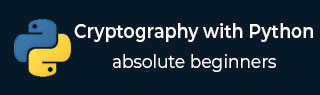
- Cryptography with Python Tutorial
- Home
- Overview
- Double Strength Encryption
- Python Overview and Installation
- Reverse Cipher
- Caesar Cipher
- ROT13 Algorithm
- Transposition Cipher
- Encryption of Transposition Cipher
- Decryption of Transposition Cipher
- Encryption of files
- Decryption of files
- Base64 Encoding & Decoding
- XOR Process
- Multiplicative Cipher
- Affine Ciphers
- Hacking Monoalphabetic Cipher
- Simple Substitution Cipher
- Testing of Simple Substitution Cipher
- Decryption of Simple Substitution Cipher
- Python Modules of Cryptography
- Understanding Vignere Cipher
- Implementing Vignere Cipher
- One Time Pad Cipher
- Implementation of One Time Pad Cipher
- Symmetric & Asymmetric Cryptography
- Understanding RSA Algorithm
- Creating RSA Keys
- RSA Cipher Encryption
- RSA Cipher Decryption
- Hacking RSA Cipher
- Useful Resources
- Quick Guide
- Resources
- Discussions
RSA Cipher Decryption
This chapter is a continuation of the previous chapter where we followed step wise implementation of encryption using RSA algorithm and discusses in detail about it.
The function used to decrypt cipher text is as follows −
def decrypt(ciphertext, priv_key): cipher = PKCS1_OAEP.new(priv_key) return cipher.decrypt(ciphertext)
For public key cryptography or asymmetric key cryptography, it is important to maintain two important features namely Authentication and Authorization.
Authorization
Authorization is the process to confirm that the sender is the only one who have transmitted the message. The following code explains this −
def sign(message, priv_key, hashAlg="SHA-256"): global hash hash = hashAlg signer = PKCS1_v1_5.new(priv_key) if (hash == "SHA-512"): digest = SHA512.new() elif (hash == "SHA-384"): digest = SHA384.new() elif (hash == "SHA-256"): digest = SHA256.new() elif (hash == "SHA-1"): digest = SHA.new() else: digest = MD5.new() digest.update(message) return signer.sign(digest)
Authentication
Authentication is possible by verification method which is explained as below −
def verify(message, signature, pub_key): signer = PKCS1_v1_5.new(pub_key) if (hash == "SHA-512"): digest = SHA512.new() elif (hash == "SHA-384"): digest = SHA384.new() elif (hash == "SHA-256"): digest = SHA256.new() elif (hash == "SHA-1"): digest = SHA.new() else: digest = MD5.new() digest.update(message) return signer.verify(digest, signature)
The digital signature is verified along with the details of sender and recipient. This adds more weight age for security purposes.
RSA Cipher Decryption
You can use the following code for RSA cipher decryption −
from Crypto.PublicKey import RSA from Crypto.Cipher import PKCS1_OAEP from Crypto.Signature import PKCS1_v1_5 from Crypto.Hash import SHA512, SHA384, SHA256, SHA, MD5 from Crypto import Random from base64 import b64encode, b64decode hash = "SHA-256" def newkeys(keysize): random_generator = Random.new().read key = RSA.generate(keysize, random_generator) private, public = key, key.publickey() return public, private def importKey(externKey): return RSA.importKey(externKey) def getpublickey(priv_key): return priv_key.publickey() def encrypt(message, pub_key): cipher = PKCS1_OAEP.new(pub_key) return cipher.encrypt(message) def decrypt(ciphertext, priv_key): cipher = PKCS1_OAEP.new(priv_key) return cipher.decrypt(ciphertext) def sign(message, priv_key, hashAlg = "SHA-256"): global hash hash = hashAlg signer = PKCS1_v1_5.new(priv_key) if (hash == "SHA-512"): digest = SHA512.new() elif (hash == "SHA-384"): digest = SHA384.new() elif (hash == "SHA-256"): digest = SHA256.new() elif (hash == "SHA-1"): digest = SHA.new() else: digest = MD5.new() digest.update(message) return signer.sign(digest) def verify(message, signature, pub_key): signer = PKCS1_v1_5.new(pub_key) if (hash == "SHA-512"): digest = SHA512.new() elif (hash == "SHA-384"): digest = SHA384.new() elif (hash == "SHA-256"): digest = SHA256.new() elif (hash == "SHA-1"): digest = SHA.new() else: digest = MD5.new() digest.update(message) return signer.verify(digest, signature)