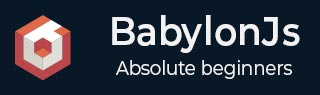
- BabylonJS Tutorial
- BabylonJS - Home
- BabylonJS - Introduction
- BabylonJS - Environment Setup
- BabylonJS - Overview
- BabylonJS - Basic Elements
- BabylonJS - Materials
- BabylonJS - Animations
- BabylonJS - Cameras
- BabylonJS - Lights
- BabylonJS - Parametric Shapes
- BabylonJS - Mesh
- VectorPosition and Rotation
- BabylonJS - Decals
- BabylonJS - Curve3
- BabylonJS - Dynamic Texture
- BabylonJS - Parallax Mapping
- BabylonJS - Lens Flares
- BabylonJS - Create ScreenShot
- BabylonJS - Reflection Probes
- Standard Rendering Pipeline
- BabylonJS - ShaderMaterial
- BabylonJS - Bones and Skeletons
- BabylonJS - Physics Engine
- BabylonJS - Playing Sounds & Music
- BabylonJS Useful Resources
- BabylonJS - Quick Guide
- BabylonJS - Useful Resources
- BabylonJS - Discussion
BabylonJS - Mesh Instances
If you want to draw identical meshes in your scene , make use of the instances.
Syntax
var newInstance = mesh.createInstance("i" + index); // creates new instance
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = BABYLON.Color3.Gray(); // This creates and positions a free camera (non-mesh) var camera = new BABYLON.FreeCamera("camera1", new BABYLON.Vector3(0, 5, -10), scene); // This targets the camera to scene origin camera.setTarget(BABYLON.Vector3.Zero()); // This attaches the camera to the canvas camera.attachControl(canvas, true); var light = new BABYLON.DirectionalLight("dir01", new BABYLON.Vector3(0, -1, -0.3), scene); light.position = new BABYLON.Vector3(20, 60, 30); // Ground var ground = BABYLON.Mesh.CreateGround("ground1", 50, 50, 2, scene); ground.receiveShadows = true; var array_instances = []; // Trees BABYLON.SceneLoader.ImportMesh("", "scenes/", "Rabbit.babylon", scene, function (newMeshes, particleSystems, skeletons) { var rabbit = newMeshes[1]; rabbit.isVisible = false; var range = 50; var count = 100; for (var index = 0; index < count; index++) { var newInstance = rabbit.createInstance("i" + index); var x = range / 2 - Math.random() * range; var z = range / 2 - Math.random() * range; newInstance.position = new BABYLON.Vector3(x, 0, z); newInstance.scaling = new BABYLON.Vector3(0.05, 0.05, 0.05); array_instances.push(newInstance); } }); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
In the above demo link, we have used Rabbit.babylon mesh. You can download the json file for Rabbit.babylon from here
Save the file in scenes to get the output shown below.
Output
The above line of code will generate the following output −
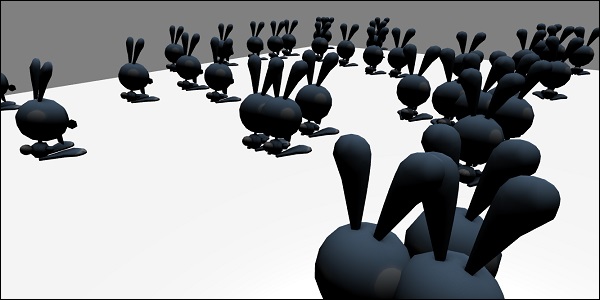
Explanation
Following code helps to make identical meshes in your scene −
var array_instances = []; // Trees BABYLON.SceneLoader.ImportMesh("", "scenes/", "Rabbit.babylon", scene, function (newMeshes, particleSystems, skeletons) { var rabbit = newMeshes[1]; rabbit.isVisible = false; var range = 50; var count = 100; for (var index = 0; index < count; index++) { var newInstance = rabbit.createInstance("i" + index); // creates new instance var x = range / 2 - Math.random() * range; var z = range / 2 - Math.random() * range; newInstance.position = new BABYLON.Vector3(x, 0, z); // sets the position of the instance created. newInstance.scaling = new BABYLON.Vector3(0.05, 0.05, 0.05);//scaling the instanc on x,y and z axis. array_instances.push(newInstance); } }); return scene;
babylonjs_mesh.htm
Advertisements