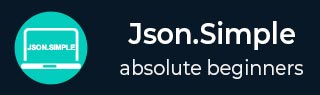
- JSON.simple Tutorial
- JSON.simple - Home
- JSON.simple - Overview
- JSON.simple - Environment Setup
- JSON.simple - JAVA Mapping
- Decoding Examples
- Escaping Special Characters
- JSON.simple - Using JSONValue
- JSON.simple - Exception Handling
- JSON.simple - Container Factory
- JSON.simple - Content Handler
- Encoding Examples
- JSON.simple - Encode JSONObject
- JSON.simple - Encode JSONArray
- Merging Examples
- JSON.simple - Merging Objects
- JSON.simple - Merging Arrays
- Combination Examples
- JSON.simple - Primitive, Object, Array
- JSON.simple - Primitive, Map, List
- Primitive, Object, Map, List
- Customization Examples
- JSON.simple - Customized Output
- Customized Output Streaming
- JSON.simple Useful Resources
- JSON.simple - Quick Guide
- JSON.simple - Useful Resources
- JSON.simple - Discussion
JSON.simple - Encode JSONArray
Using JSON.simple, we can encode a JSON Array using following ways −
Encode a JSON Array - to String − Simple encoding.
Encode a JSON Array - Streaming − Output can be used for streaming.
Encode a JSON Array - Using List − Encoding by using the List.
Encode a JSON Array - Using List and Streaming − Encoding by using List and to stream.
Following example illustrates the above concepts.
Example
import java.io.IOException; import java.io.StringWriter; import java.util.LinkedList; import java.util.List; import org.json.simple.JSONArray; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray list = new JSONArray(); String jsonText; list.add("foo"); list.add(new Integer(100)); list.add(new Double(1000.21)); list.add(new Boolean(true)); list.add(null); jsonText = list.toString(); System.out.println("Encode a JSON Array - to String"); System.out.print(jsonText); StringWriter out = new StringWriter(); list.writeJSONString(out); jsonText = out.toString(); System.out.println("\nEncode a JSON Array - Streaming"); System.out.print(jsonText); List list1 = new LinkedList(); list1.add("foo"); list1.add(new Integer(100)); list1.add(new Double(1000.21)); list1.add(new Boolean(true)); list1.add(null); jsonText = JSONValue.toJSONString(list1); System.out.println("\nEncode a JSON Array - Using List"); System.out.print(jsonText); out = new StringWriter(); JSONValue.writeJSONString(list1, out); jsonText = out.toString(); System.out.println("\nEncode a JSON Array - Using List and Stream"); System.out.print(jsonText); } }
Output
Encode a JSON Array - to String ["foo",100,1000.21,true,null] Encode a JSON Array - Streaming ["foo",100,1000.21,true,null] Encode a JSON Array - Using List ["foo",100,1000.21,true,null] Encode a JSON Array - Using List and Stream ["foo",100,1000.21,true,null]
Advertisements