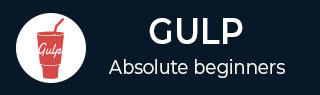
- Gulp Tutorial
- Gulp - Home
- Gulp - Overview
- Gulp - Installation
- Gulp - Basics
- Gulp - Developing An Application
- Gulp - Combining Tasks
- Gulp - Watch
- Gulp - Live Reload
- Gulp - Optimizing CSS and JavaScript
- Gulp - Optimizing Images
- Gulp - Useful Plugins
- Gulp - Cleaning Unwanted Files
- Gulp Useful Resources
- Gulp - Quick Guide
- Gulp - Useful Resources
- Gulp - Discussion
Gulp - Combining Tasks
Task enables a modular approach to configure Gulp. We need to create task for each dependency, which we would add up as we find and install other plugins. The Gulp task will have following structure −
gulp.task('task-name', function() { //do stuff here });
Where “task-name” is a string name and “function()” performs your task. The “gulp.task” registers the function as a task within the name and specifies the dependencies on other tasks.
Installing Plugins
Let's take one plugin called minify-css to merge and minify all CSS scripts. It can be installed by using npm as shown in the following command −
npm install gulp-minify-css --save-dev
To work with “gulp-minify-css plugin”, you need to install another plugin called “gulp-autoprefixer” as shown in the following command −
npm install gulp-autoprefixer --save-dev
To concatenate the CSS files, install the gulp-concat as shown in the following command −
npm install gulp-concat --save-dev
After installation of plugins, you need to write dependencies in your configuration file as follows −
var autoprefix = require('gulp-autoprefixer'); var minifyCSS = require('gulp-minify-css'); var concat = require('gulp-concat');
Adding Task to Gulp file
We need to create task for each dependency, which we would add up as we install the plugins. The Gulp task will have following structure −
gulp.task('styles', function() { gulp.src(['src/styles/*.css']) .pipe(concat('styles.css')) .pipe(autoprefix('last 2 versions')) .pipe(minifyCSS()) .pipe(gulp.dest('build/styles/')); });
The ‘concat’ plugin concatenates the CSS files and ‘autoprefix’ plugin indicates the current and the previous versions of all browsers. It minifies all CSS scripts from src folder and copies to the build folder by calling ‘dest’ method with an argument, which represents the target directory.
To run the task, use the following command in your project directory −
gulp styles
Similarly, we will use another plugin called ‘gulp-imagemin’ to compress the image file, which can be installed using the following command −
npm install gulp-imagemin --save-dev
You can add dependencies to your configuration file using the following command −
var imagemin = require('gulp-imagemin');
You can create the task for above defined dependency as shown in the following code.
gulp.task('imagemin', function() { var img_src = 'src/images/**/*', img_dest = 'build/images'; gulp.src(img_src) .pipe(changed(img_dest)) .pipe(imagemin()) .pipe(gulp.dest(img_dest)); });
The images are located in “src/images/**/*” which are saved in the img_srcobject. It is piped to other functions created by the ‘imagemin’ constructor. It compresses the images from src folder and copies to the build folder by calling ‘dest’ method with an argument, which represents the target directory.
To run the task, use the following command in your project directory −
gulp imagemin
Combining Multiple Tasks
You can run multiple tasks at a time by creating default task in the configuration file as shown in the following code −
gulp.task('default', ['imagemin', 'styles'], function() { });
Gulp file is set up and ready to execute. Run the following command in your project directory to run the above combined tasks −
gulp
On running the task using the above command, you will get the following result in the command prompt −
C:\work>gulp [16:08:51] Using gulpfile C:\work\gulpfile.js [16:08:51] Starting 'imagemin'... [16:08:51] Finished 'imagemin' after 20 ms [16:08:51] Starting 'styles'... [16:08:51] Finished 'styles' after 13 ms [16:08:51] Starting 'default'... [16:08:51] Finished 'default' after 6.13 ms [16:08:51] gulp-imagemin: Minified 0 images