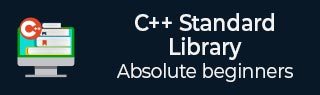
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Library - <bitset>
Introduction
Bitset represents a fixed-size sequence of N bits and stores values either 0 or 1. Zero means value is false or bit is unset and one means value is true or bit is set. Bitset class emulates space efficient array of boolean values, where each element occupies only one bit.
As it emulates array, its index also starts from 0th position. Individual bit from bitset can be accessed using subscript operator. For instance to access first element of bitset foo use foo[0].
Bitset class provides constructors to create bitset from integer as well as from strings. The size of the bitset is fixed at compile time. STL provides vector<bool> class that provides dynamic resize functionality.
Definition
Below is definition of std::bitset from <bitset> header file
template <size_t N> class bitset;
Parameters
N − Size of the bitset.
Member types
Following member types can be used as parameters or return type by member functions.
Sr.No. | Member types | Definition |
---|---|---|
1 | reference | Proxy class that represents a reference to a bit. |
Functions from <bitset>
Below is list of all methods from <bitset> header.
Constructors
Sr.No. | Method & Description |
---|---|
1 | bitset::bitset()
Constructs bitset container and initialize it with zero. |
2 | bitset::bitset()
Constructs bitset container and initialize it with the bit value of val. |
3 | bitset::bitset()
Constructs and initializes a bitset container from C++ string object. |
4 | bitset::bitset()
Constructs and initializes a bitset container from c-style string. |
Member class
Sr.No. | Method & Description |
---|---|
1 | bitset::reference()
This is embedded class which provides l-value that can be returned from std::bitset::operator[]. |
Bitset operators
Sr.No. | Method & Description |
---|---|
1 | bitset::operator&=
Performs bitwise AND operation on current bitset object. |
2 | bitset::operator|=
Performs bitwise OR operation on current bitset object. |
3 | bitset::operator^=
Performs bitwise XOR operation on current bitset object. |
4 | bitset::operator<<=
Performs bitwise left SHIFT operation on current bitset object. |
5 | bitset::operator>>=
Performs bitwise right SHIFT operation on current bitset object. |
6 | bitset::operator~
Performs bitwise NOT operation on bitset. |
7 | bitset::operator<<
Performs bitwise left SHIFT operation on bitset. |
8 | bitset::operator>>
Performs bitwise right SHIFT operation on bitset. |
9 | bitset::operator==
Test whether two bitsets are equal or not. |
10 | bitset::operator!=
Test whether two bitsets are equal or not. |
11 | bitset::operator&
Performs bitwise AND operation on bitset. |
12 | bitset::operator|
Performs bitwise OR operation on bitset. |
13 | bitset::operator^
Performs bitwise XOR operation on bitset. |
14 | bitset::operator>>
Extracts upto N bits from is and stores into another bitset x. |
15 | bitset::operator>>
Inserts bitset x to the character stream os. |
Member functions
Sr.No. | Method & Description |
---|---|
1 | bitset::all()
Tests whether all bits from bitset are set or not. |
2 | bitset::any()
Tests whether at least one bit from bitset is set or not. |
3 | bitset::count()
Count number of set bits from bitset. |
4 | bitset::flip() all bits
Toggles all bits from bitset. |
5 | bitset::flip() single bit
Toggles single bit from bitset. |
6 | bitset::none()
Tests whether all bits are unset or not. |
7 | bitset::operator[] bool version
Returns the value of bit at position pos. |
8 | bitset::operator[] reference version
Returns the reference of bit at position pos. |
9 | bitset::reset() all bits
Reset all bits of bitset to zero. |
10 | bitset::reset() single bit
Reset single bit of bitset to zero. |
11 | bitset::set() all bits
Set all bits from bitset to one. |
12 | bitset::set() single bit Set single bit from bitset to either one or zero. |
13 | bitset::size()
Reports the size of the bitset. |
14 | bitset::test()
Tests whether Nth bit is set or not. |
15 | bitset::to_string()
Converts bitset object to string object. |
16 | bitset::to_ullong()
Convert bitset to unsigned long long. |
17 | bitset::to_ulong()
Convert bitset to unsigned long. |
Non-member functions
Sr.No. | Method & Description |
---|---|
1 | bitset::hash()
Returns hash value based on the provided bitset. |