
- PySimpleGUI Tutorial
- PySimpleGUI - Home
- PySimpleGUI - Introduction
- PySimpleGUI - Environment Setup
- PySimpleGUI - Hello World
- PySimpleGUI - Popup Windows
- PySimpleGUI - Window Class
- PySimpleGUI - Element Class
- PySimpleGUI - Events
- PySimpleGUI - Menubar
- PySimpleGUI - Matplotlib Integration
- PySimpleGUI - Working with PIL
- PySimpleGUI - Debugger
- PySimpleGUI - Settings
- PySimpleGUI Useful Resources
- PySimpleGUI - Quick Guide
- PySimpleGUI - Useful Resources
- PySimpleGUI - Discussion
PySimpleGUI - Canvas Element
The Canvas Element provides a drawable panel on the surface of the PySimpleGUI application window. It is equivalent to the Canvas widget in the original TKinter package.
First, declare an object of the Canvas class with following parameters −
can = PySimpleGUI.Canvas(canvas, background_color, size)
Where,
canvas − Leave blank to create a Canvas
background_color − color of background
size − (width in char, height in rows) size in pixels to make canvas
Place this object in the layout for our application window.
We can use the various drawing methods of tkinter’s Canvas by first obtaining the underlying canvas object with TkCanvas property.
tkc = can.TkCanvas
Now we can call the various draw methods as follows −
Sr.No. | Method & Description |
---|---|
1 | create_line Draws a straight line from (x1,y1) to (x2,y2). Color is specified with fill parameter and thickness by width parameter. |
2 | create_rectangle Draws rectangle shape where (x1,y1) denote the coordinates of top left corner and (x2,y2) are coordinates of right bottom corner. The fill parameter is used to display solid rectangle with specified colour. |
3 | create_oval Displays an ellipse. (x1,y1) represents the coordinates of center. r1 and r2 stand for "x" radius and "y" radius. If r1 and r2 same, circle is drawn. |
4 | create_text Displays a string value of text parameter at x1,y1 coordinates. Font parameter decides font name and size and fill parameter is given to apply font colour. |
Given below is a simple implementation of Canvas element −
import PySimpleGUI as sg can=sg.Canvas(size=(700,500), background_color='grey', key='canvas') layout = [[can]] window = sg.Window('Canvas Example', layout, finalize=True) tkc=can.TKCanvas fig = [tkc.create_rectangle(100, 100, 600, 400, outline='white'), tkc.create_line(50, 50, 650, 450, fill='red', width=5), tkc.create_oval(150,150,550,350, fill='blue'), tkc.create_text(350, 250, text="Hello World", fill='white', font=('Arial Bold', 16)), ] while True: event, values = window.read() if event == sg.WIN_CLOSED: break
When the above code is run, you get the following result −
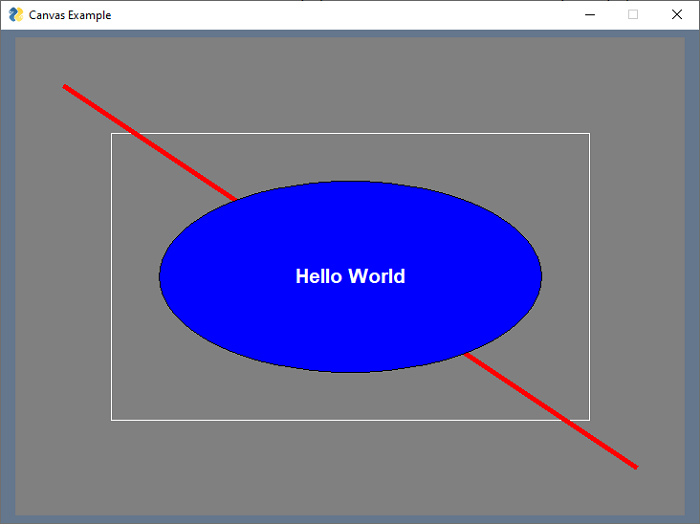