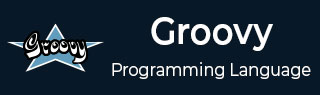
- Groovy Tutorial
- Groovy - Home
- Groovy - Overview
- Groovy - Environment
- Groovy - Basic Syntax
- Groovy - Data Types
- Groovy - Variables
- Groovy - Operators
- Groovy - Loops
- Groovy - Decision Making
- Groovy - Methods
- Groovy - File I/O
- Groovy - Optionals
- Groovy - Numbers
- Groovy - Strings
- Groovy - Ranges
- Groovy - Lists
- Groovy - Maps
- Groovy - Dates & Times
- Groovy - Regular Expressions
- Groovy - Exception Handling
- Groovy - Object Oriented
- Groovy - Generics
- Groovy - Traits
- Groovy - Closures
- Groovy - Annotations
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSLS
- Groovy - Database
- Groovy - Builders
- Groovy - Command Line
- Groovy - Unit Testing
- Groovy - Template Engines
- Groovy - Meta Object Programming
- Groovy Useful Resources
- Groovy - Quick Guide
- Groovy - Useful Resources
- Groovy - Discussion
Groovy - Dates & Times
The class Date represents a specific instant in time, with millisecond precision. The Date class has two constructors as shown below.
Date()
Syntax
public Date()
Parameters − None.
Return Value
Allocates a Date object and initializes it so that it represents the time at which it was allocated, measured to the nearest millisecond.
Example
Following is an example of the usage of this method −
class Example { static void main(String[] args) { Date date = new Date(); // display time and date using toString() System.out.println(date.toString()); } }
When we run the above program, we will get the following result. The following output will give you the current date and time −
Thu Dec 10 21:31:15 GST 2015
Date (long millisec)
Syntax
public Date(long millisec)
Parameters
Millisec – The number of millisecconds to specify since the standard base time.
Return Value − Allocates a Date object and initializes it to represent the specified number of milliseconds since the standard base time known as "the epoch", namely January 1, 1970, 00:00:00 GMT.
Example
Following is an example of the usage of this method −
class Example { static void main(String[] args) { Date date = new Date(100); // display time and date using toString() System.out.println(date.toString()); } }
When we run the above program, we will get the following result −
Thu Jan 01 04:00:00 GST 1970
Following are the given methods of the Date class. In all methods of class Date that accept or return year, month, date, hours, minutes, and seconds values, the following representations are used −
A year y is represented by the integer y - 1900.
A month is represented by an integer from 0 to 11; 0 is January, 1 is February, and so forth; thus 11 is December.
A date (day of month) is represented by an integer from 1 to 31 in the usual manner.
An hour is represented by an integer from 0 to 23. Thus, the hour from midnight to 1 a.m. is hour 0, and the hour from noon to 1 p.m. is hour 12.
A minute is represented by an integer from 0 to 59 in the usual manner.
A second is represented by an integer from 0 to 61.
Sr.No. | Methods & Description |
---|---|
1 | after()
Tests if this date is after the specified date. |
2 | equals()
Compares two dates for equality. The result is true if and only if the argument is not null and is a Date object that represents the same point in time, to the millisecond, as this object. |
3 | compareTo()
Compares two Dates for ordering. |
4 | toString()
Converts this Date object to a String |
5 | before()
Tests if this date is before the specified date. |
6 | getTime()
Returns the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object. |
7 | setTime()
Sets this Date object to represent a point in time that is time milliseconds after January 1, 1970 00:00:00 GMT. |
To Continue Learning Please Login