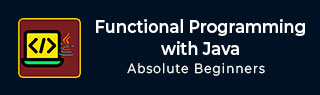
- Functional Programming with Java Tutorial
- Home
- Overview
- Aspects
- Functions
- Functional Composition
- Eager vs Lazy Evaluation
- Persistent Data Structure
- Recursion
- Parallelism
- Optionals & Monads
- Closure
- Currying
- Reducing
- Java 8 Onwards
- Lambda Expressions
- Default Methods
- Functional Interfaces
- Method References
- Constructor References
- Collections
- Functional Programming
- High Order Functions
- Returning a Function
- First Class Functions
- Pure Functions
- Type Inference
- Exception Handling in Lambda Expressions
- Streams
- Intermediate Methods
- Terminal methods
- Infinite Streams
- Fixed Length Streams
- Useful Resources
- Quick Guide
- Resources
- Discussion
Functional Programming - Infinite Streams
Collections are in-memory data structure which have all the elements present in the collection and we have external iteration to iterate through collection whereas Stream is a fixed data structure where elements are computed on demand and a Stream has inbuilt iteration to iterate through each element. Following example shows how to create a Stream from an array.
int[] numbers = {1, 2, 3, 4}; IntStream numbersFromArray = Arrays.stream(numbers);
Above stream is of fixed size being built from an array of four numbers and will not return element after 4th element. But we can create a Stream using Stream.iterate() or Stream.generate() method which can have lamdba expression will pass to a Stream. Using lamdba expression, we can pass a condition which once fulfilled give the required elements. Consider the case, where we need a list of numbers which are multiple of 3.
Example - Infinite Stream
import java.util.stream.Stream; public class FunctionTester { public static void main(String[] args) { //create a stream of numbers which are multiple of 3 Stream<Integer> numbers = Stream.iterate(0, n -> n + 3); numbers .limit(10) .forEach(System.out::println); } }
Output
0 3 6 9 12 15 18 21 24 27
In order to operate on infinite stream, we've used limit() method of Stream interface to restrict the iteration of numbers when their count become 10.