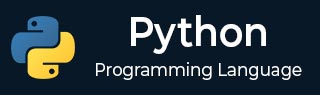
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python bool() function
The Python bool() function is a built-in function that returns a Boolean value (either True or False) based on the result of truth value testing procedure of the given object. It is not necessary to pass any arguments to this function and if we don't pass, it will return False.
The truth value testing for an object can be implemented using if or while conditions or a boolean operation. In the boolean operation, __bool__() and __len__() methods are used.
If an object does not have a __bool__() method, then its __len__() method is called, which returns the length of the object. If the length is zero, then the object is considered False, otherwise, it is considered True.
Syntax
Following is the syntax of the Python bool() function −
bool(object)
Parameters
The Python bool() function accepts a single parameter −
object − This parameter indicates an object such as a list, string, number or any expression.
Return Value
The Python bool() function returns either True or False depending on the given input.
Examples
Let's understand the working of bool() function with the help of some examples −
Example 1
The following example shows the basic usage of Python bool() function. If a number is not zero, it is considered true. Therefore, the code below will return false for zero and true for other numbers.
output1 = bool(111) print("The result of bool operation on positive num:", output1) output2 = bool(-68) print("The result of bool operation on negative num:", output2) output3 = bool(0) print("The result of bool operation on 0:", output3)
When we run above program, it produces following result −
The result of bool operation on positive num: True The result of bool operation on negative num: True The result of bool operation on 0: False
Example 2
In the code below, we have two strings and the bool() function is used to check whether the given strings are empty or non-empty. Since any non-empty string is considered True, this function will return False for an empty string and True for a non-empty string.
output1 = bool("Tutorialspoint") print("The result of bool operation on non-empty String:", output1) output2 = bool("") print("The result of bool operation on an empty String:", output2)
Following is an output of the above code −
The result of bool operation on non-empty String: True The result of bool operation on an empty String: False
Example 3
The code below shows how to use bool() function to check truth value of an empty list and a non-empty list.
output1 = bool([71, 87, 44, 34, 15]) print("The result of bool operation on non-empty List:", output1) output2 = bool([]) print("The result of bool operation on an empty List:", output2)
Output of the above code is as follows −
The result of bool operation on non-empty List: True The result of bool operation on an empty List: False
Example 4
In the following code, we are going to demonstrate how to use the __bool__() and bool() methods together to check if a person is a senior citizen based on their age.
class SeniorCitizen: def __init__(self, name, age): self.name = name self.age = age def __bool__(self): return self.age > 60 senCitzn1 = SeniorCitizen("Raman", 20) senCitzn2 = SeniorCitizen("Ramesh", 65) output1 = bool(senCitzn1) output2 = bool(senCitzn2) print("Is first person is senior citizen:", output1) print("Is second person is senior citizen:", output2)
Following is an output of the above code −
Is first person is senior citizen: False Is second person is senior citizen: True
To Continue Learning Please Login