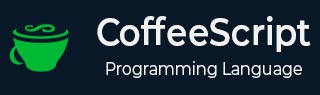
- CoffeeScript Tutorial
- CoffeeScript - Home
- CoffeeScript - Overview
- CoffeeScript - Environment
- CoffeeScript - command-line utility
- CoffeeScript - Syntax
- CoffeeScript - Data Types
- CoffeeScript - Variables
- CoffeeScript - Operators and Aliases
- CoffeeScript - Conditionals
- CoffeeScript - Loops
- CoffeeScript - Comprehensions
- CoffeeScript - Functions
- CoffeeScript Object Oriented
- CoffeeScript - Strings
- CoffeeScript - Arrays
- CoffeeScript - Objects
- CoffeeScript - Ranges
- CoffeeScript - Splat
- CoffeeScript - Date
- CoffeeScript - Math
- CoffeeScript - Exception Handling
- CoffeeScript - Regular Expressions
- CoffeeScript - Classes and Inheritance
- CoffeeScript Advanced
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript - MongoDB
- CoffeeScript - SQLite
- CoffeeScript Useful Resources
- CoffeeScript - Quick Guide
- CoffeeScript - Useful Resources
- CoffeeScript - Discussion
CoffeeScript - The loop variant of while
The loop variant is equivalent to the while loop with true value (while true). The statements in this loop will be executed repeatedly until we exit the loop using the break statement.
Syntax
Given below is the syntax of the loop alternative of the while loop in CoffeeScript.
loop statements to be executed repeatedly condition to exit the loop
Example
The following example demonstrates the usage of until loop in CoffeeScript. Here we have used the Math function random() to generate random numbers, and if the number generated is 3, we are exiting the loop using break statement. Save this code in a file with name until_loop_example.coffee
loop num = Math.random()*8|0 console.log num if num == 5 then break
Open the command prompt and compile the .coffee file as shown below.
c:\> coffee -c loop_example.coffee
On compiling, it gives you the following JavaScript.
// Generated by CoffeeScript 1.10.0 (function() { var num; while (true) { num = Math.random() * 8 | 0; console.log(num); if (num === 5) { break; } } }).call(this);
Now, open the command prompt again and run the Coffee Script file as shown below.
c:\> coffee loop_example.coffee
On executing, the CoffeeScript file produces the following output.
2 0 2 3 7 4 6 2 0 1 4 6 5
To Continue Learning Please Login