
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10878 Articles for Web Development

373 Views
A method in JavaScript is the action performed on objects. A JavaScript method has a function definition, which is stored as a property value. ExampleLet’s see an example to define a method in JavaScriptLive Demo var department = { deptName: "Marketing", deptID : 101, deptZone : "North", details : function() { return "Department Details" + "Name: " + this.deptName + " Zone: " + this.deptZone + "ID: " + this.deptID; } }; document.getElementById("myDept").innerHTML = department.details();

83 Views
The default parameter came to handle function parameters with ease. Default parameters allow you to initialize formal parameters with default values. This is possible only if no value or undefined is passed. With ES6, you can easily set default parameters. Let’s see an exampleExampleLive Demo // default is set to 1 function inc(val1, inc = 1) { return val1 + inc; } document.write(inc(10, 10)); document.write(""); ... Read More

113 Views
To achieve what you want, use JavaScript Closures. A closure is a function, which uses the scope in which it was declared when invoked. It is not the scope in which it was invoked.ExampleLet’s take your example and this is how you can achieve your task. Here, innerDisplay() is a JavaScript closure.Var myFunction = (function () { function display() { // 5 }; function innerDisplay (a) { if (/* some condition */ ) { // 1 // 2 display(); }else { // 3 // 4 display(); } } return innerDisplay; })();
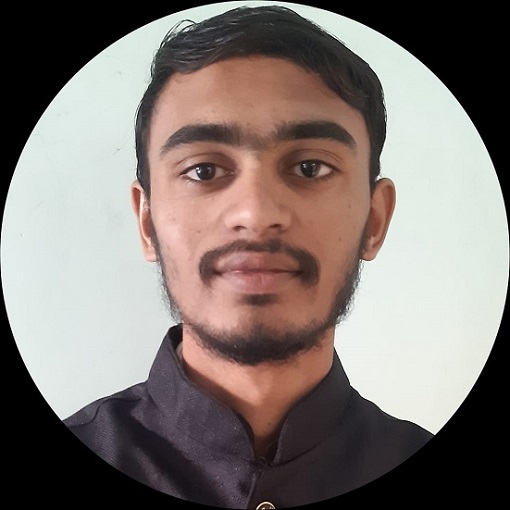
3K+ Views
In this tutorial, we will learn how to pass the value 'undefined' to a function with multiple parameters in JavaScript. In JavaScript, the data type of 'undefined' is primitive. When a variable is declared, JavaScript automatically assigns the value 'undefined' to it. If a function has multiple parameters and one of the parameters' values is not available at the moment, then we need to omit that parameter's value from the function call. But if we omit the parameter's value with a blank space, the JavaScript will show an error. Syntax function abc(param1, param2, param3) { console.log(param1, ... Read More

11K+ Views
We use the join() method of JavaScript to use a line break in array values. It allows us to concatenate all the constituent elements of an array into a single string using a common separator. The join() method in JavaScript The join() method takes as input a single separator string and returns a string with all the elements of the array separated by the specified separator string. The separator string by default is the comma(, ). The join method uses the toString() method to convert the elements of the array into corresponding strings. A null or undefined value is converted ... Read More

1K+ Views
Fat arrow function solves the issue of lexical binding “this”. It gets the context of “this “and you can fulfill the same purpose since fast arrow does not have its own this. Fat arrow function as the name suggests helps in decreasing line of code. The syntax => shows fat arrow.Example$('.button1').click(function () { setTimeout(function () { $(this).text('demo'); } ,400); });The above gives an error since the function() defines this as a global object. Let’s see how to solve it using fat arrow function and the context of “this” −$('.button1').click(function () { setTimeout( () => ... Read More

134 Views
Fat arrow function as the name suggests helps in decreasing line of code. The syntax => shows fat arrow. This also avoids you to write the keyword “function” repeatedly. Arrow functions are generally used for a non-method function. Let’s see how to use arrow functions used as methods:ExampleYou can try to run the following code to implement arrow functions used as methodsLive Demo 'use strict'; var ob1 = { val1: 75, val2: 100, x: () => document.write(this.val1, this), y: function() { document.write(""+this.val1, this); }, z: function() { document.write(""+this.val2, this); }, } ob1.x(); ob1.y(); ob1.z();

494 Views
Fat arrow function as the name suggests helps in decreasing line of code. The syntax => shows fat arrow. This also avoids you to write the keyword “function” repeatedly.Here’s the syntax:argument => expressionUse the following for more than one argument:(argument1 [, argument2]) => expressionLet’s compare a function with and without fat arrow:Function in JavaScriptvar rank = [7,8,9]; var display = rank.map(function(num) { return num * num; });Fat Arrow function in JavaScriptvar rank= [7,8,9]; var display = rank.map((num) => num*num); document.write(arr)Arrow function definitely reduces the code lines.

350 Views
The increment and decrement operators should be avoided since it can lead to unexpected results. Here are some of the conditions:In an assignment statement, it can lead to unfavorable results:ExampleLive Demo var a = 5; var b = ++a; var c = a++; var d = ++c; document.write(a); document.write("\r"+b); document.write("\r"+c); document.write("\r"+d); OutputWhitespace between the operator and variable can also lead to unexpected results:a = b = c = 1; ++a ; b -- ; c;