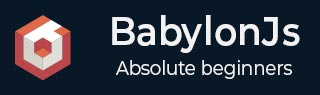
- BabylonJS Tutorial
- BabylonJS - Home
- BabylonJS - Introduction
- BabylonJS - Environment Setup
- BabylonJS - Overview
- BabylonJS - Basic Elements
- BabylonJS - Materials
- BabylonJS - Animations
- BabylonJS - Cameras
- BabylonJS - Lights
- BabylonJS - Parametric Shapes
- BabylonJS - Mesh
- VectorPosition and Rotation
- BabylonJS - Decals
- BabylonJS - Curve3
- BabylonJS - Dynamic Texture
- BabylonJS - Parallax Mapping
- BabylonJS - Lens Flares
- BabylonJS - Create ScreenShot
- BabylonJS - Reflection Probes
- Standard Rendering Pipeline
- BabylonJS - ShaderMaterial
- BabylonJS - Bones and Skeletons
- BabylonJS - Physics Engine
- BabylonJS - Playing Sounds & Music
- BabylonJS Useful Resources
- BabylonJS - Quick Guide
- BabylonJS - Useful Resources
- BabylonJS - Discussion
BabylonJS - Lights
In this chapter, we will learn about the lights used for BabylonJS. We will start by taking a look at the different types of lights available with babylonjs.
Lights are meant to produce the diffuse and specular color received by each pixel. Later, it is used on material to get the final color of each pixel.
There are 4 types of lights available with babylonjs.
- Point Light
- Directional Light
- Spot Light
- Hemispheric Light
BabylonJS - Point Light
A classic example of point light is the Sun, the rays of which are spread in all direction. Point light has a unique point in space from where it spreads the light in every direction. The color of light can be controlled using the specular and diffuse property.
Syntax
Following is the syntax for Point Light −
var light0 = new BABYLON.PointLight("Omni0", new BABYLON.Vector3(1, 10, 1), scene);
There are three different params for point light −
The 1st param is the name of the light.
The 2nd param is the position where the point light is placed.
The 3rd param is the scene to which the light needs to be attached.
Following properties are used to add color on the object created above −
light0.diffuse = new BABYLON.Color3(1, 0, 0); light0.specular = new BABYLON.Color3(1, 1, 1);
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .5, .5, .5); var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(0, 0, -0), scene); camera.setPosition(new BABYLON.Vector3(0, 0, -100)); camera.attachControl(canvas, true); var pl = new BABYLON.PointLight("pl", new BABYLON.Vector3(1, 20, 1), scene); pl.diffuse = new BABYLON.Color3(0, 1, 0); pl.specular = new BABYLON.Color3(1, 0, 0); var ground = BABYLON.Mesh.CreateGround("ground", 150, 6, 2, scene); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
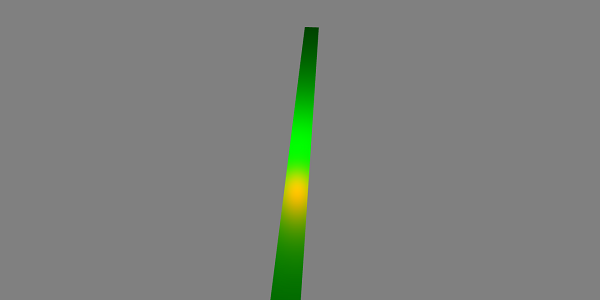
BabylonJS - The Directional Light
In directional light, the light is defined by the direction and is emitted in every direction based on where you place it.
var light0 = new BABYLON.DirectionalLight("Dir0", new BABYLON.Vector3(0, -1, 0), scene);
There are three different params for point light −
The 1st param is the name of the light.
The 2nd param is the position. Right now, it isplaced with negative -1 in the Y axis.
The 3rd param is the scene to be attached.
