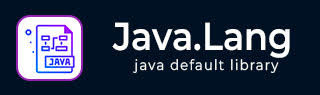
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Double valueOf(double) method
Description
The Java Double valueOf(double d) method returns a Double instance representing the specified double value d.
Declaration
Following is the declaration for java.lang.Double.valueOf() method
public static Double valueOf(double d)
Parameters
d − This is the double value.
Return Value
This method returns a a Double instance representing d.
Exception
NA
Example 1
The following example shows the usage of Double valueOf() method to get a Double object of a double value. We've initialized one Double object with a positive double using valueOf() method. Then we're printing the string representation value of the object.
package com.tutorialspoint; public class DoubleDemo { public static void main(String[] args) { Double d1 = Double.valueOf(6.5); // print the Double instance representing the specified double value System.out.println("Value = " + d1); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = 6.5
Example 2
The following example shows the usage of Double valueOf() method to get a Double object of a double value. We've initialized one Double object with a negative double using valueOf() method. Then we're printing the string representation value of the object.
package com.tutorialspoint; public class DoubleDemo { public static void main(String[] args) { Double d1 = Double.valueOf(-6.5); // print the Double instance representing the specified double value System.out.println("Value = " + d1); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = -6.5
Example 3
The following example shows the usage of Double valueOf() method to get a Double object of a double value. We've initialized one Double object with a negative zero double using valueOf() method. Then we're printing the string representation value of the object.
package com.tutorialspoint; public class DoubleDemo { public static void main(String[] args) { Double d1 = Double.valueOf(-0.0); // print the Double instance representing the specified double value System.out.println("Value = " + d1); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = -0.0
Example 4
The following example shows the usage of Double valueOf() method to get a Double object of a double value. We've initialized one Double object with a positive zero double using valueOf() method. Then we're printing the string representation value of the object.
package com.tutorialspoint; public class DoubleDemo { public static void main(String[] args) { Double d1 = Double.valueOf(0.0); // print the Double instance representing the specified double value System.out.println("Value = " + d1); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = 0.0