
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10877 Articles for Web Development

2K+ Views
The ! symbol shows that it is an immediately-invoked function expression.The exclamation mark won’t invoke the function alone; you can put () at the end −!function foo() {}()() has higher precedence than ! and instantly calls the function.You can also mention it like the following −(function(){})();The ! allows the expression to return true. This is because by default all immediately-invoked function expression return undefined, so, we’re left with ! undefined, which is true.

17K+ Views
In this tutorial, we will learn how to add a number of days to a JavaScript Date object. Here we will discuss two methods which are following. Using the setDate( ) Method Using the getTime() Method Using the setDate( ) Method JavaScript date setDate() method sets the day of the month for a specified date according to local time. Syntax Its syntax is as follows − Date.setDate( dayValue ) Here dayValue is an integer from 1 to 31, representing the day of the month. Approach To add a number of days to the current date, first we ... Read More

507 Views
To use a variable number of arguments to function, use the arguments object.ExampleYou can try to run the following code to implement arguments to a function in JavaScriptLive Demo function functionArgument(val1, val2, val3) { var res = ""; res += "Expected Arguments: " + functionArgument.length; res += ""; res += "Current Arguments : " + arguments.length; res += ""; res += "Each argument = " for (p = 0; p < arguments.length; p++) { res += ""; res += functionArgument.arguments[p]; res += " "; } document.write(res); } functionArgument(20, 50, 80, "Demo Text!","Hello World", new Date())

150 Views
ExampleLive Demo $("a").click(function() { $("html, body").animate({ scrollTop: 0 }, "slow"); return false; }); TOP OF PAGE This is demo text. This is demo text. This is demo text. This is demo text. This is demo text. This is demo text. This is ... Read More
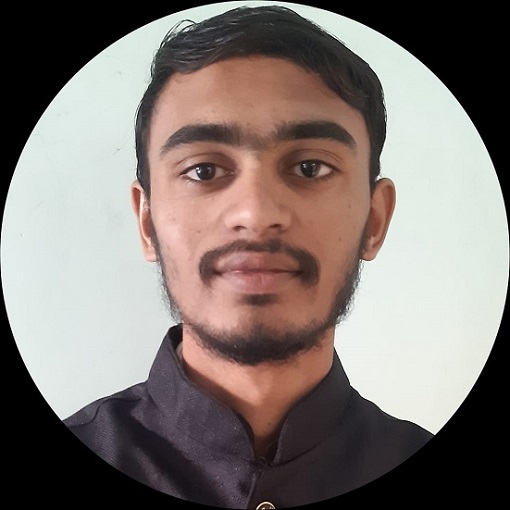
2K+ Views
In this tutorial, we will learn how to use setInterval function call in JavaScript. The setInterval() method in JavaScript evaluates an expression at intervals. It is used to perform the repetitive tasks or operations that need to be performed in a specific time interval. For example, it calls a function repeatedly in a fixed time delay to perform the task. The window interface offers this method. This method returns an interval id that uniquely identifies the interval, allowing you to remove it later by executing the clearInterval() method. Users can follow the below syntax to use the setInterval function. Syntax ... Read More
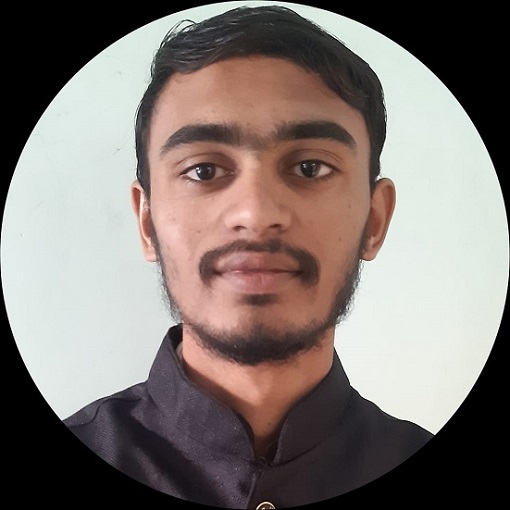
14K+ Views
This tutorial teaches us to format numbers as a dollar currency string in JavaScript. Now, the question arises that why we need to format the numbers as a dollar currency string? Well, the answer is here. Users can think about the scenario where they are developing an eCommerce website or application and must show product prices to the customers. What if they render product prices like 2500 and $2500? The second one looks better as ‘$’ represents the USD currency and is the standardized way to show off the USD currency. Also, second approach is more understandable.Below, we have given ... Read More

308 Views
The JavaScript version of sleep() is “await”. The await feature pauses the current aync function.ExampleYou can try to run the following code to implement sleep in JavaScriptLive Demo function setSleep(ms) { return new Promise(resolve => setTimeout(resolve, ms)); } async function Display() { document.write('Wait for 5 seconds!'); await setSleep(5000); document.write('After 5 seconds!'); } Display();

201 Views
The +function() {} notation is primarily used to force the parser to treat whatever follows the + as an expression. This is used for functions that are invoked immediately, for example,+function() { alert("Demo!"); }();However, + before a function is one of the symbols. You can add other options also like !, -, ~, also. Parentheses can also be used as shown below −(function() { alert("Demo!"); })();You can also use it like this −(function() { alert("Demo!"); }());

681 Views
GetterWhen a property is accessed, the value gets through calling a function implicitly. The get keyword is used in JavaScript. An identifier, either a number or a string is allowed for set.SetterWhen a property is set, it implicitly calls a function and the value is passed as an argument. With that, the return value is set to the property itself. The set keyword is used in JavaScript. An identifier, either a number or a string is allowed for set.ExampleHere’s an example showing how to implement both getter and setterLive Demo ... Read More

133 Views
To delete a setter using the delete operator, use the delete keyword. Here’s how you can delete −delete obj.nameExampleYou can try to run the following code to learn how to delete a setterLive Demo var department = { deptName: "Marketing", deptZone: "North", deptID: 101, get details() { return "Department Details" + "Name: " + this.deptName + " Zone: " ... Read More
To Continue Learning Please Login