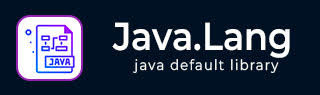
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java.lang.String.replace() Method
Description
The java.lang.String.replace(CharSequence target, CharSequence replacement) method replaces each substring of this string that matches the literal target sequence with the specified literal replacement sequence. The replacement proceeds from the beginning of the string to the end −
Example: replacing "cc" with "b" in the string "ccc" will result in "bc" rather than "cb".
Declaration
Following is the declaration for java.lang.String.replace() method
public String replace(CharSequence target, CharSequence replacement)
Parameters
target − This is the sequence of char values to be replaced.
replacement − This is the replacement sequence of char values.
Return Value
This method returns the resulting string.
Exception
NullPointerException − if target or replacement is null.
Example
The following example shows the usage of java.lang.String.replace() method.
package com.tutorialspoint; import java.lang.*; public class StringDemo { public static void main(String[] args) { String str = "aacdeaa"; System.out.println("string = " + str); CharSequence s1 = "cde"; CharSequence s2 = "ghi"; // replace sequence s1 with s2 String replaceStr = str.replace(s1, s2); // prints the string after replacement System.out.println("new string = " + replaceStr); } }
Let us compile and run the above program, this will produce the following result −
string = aacdeaa new string = aaghiaa