ranges
- Groovy - Lists
- Groovy - Maps
- Groovy - Dates & Times
- Groovy - Regular Expressions
- Groovy - Exception Handling
- Groovy - Object Oriented
- Groovy - Generics
- Groovy - Traits
- Groovy - Closures
- Groovy - Annotations
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSLS
- Groovy - Database
- Groovy - Builders
- Groovy - Command Line
- Groovy - Unit Testing
- Groovy - Template Engines
- Groovy - Meta Object Programming
- Groovy Useful Resources
- Groovy - Quick Guide
- Groovy - Useful Resources
- Groovy - Discussion
Groovy - Exception Handling
Exception handling is required in any programming language to handle the runtime errors so that normal flow of the application can be maintained.
Exception normally disrupts the normal flow of the application, which is the reason why we need to use Exception handling in our application.
Exceptions are broadly classified into the following categories −
Checked Exception − The classes that extend Throwable class except RuntimeException and Error are known as checked exceptions e.g.IOException, SQLException etc. Checked exceptions are checked at compile-time.
One classical case is the FileNotFoundException. Suppose you had the following codein your application which reads from a file in E drive.
class Example { static void main(String[] args) { File file = new File("E://file.txt"); FileReader fr = new FileReader(file); } }
if the File (file.txt) is not there in the E drive then the following exception will be raised.
Caught: java.io.FileNotFoundException: E:\file.txt (The system cannot find the file specified).
java.io.FileNotFoundException: E:\file.txt (The system cannot find the file specified).
Unchecked Exception − The classes that extend RuntimeException are known as unchecked exceptions, e.g., ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc. Unchecked exceptions are not checked at compile-time rather they are checked at runtime.
One classical case is the ArrayIndexOutOfBoundsException which happens when you try to access an index of an array which is greater than the length of the array. Following is a typical example of this sort of mistake.
class Example { static void main(String[] args) { def arr = new int[3]; arr[5] = 5; } }
When the above code is executed the following exception will be raised.
Caught: java.lang.ArrayIndexOutOfBoundsException: 5
java.lang.ArrayIndexOutOfBoundsException: 5
Error − Error is irrecoverable e.g. OutOfMemoryError, VirtualMachineError, AssertionError etc.
These are errors which the program can never recover from and will cause the program to crash.
The following diagram shows how the hierarchy of exceptions in Groovy is organized. It’s all based on the hierarchy defined in Java.
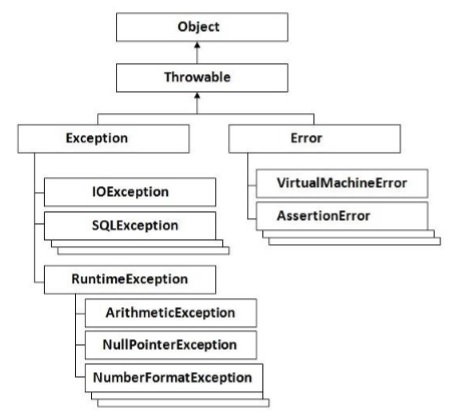
Catching Exceptions
A method catches an exception using a combination of the try and catch keywords. A try/catch block is placed around the code that might generate an exception.
try { //Protected code } catch(ExceptionName e1) { //Catch block }
All of your code which could raise an exception is placed in the Protected code block.
In the catch block, you can write custom code to handle your exception so that the application can recover from the exception.
Let’s look at an example of the similar code we saw above for accessing an array with an index value which is greater than the size of the array. But this time let’s wrap our code in a try/catch block.
class Example { static void main(String[] args) { try { def arr = new int[3]; arr[5] = 5; } catch(Exception ex) { println("Catching the exception"); } println("Let's move on after the exception"); } }
When we run the above program, we will get the following result −
Catching the exception Let's move on after the exception
From the above code, we wrap out faulty code in the try block. In the catch block we are just catching our exception and outputting a message that an exception has occurred.
Multiple Catch Blocks
One can have multiple catch blocks to handle multiple types of exceptions. For each catch block, depending on the type of exception raised you would write code to handle it accordingly.
Let’s modify our above code to catch the ArrayIndexOutOfBoundsException specifically. Following is the code snippet.
class Example { static void main(String[] args) { try { def arr = new int[3]; arr[5] = 5; }catch(ArrayIndexOutOfBoundsException ex) { println("Catching the Array out of Bounds exception"); }catch(Exception ex) { println("Catching the exception"); } println("Let's move on after the exception"); } }
When we run the above program, we will get the following result −
Catching the Aray out of Bounds exception Let's move on after the exception
From the above code you can see that the ArrayIndexOutOfBoundsException catch block is caught first because it means the criteria of the exception.
