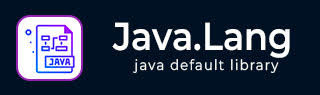
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - String intern() Method
The Java String intern() method is used to retrieve the canonical representation for the current string object. In short, the intern() method is used to make an exact copy of a String in heap memory and store it in the String constant pool. A pool is a special storage space in Java heap memory where the string literals can be stored.
A canonical representation indicates that values of a specific type of resource can be described or represented in multiple ways, with one of those ways are chosen as the preferred canonical form.
Note − The equality of two objects can easily be tested by testing the equality of their canonical forms.
Syntax
Following is the syntax of the Java String intern() method −
public String intern()
Parameters
It does not accept any parameter.
Return Value
This method returns a canonical representation for the string object.
Example
If the given string is not null, the intern() method creates the same copy of the current string object in the heap memory.
In the following program, we are instantiating the string class with the value “TutorialsPoint”. Using the intern() method, we are trying to create an exact copy of the current string in the heap memory.
import java.lang.*; public class Intern { public static void main(String[] args) { //instantiate the string class String str = new String("TutorialsPoint"); System.out.println("The given string is: " + str); //using the intern() method String str1 = str.intern(); System.out.println("The canonical representation of the current string : " + str1); } }
Output
On executing the above program, it will produce the following result −
The given string is: TutorialsPoint The canonical representation of the current string: TutorialsPoint
Example
If the given string has a null value, the intern() method throws the NullPointerException.
In the following example, we are creating a string literal with the null value. Using the intern() method, we are trying to retrieve the canonical representation of the current string object.
import java.lang.*; public class Intern { public static void main(String[] args) { try { //create string literal String str = null; System.out.println("The given string is: " + str); //using the intern() method String str1 = str.intern(); System.out.println("The canonical representation of the current : " + str1); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
Output
Following is the output of the above program −
The given string is: null java.lang.NullPointerException: Cannot invoke "String.intern()" because "str" is null at com.tutorialspoint.String.Intern.main(Intern.java:9) Exception: java.lang.NullPointerException: Cannot invoke "String.intern()" because "str" is null
Example
Using the intern() method we can check the equality of the string objects based on their conical forms.
In this program, we are creating an object of the string class with the value “Hello”. Then, using the intern() method, we are trying to retrieve the canonical representation of the string object, and compare them using the equals() method.
import java.lang.*; public class Intern { public static void main(String[] args) { // create an object of the string class String str = new String("Hello"); System.out.println("The given string is: " + str); // using intern() method String str2 = str.intern(); System.out.println("The canonical representation of the string object: " + str2); //using the conditional statement if (str.equals(str2)) { System.out.println("The string objects are equal based on their canonical forms"); } else { System.out.println("The string objects are not equal based on their canonical forms"); } } }
Output
The above program, produces the following output −
The given string is: Hello The canonical representation of the string object: Hello The string objects are equal based on their canonical forms