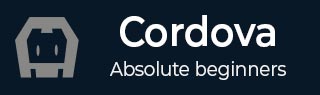
- Cordova Tutorial
- Cordova - Home
- Cordova - Overview
- Cordova - Environment Setup
- Cordova - First Application
- Cordova - Config.xml File
- Cordova - Storage
- Cordova - Events
- Cordova - Back Button
- Cordova - Plugman
- Cordova - Battery Status
- Cordova - Camera
- Cordova - Contacts
- Cordova - Device
- Cordova - Accelerometer
- Cordova - Device Orientation
- Cordova - Dialogs
- Cordova - File System
- Cordova - File Transfer
- Cordova - Geolocation
- Cordova - Globalization
- Cordova - InAppBrowser
- Cordova - Media
- Cordova - Media Capture
- Cordova - Network Information
- Cordova - Splash Screen
- Cordova - Vibration
- Cordova - Whitelist
- Cordova - Best Practices
- Cordova Useful Resources
- Cordova - Quick Guide
- Cordova - Useful Resources
- Cordova - Discussion
Cordova - Network Information
This plugin provides information about device's network.
Step 1 - Installing Network Information Plugin
To install this plugin, we will open command prompt and run the following code −
C:\Users\username\Desktop\CordovaProject>cordova plugin add cordova-plugin-network-information
Step 2 - Add Buttons
Let's create one button in index.html that will be used for getting info about network.
<button id = "networkInfo">INFO</button>
Step 3 - Add Event Listeners
We will add three event listeners inside onDeviceReady function in index.js. One will listen for clicks on the button we created before and the other two will listen for changes in connection status.
document.getElementById("networkInfo").addEventListener("click", networkInfo); document.addEventListener("offline", onOffline, false); document.addEventListener("online", onOnline, false);
Step 4 - Creating Functions
networkInfo function will return info about current network connection once button is clicked. We are calling type method. The other functions are onOffline and onOnline. These functions are listening to the connection changes and any change will trigger the corresponding alert message.
function networkInfo() { var networkState = navigator.connection.type; var states = {}; states[Connection.UNKNOWN] = 'Unknown connection'; states[Connection.ETHERNET] = 'Ethernet connection'; states[Connection.WIFI] = 'WiFi connection'; states[Connection.CELL_2G] = 'Cell 2G connection'; states[Connection.CELL_3G] = 'Cell 3G connection'; states[Connection.CELL_4G] = 'Cell 4G connection'; states[Connection.CELL] = 'Cell generic connection'; states[Connection.NONE] = 'No network connection'; alert('Connection type: ' + states[networkState]); } function onOffline() { alert('You are now offline!'); } function onOnline() { alert('You are now online!'); }
When we start the app connected to the network, onOnline function will trigger alert.
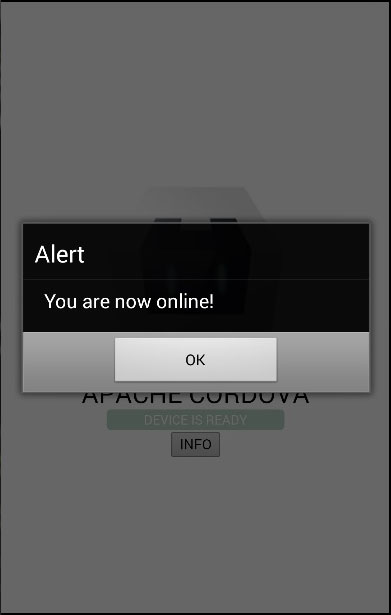
If we press INFO button the alert will show our network state.
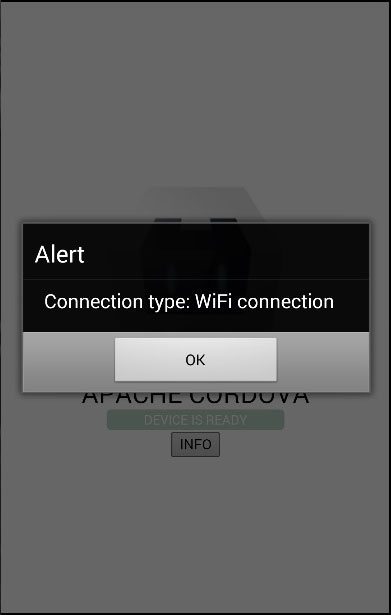
If we disconnect from the network, onOffline function will be called.
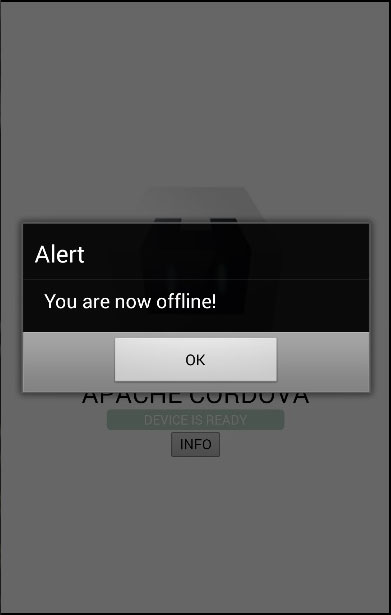