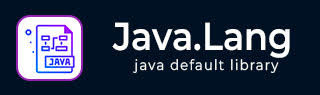
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Short valueOf(short s) method
Description
The Java Short valueOf(short s) method returns a Short instance representing the specified short value i.
Declaration
Following is the declaration for java.lang.Short.valueOf() method
public static Short valueOf(short s)
Parameters
i − This is an short value.
Return Value
This method returns a Short instance representing i.
Exception
NA
Example 1
The following example shows the usage of Short valueOf(short s) method to get the Short object using the specified short value. We've created a int variable and assigned it a positive short value. Then using valueOf() method, we're getting the object and printing it.
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { short s = 170; System.out.println("Number = " + s); /* returns the Short object of the given number */ System.out.println("valueOf = " + Short.valueOf(s)); } }
Output
Let us compile and run the above program, this will produce the following result −
Number = 170 valueOf = 170
Example 2
The following example shows the usage of Short valueOf(short s) method to get the Short object using the specified short value. We've created a int variable and assigned it a negative short value. Then using valueOf() method, we're getting the object and printing it.
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { short s = -170; System.out.println("Number = " + s); /* returns the Short object of the given number */ System.out.println("valueOf = " + Short.valueOf(s)); } }
Output
Let us compile and run the above program, this will produce the following result −
Number = -170 valueOf = -170
Example 3
The following example shows the usage of Short valueOf(short s) method to get the Short object using the specified short value. We've created a int variable and assigned it a zero value. Then using valueOf() method, we're getting the object and printing it.
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { short s = 0; System.out.println("Number = " + s); /* returns the Short object of the given number */ System.out.println("valueOf = " + Short.valueOf(s)); } }
Output
Let us compile and run the above program, this will produce the following result −
Number = 0 valueOf = 0