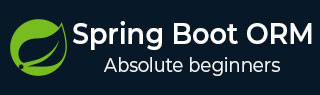
- Spring Boot ORM Tutorial
- Spring Boot ORM - Home
- Spring Boot ORM - Overview
- Environment Setup
- Spring Boot ORM & Spring Data JPA
- Spring Boot ORM - Create Project
- Application.properties
- Spring Boot ORM - Update Project
- Spring Boot ORM - Test Hibernate
- Spring Boot ORM & EclipseLink
- Maven EclipseLink
- Update Project EclipseLink
- Spring Boot ORM - Test EclipseLink
- Spring Boot ORM Useful Resources
- Spring Boot ORM - Quick Guide
- Spring Boot ORM - Useful Resources
- Spring Boot ORM - Discussion
Spring Boot ORM - Update Project EclipseLink
Spring Boot uses HibernateJpaAutoConfiguration which configures the hibernate implementation by default. In order to switch to EclipseLink, we need to create a custom configuration class which will extend the JpaBaseConfiguration class. JpaBaseConfiguration is the base class which is used to extend and configure JPA for any ORM implementation. Following is the code of EclipsLinkJpaConfiguration.
EclipsLinkJpaConfiguration.java
package com.tutorialspoint.springbootorm; import java.util.HashMap; import java.util.Map; import javax.sql.DataSource; import org.eclipse.persistence.config.PersistenceUnitProperties; import org.eclipse.persistence.logging.SessionLog; import org.springframework.beans.factory.ObjectProvider; import org.springframework.boot.autoconfigure.orm.jpa.JpaBaseConfiguration; import org.springframework.boot.autoconfigure.orm.jpa.JpaProperties; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.jdbc.datasource.DriverManagerDataSource; import org.springframework.orm.jpa.vendor.AbstractJpaVendorAdapter; import org.springframework.orm.jpa.vendor.EclipseLinkJpaVendorAdapter; import org.springframework.transaction.jta.JtaTransactionManager; @Configuration public class EclipsLinkJpaConfiguration extends JpaBaseConfiguration { protected EclipsLinkJpaConfiguration(DataSource dataSource, JpaProperties properties, ObjectProvider<JtaTransactionManager> jtaTransactionManager) { super(dataSource, properties, jtaTransactionManager); } @Override protected AbstractJpaVendorAdapter createJpaVendorAdapter() { return new EclipseLinkJpaVendorAdapter(); } @Override protected Map<String, Object> getVendorProperties() { Map<String, Object> map = new HashMap<>(); map.put(PersistenceUnitProperties.WEAVING, "false"); map.put(PersistenceUnitProperties.LOGGING_LEVEL, SessionLog.FINER_LABEL); map.put(PersistenceUnitProperties.DDL_GENERATION, PersistenceUnitProperties.CREATE_ONLY); map.put(PersistenceUnitProperties.LOGGING_LEVEL, SessionLog.FINER_LABEL); return map; } @Bean public static DataSource dataSource() { final DriverManagerDataSource dataSource = new DriverManagerDataSource(); dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver"); dataSource.setUrl("jdbc:mysql://localhost:3306/tutorialspoint?useSSL=false&allowPublicKeyRetrieval=true"); dataSource.setUsername("root"); dataSource.setPassword("root@123"); return dataSource; } }
We've added Adapter, DataSource and properties using createJpaVendorAdapter(), dataSource() and getVendorProperties() methods respectively.
Update the Entity as well to use Integer instead of int.
package com.tutorialspoint.springbootorm.entity; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class Employee { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Integer id; private String name; private Integer age; private String email; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
Advertisements