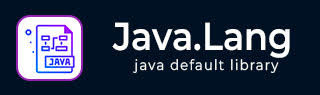
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java.lang.Character.isLowSurrogate() Method
Description
The java.lang.Character.isLowSurrogate(char ch) determines if the given char value is a Unicode low-surrogate code unit (also known as trailing-surrogate code unit).
Such values do not represent characters by themselves, but are used in the representation of supplementary characters in the UTF-16 encoding.
Declaration
Following is the declaration for java.lang.Character.isLowSurrogate() method
public static boolean isLowSurrogate(char ch)
Parameters
ch − the char value to be tested
Return Value
This method returns true if the char value is between MIN_LOW_SURROGATE and MAX_LOW_SURROGATE inclusive, false otherwise.
Exception
NA
Example
The following example shows the usage of lang.Character.isLowSurrogate() method.
package com.tutorialspoint; import java.lang.*; public class CharacterDemo { public static void main(String[] args) { // create 2 char primitives ch1, ch2 char ch1, ch2; // assign values to ch1, ch2 ch1 = '\udc28'; ch2 = 'a'; // create 2 boolean primitives b1, b2 boolean b1, b2; /** * check if ch1, ch2 are Unicode low-surrogate code units * and assign results to b1, b2 */ b1 = Character.isLowSurrogate(ch1); b2 = Character.isLowSurrogate(ch2); String str1 = "ch1 is a Unicode low-surrogate is " + b1; String str2 = ch2 + " is a Unicode low-surrogate is " + b2; // print b1, b2 values System.out.println( str1 ); System.out.println( str2 ); } }
Let us compile and run the above program, this will produce the following result −
ch1 is a Unicode low-surrogate is true a is a Unicode low-surrogate is false
java_lang_character.htm
Advertisements