Here, you can add color with the specular and diffuse property.
light0.diffuse = new BABYLON.Color3(0, 1, 0); light0.specular = new BABYLON.Color3(1,0, 0);
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .5, .5, .5); var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(0, 0, -0), scene); camera.setPosition(new BABYLON.Vector3(0, 0, -100)); camera.attachControl(canvas, true); var pl = new BABYLON.DirectionalLight("Dir0", new BABYLON.Vector3(0, -10, 0), scene); pl.diffuse = new BABYLON.Color3(0, 1, 0); pl.specular = new BABYLON.Color3(1, 0, 0); var ground = BABYLON.Mesh.CreateGround("ground", 150, 6, 2, scene); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
The above line of code generates the following output −
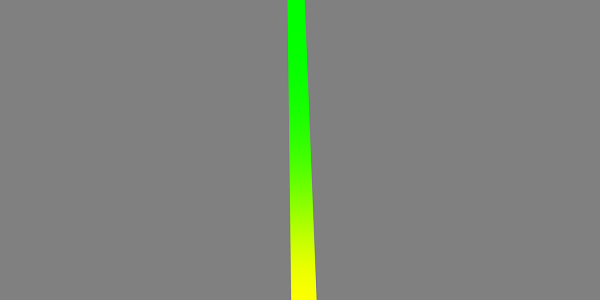
BabylonJS - The Spot Light
Spot light is just like light falling in cone shape.
Syntax
Following is the syntax for the Spot Light −
var light0 = new BABYLON.SpotLight("Spot0", new BABYLON.Vector3(0, 30, -10), new BABYLON.Vector3(0, -1, 0), 0.8, 2, scene);
There are five different params for point light −
- 1st Param is the name of the light.
- 2nd param is the position.
- 3rd param is the direction.
- 4th param is the angle.
- 5th param is the exponent.
These values define a cone of light starting from the position, emitting towards the direction. Specular and diffuse are used to control the color of the light.
light0.diffuse = new BABYLON.Color3(1, 0, 0); light0.specular = new BABYLON.Color3(1, 1, 1);
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .5, .5, .5); var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(0, 0, -0), scene); camera.setPosition(new BABYLON.Vector3(0, 0, -100)); camera.attachControl(canvas, true); var light0 = new BABYLON.SpotLight("Spot0", new BABYLON.Vector3(0, 30, -10), new BABYLON.Vector3(0, -1, 0), 0.8, 2, scene); light0.diffuse = new BABYLON.Color3(0, 1, 0); light0.specular = new BABYLON.Color3(1, 0, 0); var ground = BABYLON.Mesh.CreateGround("ground", 80,80, 2, scene); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
The above line of code generates the following output −
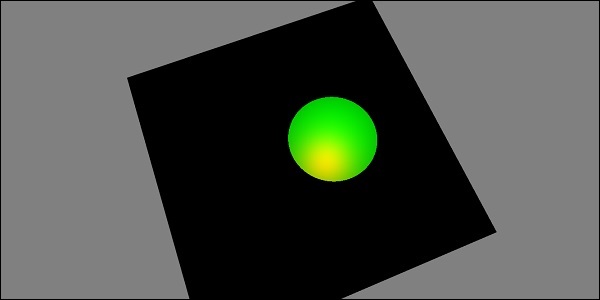
BabylonJS - The Hemispheric Light
A hemispheric light is more of getting the environment light. The direction of the light is towards the sky. 3 colors are given to the light; one for the sky, one for the ground and the last one for the specular.
Syntax
Following is the syntax for the Hemispheric Light −
var light0 = new BABYLON.HemisphericLight("Hemi0", new BABYLON.Vector3(0, 1, 0), scene);
For colors
light0.diffuse = new BABYLON.Color3(1, 0, 0); light0.specular = new BABYLON.Color3(0, 1, 0); light0.groundColor = new BABYLON.Color3(0, 0, 0);
Demo
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .5, .5, .5); var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(0, 0, -0), scene); camera.setPosition(new BABYLON.Vector3(0, 0, -100)); camera.attachControl(canvas, true); var light0 = new BABYLON.HemisphericLight("Hemi0", new BABYLON.Vector3(0, 1, 0), scene); light0.diffuse = new BABYLON.Color3(1, 0, 0); light0.specular = new BABYLON.Color3(0, 1, 0); light0.groundColor = new BABYLON.Color3(0, 0, 0); var ground = BABYLON.Mesh.CreateGround("ground", 100,100, 2, scene); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
The above line of code generates the following output −
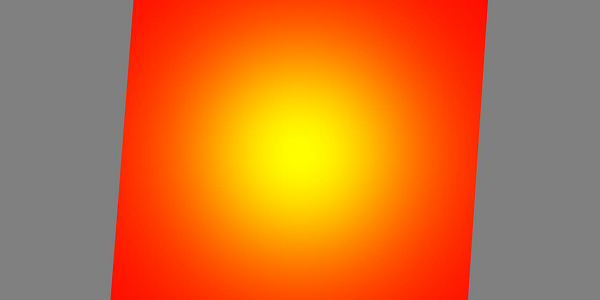