Finally Block
The finally block follows a try block or a catch block. A finally block of code always executes, irrespective of occurrence of an Exception.
Using a finally block allows you to run any cleanup-type statements that you want to execute, no matter what happens in the protected code. The syntax for this block is given below.
try { //Protected code } catch(ExceptionType1 e1) { //Catch block } catch(ExceptionType2 e2) { //Catch block } catch(ExceptionType3 e3) { //Catch block } finally { //The finally block always executes. }
Let’s modify our above code and add the finally block of code. Following is the code snippet.
class Example { static void main(String[] args) { try { def arr = new int[3]; arr[5] = 5; } catch(ArrayIndexOutOfBoundsException ex) { println("Catching the Array out of Bounds exception"); }catch(Exception ex) { println("Catching the exception"); } finally { println("The final block"); } println("Let's move on after the exception"); } }
When we run the above program, we will get the following result −
Catching the Array out of Bounds exception The final block Let's move on after the exception
Following are the Exception methods available in Groovy −
public String getMessage()
Returns a detailed message about the exception that has occurred. This message is initialized in the Throwable constructor.
public Throwable getCause()
Returns the cause of the exception as represented by a Throwable object.
public String toString()
Returns the name of the class concatenated with the result of getMessage()
public void printStackTrace()
Prints the result of toString() along with the stack trace to System.err, the error output stream.
public StackTraceElement [] getStackTrace()
Returns an array containing each element on the stack trace. The element at index 0 represents the top of the call stack, and the last element in the array represents the method at the bottom of the call stack.
public Throwable fillInStackTrace()
Fills the stack trace of this Throwable object with the current stack trace, adding to any previous information in the stack trace.
Example
Following is the code example using some of the methods given above −
class Example { static void main(String[] args) { try { def arr = new int[3]; arr[5] = 5; }catch(ArrayIndexOutOfBoundsException ex) { println(ex.toString()); println(ex.getMessage()); println(ex.getStackTrace()); } catch(Exception ex) { println("Catching the exception"); }finally { println("The final block"); } println("Let's move on after the exception"); } }
When we run the above program, we will get the following result −
java.lang.ArrayIndexOutOfBoundsException: 5 5 [org.codehaus.groovy.runtime.dgmimpl.arrays.IntegerArrayPutAtMetaMethod$MyPojoMetaMet hodSite.call(IntegerArrayPutAtMetaMethod.java:75), org.codehaus.groovy.runtime.callsite.CallSiteArray.defaultCall(CallSiteArray.java:48) , org.codehaus.groovy.runtime.callsite.AbstractCallSite.call(AbstractCallSite.java:113) , org.codehaus.groovy.runtime.callsite.AbstractCallSite.call(AbstractCallSite.java:133) , Example.main(Sample:8), sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method), sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57), sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) , java.lang.reflect.Method.invoke(Method.java:606), org.codehaus.groovy.reflection.CachedMethod.invoke(CachedMethod.java:93), groovy.lang.MetaMethod.doMethodInvoke(MetaMethod.java:325), groovy.lang.MetaClassImpl.invokeStaticMethod(MetaClassImpl.java:1443), org.codehaus.groovy.runtime.InvokerHelper.invokeMethod(InvokerHelper.java:893), groovy.lang.GroovyShell.runScriptOrMainOrTestOrRunnable(GroovyShell.java:287), groovy.lang.GroovyShell.run(GroovyShell.java:524), groovy.lang.GroovyShell.run(GroovyShell.java:513), groovy.ui.GroovyMain.processOnce(GroovyMain.java:652), groovy.ui.GroovyMain.run(GroovyMain.java:384), groovy.ui.GroovyMain.process(GroovyMain.java:370), groovy.ui.GroovyMain.processArgs(GroovyMain.java:129), groovy.ui.GroovyMain.main(GroovyMain.java:109), sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method), sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57), sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) , java.lang.reflect.Method.invoke(Method.java:606), org.codehaus.groovy.tools.GroovyStarter.rootLoader(GroovyStarter.java:109), org.codehaus.groovy.tools.GroovyStarter.main(GroovyStarter.java:131), sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method), sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57), sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) , java.lang.reflect.Method.invoke(Method.java:606), com.intellij.rt.execution.application.AppMain.main(AppMain.java:144)] The final block Let's move on after the exception