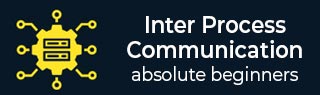
- Inter Process Communication
- Home
- Overview
- Process Information
- Process Image
- Process Creation & Termination
- Child Process Monitoring
- Process Groups, Sessions & Job Control
- Process Resources
- Other Processes
- Overlaying Process Image
- Related System Calls (System V)
- System V & Posix
- Pipes
- Named Pipes
- Shared Memory
- Message Queues
- Semaphores
- Signals
- Memory Mapping
- Useful Resources
- Quick Guide
- Useful Resources
- Discussion
Inter Process Communication - Semaphores
The first question that comes to mind is, why do we need semaphores? A simple answer, to protect the critical/common region shared among multiple processes.
Let us assume, multiple processes are using the same region of code and if all want to access parallelly then the outcome is overlapped. Say, for example, multiple users are using one printer only (common/critical section), say 3 users, given 3 jobs at same time, if all the jobs start parallelly, then one user output is overlapped with another. So, we need to protect that using semaphores i.e., locking the critical section when one process is running and unlocking when it is done. This would be repeated for each user/process so that one job is not overlapped with another job.
Basically semaphores are classified into two types −
Binary Semaphores − Only two states 0 & 1, i.e., locked/unlocked or available/unavailable, Mutex implementation.
Counting Semaphores − Semaphores which allow arbitrary resource count are called counting semaphores.
Assume that we have 5 printers (to understand assume that 1 printer only accepts 1 job) and we got 3 jobs to print. Now 3 jobs would be given for 3 printers (1 each). Again 4 jobs came while this is in progress. Now, out of 2 printers available, 2 jobs have been scheduled and we are left with 2 more jobs, which would be completed only after one of the resource/printer is available. This kind of scheduling as per resource availability can be viewed as counting semaphores.
To perform synchronization using semaphores, following are the steps −
Step 1 − Create a semaphore or connect to an already existing semaphore (semget())
Step 2 − Perform operations on the semaphore i.e., allocate or release or wait for the resources (semop())
Step 3 − Perform control operations on the message queue (semctl())
Now, let us check this with the system calls we have.
#include <sys/types.h> #include <sys/ipc.h> #include <sys/sem.h> int semget(key_t key, int nsems, int semflg)
This system call creates or allocates a System V semaphore set. The following arguments need to be passed −
The first argument, key, recognizes the message queue. The key can be either an arbitrary value or one that can be derived from the library function ftok().
The second argument, nsems, specifies the number of semaphores. If binary then it is 1, implies need of 1 semaphore set, otherwise as per the required count of number of semaphore sets.
The third argument, semflg, specifies the required semaphore flag/s such as IPC_CREAT (creating semaphore if it does not exist) or IPC_EXCL (used with IPC_CREAT to create semaphore and the call fails, if a semaphore already exists). Need to pass the permissions as well.
Note − Refer earlier sections for details on permissions.
This call would return valid semaphore identifier (used for further calls of semaphores) on success and -1 in case of failure. To know the cause of failure, check with errno variable or perror() function.
Various errors with respect to this call are EACCESS (permission denied), EEXIST (queue already exists can’t create), ENOENT (queue doesn’t exist), ENOMEM (not enough memory to create the queue), ENOSPC (maximum sets limit exceeded), etc.
#include <sys/types.h> #include <sys/ipc.h> #include <sys/sem.h> int semop(int semid, struct sembuf *semops, size_t nsemops)
This system call performs the operations on the System V semaphore sets viz., allocating resources, waiting for the resources or freeing the resources. Following arguments need to be passed −
The first argument, semid, indicates semaphore set identifier created by semget().
The second argument, semops, is the pointer to an array of operations to be performed on the semaphore set. The structure is as follows −
struct sembuf { unsigned short sem_num; /* Semaphore set num */ short sem_op; /* Semaphore operation */ short sem_flg; /* Operation flags, IPC_NOWAIT, SEM_UNDO */ };
Element, sem_op, in the above structure, indicates the operation that needs to be performed −
If sem_op is –ve, allocate or obtain resources. Blocks the calling process until enough resources have been freed by other processes, so that this process can allocate.
If sem_op is zero, the calling process waits or sleeps until semaphore value reaches 0.
If sem_op is +ve, release resources.
For example −
struct sembuf sem_lock = { 0, -1, SEM_UNDO };
struct sembuf sem_unlock = {0, 1, SEM_UNDO };
The third argument, nsemops, is the number of operations in that array.
