
- PySimpleGUI Tutorial
- PySimpleGUI - Home
- PySimpleGUI - Introduction
- PySimpleGUI - Environment Setup
- PySimpleGUI - Hello World
- PySimpleGUI - Popup Windows
- PySimpleGUI - Window Class
- PySimpleGUI - Element Class
- PySimpleGUI - Events
- PySimpleGUI - Menubar
- PySimpleGUI - Matplotlib Integration
- PySimpleGUI - Working with PIL
- PySimpleGUI - Debugger
- PySimpleGUI - Settings
- PySimpleGUI Useful Resources
- PySimpleGUI - Quick Guide
- PySimpleGUI - Useful Resources
- PySimpleGUI - Discussion
PySimpleGUI - Radio Element
A Radio button is a type of toggle button. Its state changes to True to False and vice versa on every click. A caption appears to the right of a circular clickable region the dot selection indicator in it.
When more than one radio buttons are added as members of a group, they are mutually exclusive, in the sense only one of the buttons can have True state and others become False.
Apart from the common properties inherited from the Element class, the Radio object has following properties important in the context of a Radio button −
text − Text to display next to button
group_id − Groups together multiple Radio Buttons.
default − Set to True for the one element of the group you want initially selected
If the "enable_events" property is set to True for all the buttons having same group_id, the selection changed event is transmitted.
The "get()" method returns True if it is selected, false otherwise. The "update()" method is overridden to modify the properties of the Radio element. These properties are −
value − if True change to selected and set others in group to unselected
text − Text to display next to radio button
disabled − disable or enable state of the element
In the following example, three groups of radio buttons are used. The code computes interest on a loan amount. The interest rate depends on the gender (less by 0.25% for female), period and the type of loan (personal or business - 3% more for business loan) selected by the user.
import PySimpleGUI as psg psg.set_options(font=("Arial Bold", 14)) l1 = psg.Text("Enter amount") l2 = psg.Text("Gender") l3 = psg.Text("Period") l4 = psg.Text("Category") l5 = psg.Text(" ", expand_x=True, key='-OUT-', justification='center') t1 = psg.Input("", key='-AMT-') r11 = psg.Radio("Male", "gen", key='male', default=True) r12 = psg.Radio("Female", "gen", key='female') r21 = psg.Radio("1 Yr", "per", key='one') r22 = psg.Radio("5 Yr", "per", key='five', default=True) r23 = psg.Radio("10 Yr", "per", key='ten') r31 = psg.Radio("Personal", "ctg", key='per', default=True) r32 = psg.Radio("Business", "ctg", key='bus') b1 = psg.Button("OK") b2 = psg.Button("Exit") layout = [[l1, t1], [l2, r11, r12], [l3, r21, r22, r23], [l4, r31, r32], [b1, l5, b2] ] window = psg.Window('Radio button Example', layout, size=(715, 200)) while True: rate = 12 period = 5 event, values = window.read() print(event, values) if event in (psg.WIN_CLOSED, 'Exit'): break if event == 'OK': if values['female'] == True: rate = rate - 0.25 if values['one'] == True: rate = rate + 1 period = 1 if values['ten'] == True: rate = rate - 1 period = 10 if values['bus'] == True: rate = rate + 3 amt = int(values['-AMT-']) print(amt, rate, period) interest = amt * period * rate / 100 window['-OUT-'].update("Interest={}".format(interest)) window.close()
It will produce the following output window −
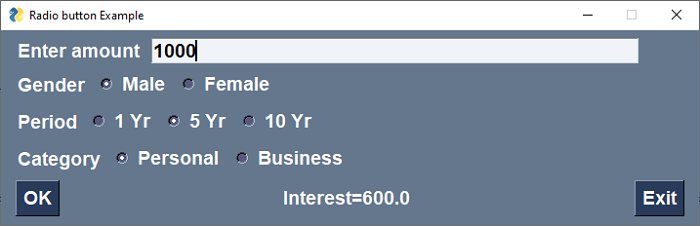