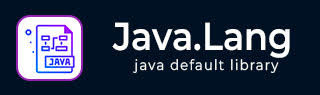
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Character toUpperCase() Method
The Java Character toUpperCase() method converts the character argument to uppercase using case mapping information from the UnicodeData file.
According to UnicodeData file, case is defined as the inherent property of a character. Case mappings in this file are informative and default mappings. For example, if a character is CAPITAL by default, then its corresponding lowercase is informative.
If a character is already present in the uppercase, the method will return the original character as itself.
This method occurs in two polymorphic methods with different parameters and return types.
Syntax
Following is the syntax for Java Character toUpperCase() method
public static char toUpperCase(char ch) (or) public static int toUpperCase(int codePoint)
Parameters
ch − the character to be converted
codePoint − the Unicode code point to be converted
Return Value
This method returns the uppercase equivalent of the character or code point, if any; otherwise, the character itself.
Example
The following example shows the usage of Java Character toUpperCase(char ch) method.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create 4 char primitives char ch1, ch2, ch3, ch4; // assign values to ch1, ch2 ch1 = '4'; ch2 = 'q'; // assign uppercase of ch1, ch2 to ch3, ch4 ch3 = Character.toUpperCase(ch1); ch4 = Character.toUpperCase(ch2); String str1 = "Uppercase of " + ch1 + " is " + ch3; String str2 = "Uppercase of " + ch2 + " is " + ch4; // print ch3, ch4 values System.out.println( str1 ); System.out.println( str2 ); } }
Output
Let us compile and run the above program, this will produce the following result −
Uppercase of 4 is 4 Uppercase of q is Q
Example
The following example shows the usage of Java Character toUpperCase(int codePoint) method.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create 4 int primitives int cp1, cp2, cp3, cp4; // assign values to cp1, cp2 cp1 = 0x0072; // represents r cp2 = 0x0569; // represents ARMENIAN SMALL LETTER TO // assign uppercase of cp1, cp2 to cp3, cp4 cp3 = Character.toUpperCase(cp1); cp4 = Character.toUpperCase(cp2); String str1 = "Uppercase equivalent of " + cp1 + " is " + cp3; String str2 = "Uppercase equivalent of " + cp2 + " is " + cp4; // print cp3, cp4 values System.out.println( str1 ); System.out.println( str2 ); } }
Output
Let us compile and run the above program, this will produce the following result −
Uppercase equivalent of 114 is 82 Uppercase equivalent of 1385 is 1337
Example
In the following example, we pass symbols as characters arguments to the method and the return values will be obtained as the arguments itself as symbols do not have case mapping.
import java.lang.*; public class UppercaseDemo { public static void main(String args[]) { char c1 = '%'; char c2 = Character.toUpperCase(c1); System.out.println("The uppercase value of " + c1 + " is " + c2); } }
Output
The output for the program above will be displayed as −
The uppercase value of % is %
Example
Another example to show the usage of the method is as follows. In this program, we pass an already uppercased character as an argument to the method.
import java.lang.*; public class Demo { public static void main(String args[]) { char c1 = 'D'; //already an uppercase character as input char c2 = Character.toUpperCase(c1); System.out.println("The uppercase value of " + c1 + " is " + c2); } }
Output
After compiling and running the program above, the output will be obtained as −
The uppercase value of D is D