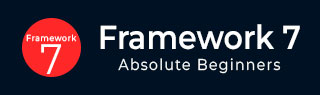
- Framework7 Tutorial
- Framework7 - Home
- Framework7 - Overview
- Framework7 - Environment
- Framework7 Components
- Framework7 - Layouts
- Framework7 - Navbars
- Framework7 - Toolbars
- Framework7 - Search Bar
- Framework7 - Status Bar
- Framework7 - Side Panels
- Framework7 - Content Block
- Framework7 - Layout Grid
- Framework7 - Overlays
- Framework7 - Preloaders
- Framework7 - Progress Bar
- Framework7 - List Views
- Framework7 - Accordion
- Framework7 - Cards
- Framework7 - Chips
- Framework7 - Buttons
- Framework7 - Action Button
- Framework7 - Forms
- Framework7 - Tabs
- Framework7 - Swiper Slider
- Framework7 - Photo Browser
- Framework7 - Autocomplete
- Framework7 - Picker
- Framework7 - Calendar
- Framework7 - Refresh
- Framework7 - Infinite Scroll
- Framework7 - Messages
- Framework7 - Message Bar
- Framework7 - Notifications
- Framework7 - Lazy Load
- Framework7 Styling
- Framework7 - Color Themes
- Framework7 - Hairlines
- Framework7 Templates
- Framework7 - Templates Overview
- Framework7 - Auto Compilation
- Framework7 - Template7 Pages
- Framework7 Fast Clicks
- Framework7 - Active State
- Framework7 - Tap Hold Event
- Framework7 - Touch Ripple
- Framework7 Useful Resources
- Framework7 - Quick Guide
- Framework7 - Useful Resources
- Framework7 - Discussion
Framework7 - Create & Open Action Sheet
Description
Since Action Sheet is a dynamic element, it could be created and opened using JavaScript only. The related app's methods to create Action Sheet are listed below −
myApp.actions(groups) − It is used to create and open Action Sheet with specified number of buttons groups.
myApp.actions(buttons) − It is used to create and open Action Sheet with a group and defined number of buttons.
groups − It is an array of groups where each group containing array of buttons.
buttons − It is an array of buttons wherein there will be one group.
This method will return dynamically created HTMLElement of the Action Sheet.
Every button in an array of buttons must be presented as Object with the button parameters as listed in the following table −
S.No | Parameter & Description | Type | Default |
---|---|---|---|
1 | text It is the String with Button's text. |
string | |
2 | bold It is optional parameter, which makes the button's text bolder when set to true. |
boolean | false |
3 | color It is an optional parameter and it is one of the 10 default button color. |
string | |
4 | bg It is an optional parameter and it is one of the 10 default button background color. |
string | |
5 | label It is an optional parameter and if is set to true then, it will be the title instead of button. |
boolean | true |
6 | disabled It is an optional parameter and if you want to disable a button then, set this parameter to true. |
boolean | false |
7 | onClick It is an optional parameter and is the callback function which will be executed whenever the user clicks this button. |
function |
Example
The following example demonstrates the use of Action Sheet in Framework7, which displays one group and specified amount of buttons groups when you click on the links −
<!DOCTYPE html> <html> <head> <meta name = "viewport" content = "width = device-width, initial-scale = 1, maximum-scale = 1, minimum-scale = 1, user-scalable = no, minimal-ui" /> <meta name = "apple-mobile-web-app-capable" content = "yes" /> <meta name = "apple-mobile-web-app-status-bar-style" content = "black" /> <title>Create and open Action Sheet</title> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.min.css" /> <link rel = "stylesheet" href = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/css/framework7.ios.colors.min.css" /> </head> <body> <div class = "views"> <div class = "view view-main"> <div class = "pages"> <div data-page = "home" class = "page navbar-fixed"> <div class = "navbar"> <div class = "navbar-inner"> <div class = "left"> </div> <div class = "center">Action Sheet</div> <div class = "right"> </div> </div> </div> <div class = "page-content"> <div class = "content-block"> <p><a href = "#" class = "ac-1"><b>One group</b> with <b>three buttons</b></a></p> <p><a href = "#" class = "ac-2"><b>One group</b> with <b>title</b> and <b>three buttons</b></a></p> <p><a href = "#" class = "ac-3"><b>Two groups</b></a></p> <p><a href = "#" class = "ac-4"><b>Three groups</b></a></p> <p><a href = "#" class = "ac-5">With <b>callbacks</b> on click</a></p> </div> </div> </div> </div> </div> </div> <script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/framework7/1.4.2/js/framework7.min.js"></script> <script> var myApp = new Framework7(); var $$ = Dom7; $$('.ac-1').on('click', function () { var buttons = [ { text: 'Button1', color: 'green' }, { text: 'Button2', color: 'green' }, { text: 'Cancel', color: 'red' }, ]; myApp.actions(buttons); }); $$('.ac-2').on('click', function () { var buttons = [ { text: 'Some Text', label: true }, { text: 'Button1', color: 'pink' }, { text: 'Button2', color: 'pink' }, { text: 'Cancel', color: 'green' }, ]; myApp.actions(buttons); }); $$('.ac-3').on('click', function () { var buttons1 = [ { text: 'Some Text', label: true }, { text: 'Button1', bold: true }, { text: 'Button2', bold: true }, { text: 'Button3', bold: true }, { text: 'Button4', } ]; var buttons2 = [ { text: 'Cancel', color: 'red' } ]; var groups = [buttons1, buttons2]; myApp.actions(groups); }); $$('.ac-4').on('click', function () { var buttons1 = [ { text: 'Share', label: true }, { text: 'Email', }, { text: 'Message', } ]; var buttons2 = [ { text: 'Social Networks', label: true }, { text: 'Facebook', }, { text: 'YouTube', } ]; var buttons3 = [ { text: 'Cancel', color: 'red' } ]; var groups = [buttons1, buttons2, buttons3]; myApp.actions(groups); }); $$('.ac-5').on('click', function () { var buttons = [ { text: 'Callback Button1', onClick: function () { myApp.alert('Callback Button1 clicked'); } }, { text: 'Callback Button2', onClick: function () { myApp.alert('Callback Button2 clicked'); } }, { text: 'Cancel', color: 'red', onClick: function () { myApp.alert('Cancel clicked'); } }, ]; myApp.actions(buttons); }); </script> </body> </html>
Output
Let us carry out the following steps to see how the above given code works −
Save the above given HTML code as actionsheet_create_open.html file in your server root folder.
Open this HTML file as http://localhost/actionsheet_create_open.html and the output is displayed as shown below.
When you click on the first option, action sheet is created with three buttons in one group.
When you click the second option, action sheet is created with three buttons along with a title in one group.
When you click the third option, two groups are created and when you click the fourth option, three groups are created.
In the last option, a callback function is executed on clicking the options.
To Continue Learning Please Login