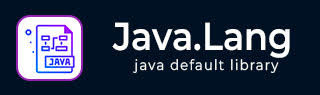
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Short decode() Method
A string is converted into a short by Java Short decode() method of the Short class. It accepts decimal, hexadecimal, and octal numbers given by the grammar that follows −
DecodableString −
Signopt DecimalNumeral
Signopt 0x HexDigits
Signopt 0x HexDigits
Signopt # HexDigits
Signopt 0 OctalDigits
The Short.parseShort method parses the sequence of characters after an optional sign and/or radix specifier ("0x", "0X", "#", or leading zero") according to the specified radix (10, 16, or 8). Unless a positive value is represented by this string of characters, a NumberFormatException will be raised. If the negative sign appears as the first character in the provided String, the result is negated. The String cannot contain any whitespace characters.
Syntax
Following is the syntax for Java Short decode() method −
public static Short decode(String nm) throws NumberFormatException
Parameters
nm − This is the String to decode.
Return Value
This method returns a Short object holding the short value represented by nm.
Example
When positive integers are passed as an argument to this method, it returns the same value.
Following is an example to decode the string value into short by passing a positive integer value in string argument to the created variable ‘x’ −
public class ShortDemo { public static void main(String[] args) { String x = "87"; Short decode = Short.decode(x); System.out.println("String value decoded to short is: = " + decode); } }
Output
Let us compile and run the above program, this will produce the following result −
String value decoded to short is: = 87
Example
When we pass octal number as an argument to this method, it returns the equivalent decoded string value to short.
In the following example the string variable ‘x’ is created. Then an octal value is assigned to this variable. After that it is decoded into short by passing octal number in string argument −
public class ShortDemo { public static void main(String[] args) { String x = "072"; Short decode = Short.decode(x); System.out.println("decoded String value in to Short = " + decode); } }
Output
Following is an output of the above code −
decoded String value in to Short = 58
Example
If we pass hexa number as an argument, the equivalent decoded string value to short is returned by this method.
Following is an example where a string variable ‘x’ is created. A hexa number is assigned to the variable. Then to decode the string value into short the hexa number is passed in string argument −
public class ShortDemo { public static void main(String[] args) { String x = "0x6f"; Short decode = Short.decode(x); System.out.println("decoded String value in to Short = " + decode); } }
Output
On executing the program above, the output is obtained as follows −
decoded String value in to Short = 111
Example
When we pass a string as an argument using this method, it returns a Number Format Exception.
In the following example the value "TutorialsPoint" assigned to the string variable ‘x’ does not contain a parsable short value. Hence, it throws the NumberFormatException −
public class ShortDemo { public static void main(String[] args) { String x = "TutorialsPoint"; Short decode = Short.decode(x); System.out.println("decoded String value in to Short = " + decode); } }
Number Format Exception
While executing the above code, we get the following output −
Exception in thread "main" java.lang.NumberFormatException: For input string: "TutorialsPoint" at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67) at java.base/java.lang.Integer.parseInt(Integer.java:668) at java.base/java.lang.Integer.decode(Integer.java:1454) at java.base/java.lang.Short.decode(Short.java:326) at ShortDemo.main(ShortDemo.java:4)