#include <sys/types.h> #include <sys/ipc.h> #include <sys/sem.h> int semctl(int semid, int semnum, int cmd, …)
This system call performs control operation for a System V semaphore. The following arguments need to be passed −
The first argument, semid, is the identifier of the semaphore. This id is the semaphore identifier, which is the return value of semget() system call.
The second argument, semnum, is the number of semaphore. The semaphores are numbered from 0.
The third argument, cmd, is the command to perform the required control operation on the semaphore.
The fourth argument, of type, union semun, depends on the cmd. For few cases, the fourth argument is not applicable.
Let us check the union semun −
union semun { int val; /* val for SETVAL */ struct semid_ds *buf; /* Buffer for IPC_STAT and IPC_SET */ unsigned short *array; /* Buffer for GETALL and SETALL */ struct seminfo *__buf; /* Buffer for IPC_INFO and SEM_INFO*/ };
The semid_ds data structure which is defined in sys/sem.h is as follows −
struct semid_ds { struct ipc_perm sem_perm; /* Permissions */ time_t sem_otime; /* Last semop time */ time_t sem_ctime; /* Last change time */ unsigned long sem_nsems; /* Number of semaphores in the set */ };
Note − Please refer manual pages for other data structures.
union semun arg; Valid values for cmd are −
IPC_STAT − Copies the information of the current values of each member of struct semid_ds to the passed structure pointed by arg.buf. This command requires read permission to the semaphore.
IPC_SET − Sets the user ID, group ID of the owner, permissions, etc. pointed to by the structure semid_ds.
IPC_RMID − Removes the semaphores set.
IPC_INFO − Returns the information about the semaphore limits and parameters in the structure semid_ds pointed by arg.__buf.
SEM_INFO − Returns a seminfo structure containing information about the consumed system resources by the semaphore.
This call would return value (non-negative value) depending upon the passed command. Upon success, IPC_INFO and SEM_INFO or SEM_STAT returns the index or identifier of the highest used entry as per Semaphore or the value of semncnt for GETNCNT or the value of sempid for GETPID or the value of semval for GETVAL 0 for other operations on success and -1 in case of failure. To know the cause of failure, check with errno variable or perror() function.
Before looking at the code, let us understand its implementation −
Create two processes say, child and parent.
Create shared memory mainly needed to store the counter and other flags to indicate end of read/write process into the shared memory.
The counter is incremented by count by both parent and child processes. The count is either passed as a command line argument or taken as default (if not passed as command line argument or the value is less than 10000). Called with certain sleep time to ensure both parent and child accesses the shared memory at the same time i.e., in parallel.
Since, the counter is incremented in steps of 1 by both parent and child, the final value should be double the counter. Since, both parent and child processes performing the operations at same time, the counter is not incremented as required. Hence, we need to ensure the completeness of one process completion followed by other process.
All the above implementations are performed in the file shm_write_cntr.c
Check if the counter value is implemented in file shm_read_cntr.c
To ensure completion, the semaphore program is implemented in file shm_write_cntr_with_sem.c. Remove the semaphore after completion of the entire process (after read is done from other program)
Since, we have separate files to read the value of counter in the shared memory and don’t have any effect from writing, the reading program remains the same (shm_read_cntr.c)
It is always better to execute the writing program in one terminal and reading program from another terminal. Since, the program completes execution only after the writing and reading process is complete, it is ok to run the program after completely executing the write program. The write program would wait until the read program is run and only finishes after it is done.
Programs without semaphores.
