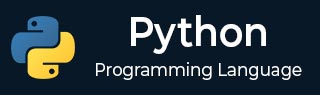
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Logging
The term "logging" refers to the mechanism of recording different intermediate events in a certain process. Recording logs in a software application proves helpful for the developer in debugging and tracing any errors in the application logic. Python's standard library includes logging module with which application logs can be generated and recorded.
It is a normal practice to use print() statements intermittently in a program to check intermediate values of different variables and objects. It helps the developer to verify if the program is behaving as per expectation or not. However, logging is more beneficial than the intermittent print statements as it gives more insight into the events.
Logging Levels
One of the important features of logging is that you can generate log message of different severity levels. The logging module defines following levels with their values.
Level | When it's used | Value |
---|---|---|
DEBUG |
Detailed information, typically of interest only when diagnosing problems. |
10 |
INFO |
Confirmation that things are working as expected. |
20 |
WARNING |
An indication that something unexpected happened, or indicative of some problem in the near future (e.g. 'disk space low'). The software is still working as expected. |
30 |
ERROR |
Due to a more serious problem, the software has not been able to perform some function. |
40 |
CRITICAL |
A serious error, indicating that the program itself may be unable to continue running. |
50 |
Example
The following code illustrates how to generate logging messages.
import logging logging.debug('This is a debug message') logging.info('This is an info message') logging.warning('This is a warning message') logging.error('This is an error message') logging.critical('This is a critical message')
It will produce the following output −
WARNING:root:This is a warning message ERROR:root:This is an error message CRITICAL:root:This is a critical message
Note that only the log messages after the WARNING level are displayed here. That is because root - the default logger ignores all severity levels above WARNING severity level. Notice severity level is logged before the first colons (:) of each line. Similarly, root is the name of the logger is also displayed the LogRecord.
Logging Configuration
The logs generated by the program can be customized with BasicConfig() method. You can define one or more of the following parameters for configuration −
filename − Specifies that a FileHandler be created, using the specified filename, rather than a StreamHandler.
filemode − If filename is specified, open the file in this mode. Defaults to 'a'.
datefmt − Use the specified date/time format, as accepted by time.strftime().
style − If format is specified, use this style for the format string. One of '%', '{' or '$' for printf-style, str.format() or string.Template respectively. Defaults to '%'.
level − Set the root logger level to the specified level.
errors − If this keyword argument is specified along with filename, its value is used when the FileHandler is created, and thus used when opening the output file. If not specified, the value 'backslashreplace' is used. Note that if None is specified, it will be passed as such to open(), which means that it will be treated the same as passing 'errors'.
Example
To log all the messages above DEBUG level, set the level parameter to logging.DEBUG
import logging logging.basicConfig(level=logging.DEBUG) logging.debug('This message will get logged')
It will produce the following output −
DEBUG:root:This message will get logged
To record the logging messages in a file instead of echoing them on the console, use filename parameter.
import logging logging.basicConfig(filename='logs.txt', filemode='w', level=logging.DEBUG) logging.warning('This messagewill be saved to a file')
No output will be displayed on the console. However, a logs.txt file is created in current directory with the text WARNING:root:This message will be saved to a file in it.
Variable Data in L ogging M essage
More often than not, you would like to include values of one or more variables in the logging messages to gain more insight into the cause especially of errors generated while the application is running. To do that, any of the dynamic string formatting techniques such as format() method of str class, or f-strings can be used.
Example
import logging logging.basicConfig(level=logging.DEBUG) marks = 120 logging.error("Invalid marks:{} Marks must be between 0 to 100".format(marks)) subjects = ["Phy", "Maths"] logging.warning("Number of subjects: {}. Should be at least three".format(len(subjects)))
It will produce the following output −
ERROR:root:Invalid marks:120 Marks must be between 0 to 100 WARNING:root:Number of subjects: 2. Should be at least three