
- PySimpleGUI Tutorial
- PySimpleGUI - Home
- PySimpleGUI - Introduction
- PySimpleGUI - Environment Setup
- PySimpleGUI - Hello World
- PySimpleGUI - Popup Windows
- PySimpleGUI - Window Class
- PySimpleGUI - Element Class
- PySimpleGUI - Events
- PySimpleGUI - Menubar
- PySimpleGUI - Matplotlib Integration
- PySimpleGUI - Working with PIL
- PySimpleGUI - Debugger
- PySimpleGUI - Settings
- PySimpleGUI Useful Resources
- PySimpleGUI - Quick Guide
- PySimpleGUI - Useful Resources
- PySimpleGUI - Discussion
PySimpleGUI - Checkbox Element
The Checkbox is also a toggle button having two states: checked and unchecked. It presents a rectangular box which when clicked displays a check mark (or removes it when it already has one) and a caption next to it.
Usually, checkbox controls are provided to let the user select one or more items from the available options. Unlike the Radio button, the Checkboxes on the GUI window do not belong to any group. Hence, user can make multiple selections.
The object of Checkbox class is declared with following specific parameters
PySimpleGUI.Checkbox(text, default, checkbox_color)
These are the properties specific to Checkbox class −
text − This is a string, representing the text to display next to checkbox
default − Set to True if you want this checkbox initially checked
checkbox_color − You can specify the color of background of the box that has the check mark in it.
Apart from these, other common keyword arguments to set the properties inherited from the Element class can be given to the constructor.
The two important methods inherited but overridden in the Checkbox class are −
get() − It return the current state of this checkbox
update() − The Checkbox emits the selection changed event. One or more properties of the Checkbox element are updated in response to an event on the window. These properties are:
value − if True checks the checkbox, False clears it.
text − Text to display next to checkbox
In the following example, a group of three radio buttons represent the faculty streams available in a college. Depending on the faculty chosen, three subjects of that faculty are made available for the user to select one or more from the available options.
import PySimpleGUI as psg psg.set_options(font=("Arial Bold",14)) l1=psg.Text("Enter Name") l2=psg.Text("Faculty") l3=psg.Text("Subjects") l4=psg.Text("Category") l5=psg.Multiline(" ", expand_x=True, key='-OUT-', expand_y=True,justification='left') t1=psg.Input("", key='-NM-') rb=[] rb.append(psg.Radio("Arts", "faculty", key='arts', enable_events=True,default=True)) rb.append(psg.Radio("Commerce", "faculty", key='comm', enable_events=True)) rb.append(psg.Radio("Science", "faculty", key='sci',enable_events=True)) cb=[] cb.append(psg.Checkbox("History", key='s1')) cb.append(psg.Checkbox("Sociology", key='s2')) cb.append(psg.Checkbox("Economics", key='s3')) b1=psg.Button("OK") b2=psg.Button("Exit") layout=[[l1, t1],[rb],[cb],[b1, l5, b2]] window = psg.Window('Checkbox Example', layout, size=(715,250)) while True: event, values = window.read() print (event, values) if event in (psg.WIN_CLOSED, 'Exit'): break if values['comm']==True: window['s1'].update(text="Accounting") window['s2'].update(text="Business Studies") window['s3'].update(text="Statistics") if values['sci']==True: window['s1'].update(text="Physics") window['s2'].update(text="Mathematics") window['s3'].update(text="Biology") if values['arts']==True: window['s1'].update(text="History") window['s2'].update(text="Sociology") window['s3'].update(text="Economics") if event=='OK': subs=[x.Text for x in cb if x.get()==True] fac=[x.Text for x in rb if x.get()==True] out=""" Name={} Faculty: {} Subjects: {} """.format(values['-NM-'], fac[0], " ".join(subs)) window['-OUT-'].update(out) window.close()
Run the above code. Select a faculty name and mark checks in the corresponding check buttons to register the selection. Note that the subjects change as the faculty option is changed.
Press the OK button so that the choices are printed in the Multiline box, as shown below −
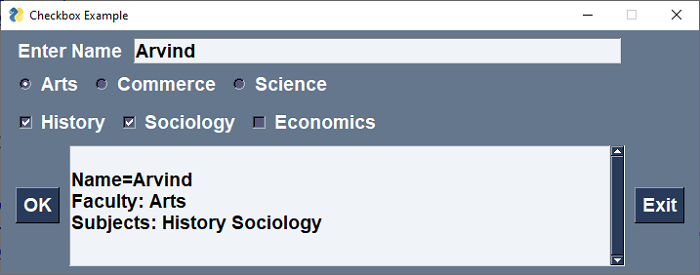