/* Filename: shm_write_cntr.c */ #include<stdio.h> #include<sys/ipc.h> #include<sys/shm.h> #include<sys/types.h> #include<string.h> #include<errno.h> #include<stdlib.h> #include<unistd.h> #include<string.h> #define SHM_KEY 0x12345 struct shmseg { int cntr; int write_complete; int read_complete; }; void shared_memory_cntr_increment(int pid, struct shmseg *shmp, int total_count); int main(int argc, char *argv[]) { int shmid; struct shmseg *shmp; char *bufptr; int total_count; int sleep_time; pid_t pid; if (argc != 2) total_count = 10000; else { total_count = atoi(argv[1]); if (total_count < 10000) total_count = 10000; } printf("Total Count is %d\n", total_count); shmid = shmget(SHM_KEY, sizeof(struct shmseg), 0644|IPC_CREAT); if (shmid == -1) { perror("Shared memory"); return 1; } // Attach to the segment to get a pointer to it. shmp = shmat(shmid, NULL, 0); if (shmp == (void *) -1) { perror("Shared memory attach"); return 1; } shmp->cntr = 0; pid = fork(); /* Parent Process - Writing Once */ if (pid > 0) { shared_memory_cntr_increment(pid, shmp, total_count); } else if (pid == 0) { shared_memory_cntr_increment(pid, shmp, total_count); return 0; } else { perror("Fork Failure\n"); return 1; } while (shmp->read_complete != 1) sleep(1); if (shmdt(shmp) == -1) { perror("shmdt"); return 1; } if (shmctl(shmid, IPC_RMID, 0) == -1) { perror("shmctl"); return 1; } printf("Writing Process: Complete\n"); return 0; } /* Increment the counter of shared memory by total_count in steps of 1 */ void shared_memory_cntr_increment(int pid, struct shmseg *shmp, int total_count) { int cntr; int numtimes; int sleep_time; cntr = shmp->cntr; shmp->write_complete = 0; if (pid == 0) printf("SHM_WRITE: CHILD: Now writing\n"); else if (pid > 0) printf("SHM_WRITE: PARENT: Now writing\n"); //printf("SHM_CNTR is %d\n", shmp->cntr); /* Increment the counter in shared memory by total_count in steps of 1 */ for (numtimes = 0; numtimes < total_count; numtimes++) { cntr += 1; shmp->cntr = cntr; /* Sleeping for a second for every thousand */ sleep_time = cntr % 1000; if (sleep_time == 0) sleep(1); } shmp->write_complete = 1; if (pid == 0) printf("SHM_WRITE: CHILD: Writing Done\n"); else if (pid > 0) printf("SHM_WRITE: PARENT: Writing Done\n"); return; }
Compilation and Execution Steps
Total Count is 10000 SHM_WRITE: PARENT: Now writing SHM_WRITE: CHILD: Now writing SHM_WRITE: PARENT: Writing Done SHM_WRITE: CHILD: Writing Done Writing Process: Complete
Now, let us check the shared memory reading program.
/* Filename: shm_read_cntr.c */ #include<stdio.h> #include<sys/ipc.h> #include<sys/shm.h> #include<sys/types.h> #include<string.h> #include<errno.h> #include<stdlib.h> #include<unistd.h> #define SHM_KEY 0x12345 struct shmseg { int cntr; int write_complete; int read_complete; }; int main(int argc, char *argv[]) { int shmid, numtimes; struct shmseg *shmp; int total_count; int cntr; int sleep_time; if (argc != 2) total_count = 10000; else { total_count = atoi(argv[1]); if (total_count < 10000) total_count = 10000; } shmid = shmget(SHM_KEY, sizeof(struct shmseg), 0644|IPC_CREAT); if (shmid == -1) { perror("Shared memory"); return 1; } // Attach to the segment to get a pointer to it. shmp = shmat(shmid, NULL, 0); if (shmp == (void *) -1) { perror("Shared memory attach"); return 1; } /* Read the shared memory cntr and print it on standard output */ while (shmp->write_complete != 1) { if (shmp->cntr == -1) { perror("read"); return 1; } sleep(3); } printf("Reading Process: Shared Memory: Counter is %d\n", shmp->cntr); printf("Reading Process: Reading Done, Detaching Shared Memory\n"); shmp->read_complete = 1; if (shmdt(shmp) == -1) { perror("shmdt"); return 1; } printf("Reading Process: Complete\n"); return 0; }
Compilation and Execution Steps
Reading Process: Shared Memory: Counter is 11000 Reading Process: Reading Done, Detaching Shared Memory Reading Process: Complete
If you observe the above output, the counter should be 20000, however, since before completion of one process task other process is also processing in parallel, the counter value is not as expected. The output would vary from system to system and also it would vary with each execution. To ensure the two processes perform the task after completion of one task, it should be implemented using synchronization mechanisms.
Now, let us check the same application using semaphores.
Note − Reading program remains the same.
