
- PySimpleGUI Tutorial
- PySimpleGUI - Home
- PySimpleGUI - Introduction
- PySimpleGUI - Environment Setup
- PySimpleGUI - Hello World
- PySimpleGUI - Popup Windows
- PySimpleGUI - Window Class
- PySimpleGUI - Element Class
- PySimpleGUI - Events
- PySimpleGUI - Menubar
- PySimpleGUI - Matplotlib Integration
- PySimpleGUI - Working with PIL
- PySimpleGUI - Debugger
- PySimpleGUI - Settings
- PySimpleGUI Useful Resources
- PySimpleGUI - Quick Guide
- PySimpleGUI - Useful Resources
- PySimpleGUI - Discussion
PySimpleGUI - Spin Element
The object of Spin class in PysimpleGUI library is also a selection widget. Its visual appearance shows a non-editable text box with up/down buttons to the right side. It is capable of displaying any one item from a list of numbers or strings.
As the up or down button is pressed, the index of the item to be displayed increments or decrements and the next or previous item in the list is displayed in the control’s text box. The displayed value may be used as required in the program logic.
The parameters to the Spin() constructor are −
PySimpleGUI.Spin(values, initial_value, disabled, readonly, size)
Where,
values − List or tuple of valid values - numeric or string
initial_value − Any one item from the supplied list to be initially displayed
disabled − set disable state
readonly − Turns on the Spin element events when up/down button is clicked
size − (w, h) w=characters-wide, h=rows-high
The get() method of the Spin class returns the current item displayed in its text box. On the other hand, the update() method is used to dynamically change following properties of the Spin element −
value − Set the current value from list of choices
values − Set new list object as available choices
The Spin element generates the selection changed event identified by the key parameter when the up/down button is clicked.
In the following example, we construct a simple date selector with the help of three Spin elements - for date, name of month and year between 2000 to 2025. Ranges for date and year elements are numeric whereas for the month spin element, the range is of strings.
import PySimpleGUI as psg import calendar from datetime import datetime dates = [i for i in range(1, 32)] s1 = psg.Spin(dates, initial_value=1, readonly=True, size=3, enable_events=True, key='-DAY-') months = calendar.month_abbr[1:] s2 = psg.Spin(months, initial_value='Jan', readonly=True, size=10, enable_events=True, key='-MON-') yrs = [i for i in range(2000, 2025)] s3 = psg.Spin(yrs, initial_value=2000, readonly=True, size=5, enable_events=True, key='-YR-') layout = [ [psg.Text('Date'), s1, psg.Text("Month"), s2, psg.Text("Year"), s3], [psg.OK(), psg.Text("", key='-OUT-')] ] window = psg.Window('Spin Element Example', layout, font='_ 18', size=(700, 100)) while True: event, values = window.read() if event == 'OK': datestr = str(values['-DAY-']) + " " \ + values['-MON-'] + "," \ + str(values['-YR-']) try: d = datetime.strptime(datestr, '%d %b,%Y') window['-OUT-'].update("Date: {}".format(datestr)) except: window['-OUT-'].update("") psg.Popup("Invalid date") if event == psg.WIN_CLOSED: break window.close()
Set the Spin elements to the desired date value and press OK. If the date string represents a valid date, it is displayed in the Text element in the bottom row.

If the date string is incorrect (for example, 29-Feb-2022), then a popup appears indicating that the value is invalid.
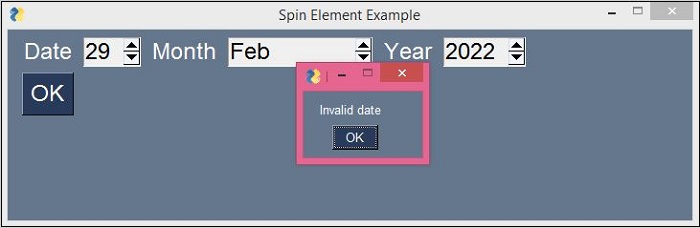