
- PySimpleGUI Tutorial
- PySimpleGUI - Home
- PySimpleGUI - Introduction
- PySimpleGUI - Environment Setup
- PySimpleGUI - Hello World
- PySimpleGUI - Popup Windows
- PySimpleGUI - Window Class
- PySimpleGUI - Element Class
- PySimpleGUI - Events
- PySimpleGUI - Menubar
- PySimpleGUI - Matplotlib Integration
- PySimpleGUI - Working with PIL
- PySimpleGUI - Debugger
- PySimpleGUI - Settings
- PySimpleGUI Useful Resources
- PySimpleGUI - Quick Guide
- PySimpleGUI - Useful Resources
- PySimpleGUI - Discussion
PySimpleGUI - Tab Element
Sometimes, the application’s GUI design is too big to fit in a single window, and even if we try to arrange all the elements in a layout for the main window, it becomes very clumsy. The use of Tab elements makes the design very convenient, effective and easy for the user to navigate. Tab element is also a container element such as Frame or Column.
First divide the elements in logically relevant groups and put them in separate layouts. Each of them is used to construct a Tab element. These Tab elements are put in a TabGroup as per the following syntax −
# l1 is the layout for tab1 # l2 is the layout for tab2 tab1=PySimpleGUI.Tab("title1", l1) tab2=PySimpleGUI.Tab("title2", l2) Tg = PySimpleGUI.TabGroup([[tab1, tab2]]) # This titlegroup object may be further used # in the row of the main layout.
The advantage of the tabgroup and tab elements is that only one tab of all the tabs in a group is visible at a time. When you click the title of one tab, it becomes visible and all others are hidden. So that, the entire available area can be utilized to display the elements in a single tab. This makes the GUI design very clean and effective.
Remember that the Tab elements are never placed directly in the main layout. They are always contained in a TabGroup.
To construct a Tab element, use the following syntax −
PySimpleGUI.Tab(title, layout, title_color)
Here, the title parameter is a string displayed on the tab. The layout refers to the nested list of elements to be shown on the top and the title_color the color to be used for displaying the title.
In the following example, a typical registration form is designed as a tabgroup with two tabs, one called Basic Info and the other Contact details. Below the tabgroup, two Buttons with OK and Cancel are placed.
import PySimpleGUI as psg psg.set_options(font=("Arial Bold",14)) l1=psg.Text("Enter Name") lt1=psg.Text("Address") t1=psg.Input("", key='-NM-') a11=psg.Input(key='-a11-') a12=psg.Input(key='-a12-') a13=psg.Input(key='-a13-') tab1=[[l1,t1],[lt1],[a11], [a12], [a13]] lt2=psg.Text("EmailID:") lt3=psg.Text("Mob No:") a21=psg.Input("", key='-ID-') a22=psg.Input("", key='-MOB-') tab2=[[lt2, a21], [lt3, a22]] layout = [[psg.TabGroup([ [psg.Tab('Basic Info', tab1), psg.Tab('Contact Details', tab2)]])], [psg.OK(), psg.Cancel()] ] window = psg.Window('Tab Group Example', layout) while True: event, values = window.read() print (event, values) if event in (psg.WIN_CLOSED, 'Exit'): break window.close()
Run the above code. The main window with two tabs is displayed, with the first tab visible by default.
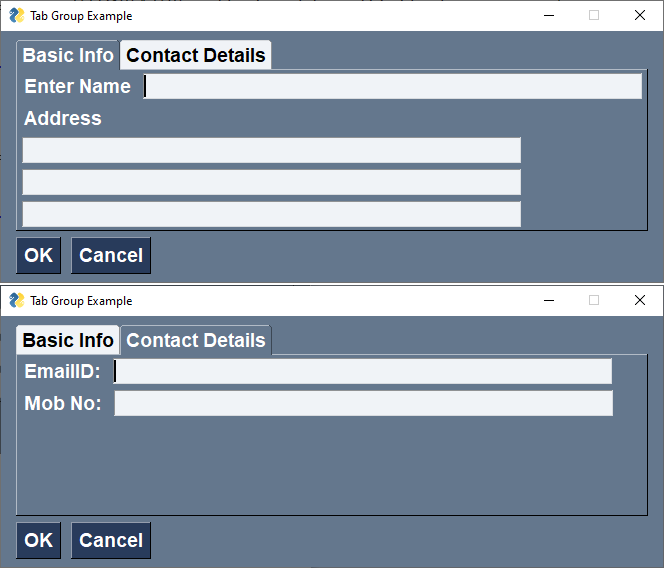
Click the title of the second tab which will show the two Input controls for entering the EmailID and the Mobile number.