/* Filename: shm_write_cntr_with_sem.c */ #include<stdio.h> #include<sys/types.h> #include<sys/ipc.h> #include<sys/shm.h> #include<sys/sem.h> #include<string.h> #include<errno.h> #include<stdlib.h> #include<unistd.h> #include<string.h> #define SHM_KEY 0x12345 #define SEM_KEY 0x54321 #define MAX_TRIES 20 struct shmseg { int cntr; int write_complete; int read_complete; }; void shared_memory_cntr_increment(int, struct shmseg*, int); void remove_semaphore(); int main(int argc, char *argv[]) { int shmid; struct shmseg *shmp; char *bufptr; int total_count; int sleep_time; pid_t pid; if (argc != 2) total_count = 10000; else { total_count = atoi(argv[1]); if (total_count < 10000) total_count = 10000; } printf("Total Count is %d\n", total_count); shmid = shmget(SHM_KEY, sizeof(struct shmseg), 0644|IPC_CREAT); if (shmid == -1) { perror("Shared memory"); return 1; } // Attach to the segment to get a pointer to it. shmp = shmat(shmid, NULL, 0); if (shmp == (void *) -1) { perror("Shared memory attach: "); return 1; } shmp->cntr = 0; pid = fork(); /* Parent Process - Writing Once */ if (pid > 0) { shared_memory_cntr_increment(pid, shmp, total_count); } else if (pid == 0) { shared_memory_cntr_increment(pid, shmp, total_count); return 0; } else { perror("Fork Failure\n"); return 1; } while (shmp->read_complete != 1) sleep(1); if (shmdt(shmp) == -1) { perror("shmdt"); return 1; } if (shmctl(shmid, IPC_RMID, 0) == -1) { perror("shmctl"); return 1; } printf("Writing Process: Complete\n"); remove_semaphore(); return 0; } /* Increment the counter of shared memory by total_count in steps of 1 */ void shared_memory_cntr_increment(int pid, struct shmseg *shmp, int total_count) { int cntr; int numtimes; int sleep_time; int semid; struct sembuf sem_buf; struct semid_ds buf; int tries; int retval; semid = semget(SEM_KEY, 1, IPC_CREAT | IPC_EXCL | 0666); //printf("errno is %d and semid is %d\n", errno, semid); /* Got the semaphore */ if (semid >= 0) { printf("First Process\n"); sem_buf.sem_op = 1; sem_buf.sem_flg = 0; sem_buf.sem_num = 0; retval = semop(semid, &sem_buf, 1); if (retval == -1) { perror("Semaphore Operation: "); return; } } else if (errno == EEXIST) { // Already other process got it int ready = 0; printf("Second Process\n"); semid = semget(SEM_KEY, 1, 0); if (semid < 0) { perror("Semaphore GET: "); return; } /* Waiting for the resource */ sem_buf.sem_num = 0; sem_buf.sem_op = 0; sem_buf.sem_flg = SEM_UNDO; retval = semop(semid, &sem_buf, 1); if (retval == -1) { perror("Semaphore Locked: "); return; } } sem_buf.sem_num = 0; sem_buf.sem_op = -1; /* Allocating the resources */ sem_buf.sem_flg = SEM_UNDO; retval = semop(semid, &sem_buf, 1); if (retval == -1) { perror("Semaphore Locked: "); return; } cntr = shmp->cntr; shmp->write_complete = 0; if (pid == 0) printf("SHM_WRITE: CHILD: Now writing\n"); else if (pid > 0) printf("SHM_WRITE: PARENT: Now writing\n"); //printf("SHM_CNTR is %d\n", shmp->cntr); /* Increment the counter in shared memory by total_count in steps of 1 */ for (numtimes = 0; numtimes < total_count; numtimes++) { cntr += 1; shmp->cntr = cntr; /* Sleeping for a second for every thousand */ sleep_time = cntr % 1000; if (sleep_time == 0) sleep(1); } shmp->write_complete = 1; sem_buf.sem_op = 1; /* Releasing the resource */ retval = semop(semid, &sem_buf, 1); if (retval == -1) { perror("Semaphore Locked\n"); return; } if (pid == 0) printf("SHM_WRITE: CHILD: Writing Done\n"); else if (pid > 0) printf("SHM_WRITE: PARENT: Writing Done\n"); return; } void remove_semaphore() { int semid; int retval; semid = semget(SEM_KEY, 1, 0); if (semid < 0) { perror("Remove Semaphore: Semaphore GET: "); return; } retval = semctl(semid, 0, IPC_RMID); if (retval == -1) { perror("Remove Semaphore: Semaphore CTL: "); return; } return; }
Compilation and Execution Steps
Total Count is 10000 First Process SHM_WRITE: PARENT: Now writing Second Process SHM_WRITE: PARENT: Writing Done SHM_WRITE: CHILD: Now writing SHM_WRITE: CHILD: Writing Done Writing Process: Complete
Now, we will check the counter value by the reading process.
Execution Steps
Reading Process: Shared Memory: Counter is 20000 Reading Process: Reading Done, Detaching Shared Memory Reading Process: Complete