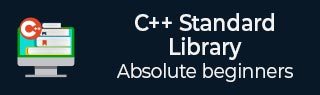
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Bitset Library - operator== Function
Description
The C++ function std::bitset::operator== test whether two bitsets are equal or not.
Declaration
Following is the declaration for std::bitset::operator== function form std::bitset header.
C++98
bool operator== (const bitset& other) const;
C++11
bool operator== (const bitset& other) const noexcept;
Parameters
other − Another bitset object.
Return value
Returns true if both bitsets are equal otherwise false.
Exceptions
This member function never throws exception.
Example
The following example shows the usage of std::bitset::operator== function.
#include <iostream> #include <bitset> using namespace std; int main(void) { bitset<4> b1("1010"); bitset<4> b2("1010"); if (b1 == b2) cout << "Both bitsets are equal." << endl; b1 >>= 1; if (!(b1 == b2)) cout << "Both bitsets are not equal." << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
Both bitsets are equal. Both bitsets are not equal.
bitset.htm
Advertisements