
- PySimpleGUI Tutorial
- PySimpleGUI - Home
- PySimpleGUI - Introduction
- PySimpleGUI - Environment Setup
- PySimpleGUI - Hello World
- PySimpleGUI - Popup Windows
- PySimpleGUI - Window Class
- PySimpleGUI - Element Class
- PySimpleGUI - Events
- PySimpleGUI - Menubar
- PySimpleGUI - Matplotlib Integration
- PySimpleGUI - Working with PIL
- PySimpleGUI - Debugger
- PySimpleGUI - Settings
- PySimpleGUI Useful Resources
- PySimpleGUI - Quick Guide
- PySimpleGUI - Useful Resources
- PySimpleGUI - Discussion
PySimpleGUI - Image Element
The PySimpleGUI library contains an Image element, which has the ability to display images of PNG, GIF, PPM/PGM format. The Image() function needs one mandatory argument which is the path to the image file.
The following code displays the PySimpleGUI logo on the application window.
import PySimpleGUI as psg layout = [[psg.Text(text='Python GUIs for Humans', font=('Arial Bold', 16), size=20, expand_x=True, justification='center')], [psg.Image('PySimpleGUI_Logo.png', expand_x=True, expand_y=True )] ] window = psg.Window('HelloWorld', layout, size=(715,350), keep_on_top=True) while True: event, values = window.read() print(event, values) if event in (None, 'Exit'): break window.close()
It will produce the following output window −
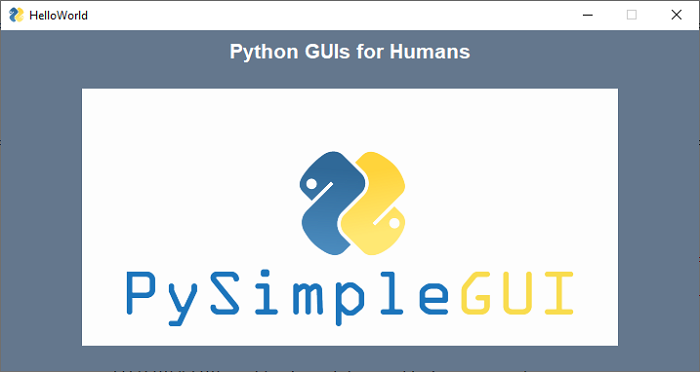
Using Graph Element
You can also display image on a Graph container element with its draw_image() method as the following code shows −
import PySimpleGUI as psg graph = psg.Graph(canvas_size=(700, 300), graph_bottom_left=(0, 0), graph_top_right=(700, 300), background_color='red', enable_events=True, drag_submits=True, key='graph') layout = [ [graph], [psg.Button('LEFT'), psg.Button('RIGHT'), psg.Button('UP'), psg.Button('DOWN')] ] window = psg.Window('Graph test', layout, finalize=True) x1, y1 = 350, 150 id = graph.draw_image(filename="PySimpleGUI_Logo.png", location=(0, 300)) while True: event, values = window.read() if event == psg.WIN_CLOSED: break if event == 'RIGHT': graph.MoveFigure(id, 10, 0) if event == 'LEFT': graph.MoveFigure(id, -10, 0) if event == 'UP': graph.MoveFigure(id, 0, 10) if event == 'DOWN': graph.MoveFigure(id, 0, -10) if event == "graph+UP": x2, y2 = values['graph'] graph.MoveFigure(id, x2 - x1, y2 - y1) x1, y1 = x2, y2 window.close()
It will produce the following output window −
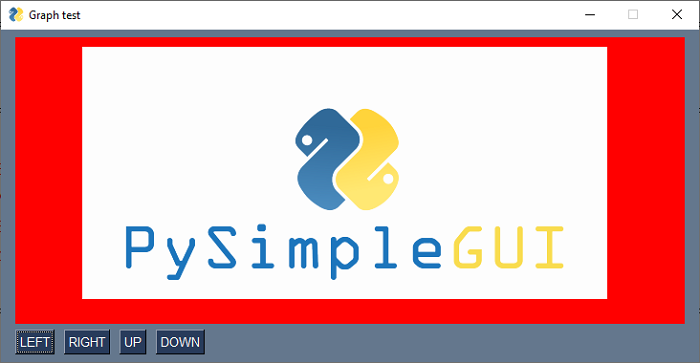
pysimplegui_element_class.htm
Advertisements