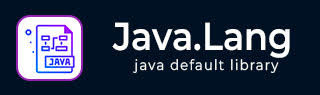
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
ClassLoader.getSystemResourceAsStream() Method Example
Description
The java.lang.ClassLoader.getSystemResourceAsStream() method opens for reading, a resource of the specified name from the search path used to load classes.
Declaration
Following is the declaration for java.lang.ClassLoader.getSystemResourceAsStream() method
public static InputStream getSystemResourceAsStream(String name)
Parameters
name − This is the resource name.
Return Value
This method returns an input stream for reading the resource, or null if the resource could not be found.
Exception
NA
Example
The following example shows the usage of java.lang.ClassLoader.getSystemResourceAsStream() method.
package com.tutorialspoint; import java.lang.*; import java.io.*; class ClassLoaderDemo { static String getResource(String rsc) { String val = ""; try { Class cls = Class.forName("ClassLoaderDemo"); // returns the ClassLoader object associated with this Class ClassLoader cLoader = cls.getClassLoader(); // input stream InputStream i = cLoader.getSystemResourceAsStream(rsc); BufferedReader r = new BufferedReader(new InputStreamReader(i)); // reads each line String l; while((l = r.readLine()) != null) { val = val + l; } i.close(); } catch(Exception e) { System.out.println(e); } return val; } public static void main(String[] args) { System.out.println("File1: " + getResource("file.txt")); System.out.println("File2: " + getResource("test.txt")); } }
Assuming we have a text file file.txt, which has the following content −
This is TutorialsPoint!
Assuming we have another text file test.txt, which has the following content −
This is Java Tutorial
Let us compile and run the above program, this will produce the following result −
File1: This is TutorialsPoint! File2: This is Java Tutorial
java_lang_classloader.htm
Advertisements