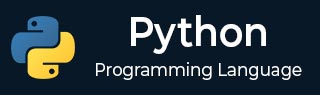
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Arithmetic Operators
In Python, numbers are the most frequently used data type. Python uses the same symbols for basic arithmetic operations Everybody is familiar with, i.e., "+" for addition, "-" for subtraction, "*" for multiplication (most programming languages use "*" instead of the "x" as used in maths/algebra), and "/" for division (again for the "÷" used in Mathematics).
In addition, Python defines few more arithmetic operators. They are "%" (Modulus), "**" (Exponent) and "//" (Floor division).
Python Arithmetic Operators
Arithmetic operators are binary operators in the sense they operate on two operands. Python fully supports mixed arithmetic. That is, the two operands can be of two different number types. In such a situation, Python widens the narrower of the operands. An integer object is narrower than float object, and float is narrower than complex object. Hence, the result of arithmetic operation of int and a float is a float. Result of float and a complex is a complex number, similarly, operation on an integer and a complex object results in a complex object.
Different Arithmetic Operators in Python
Following is the table which lists down all the arithmetic operators available in Python:
Operator | Name | Example |
---|---|---|
+ | Addition | a + b = 30 |
- | Subtraction | a – b = -10 |
* | Multiplication | a * b = 200 |
/ | Division | b / a = 2 |
% | Modulus | b % a = 0 |
** | Exponent | a**b =10**20 |
// | Floor Division | 9//2 = 4 |
Let us study these operators with examples.
Addition Operator (+)
This operator pronounced as plus, is a basic arithmetic operator. It adds the two numeric operands on the either side and returns the addition result.
Example: Addition of Two Integers
In the following example, the two integer variables are the operands for the "+" operator.
a=10 b=20 print ("Addition of two integers") print ("a =",a,"b =",b,"addition =",a+b)
It will produce the following output −
Addition of two integers a = 10 b = 20 addition = 30
Example: Addition of Integer and Float Numbers
Addition of integer and float results in a float.
a=10 b=20.5 print ("Addition of integer and float") print ("a =",a,"b =",b,"addition =",a+b)
It will produce the following output −
Addition of integer and float a = 10 b = 20.5 addition = 30.5
Example: Addition of Two Complex Numbers
The result of adding float to complex is a complex number.
a=10+5j b=20.5 print ("Addition of complex and float") print ("a=",a,"b=",b,"addition=",a+b)
It will produce the following output −
Addition of complex and float a= (10+5j) b= 20.5 addition= (30.5+5j)
Subtraction Operator (-)
This operator, known as minus, subtracts the second operand from the first. The resultant number is negative if the second operand is larger.
Example: Subtraction of Two Integers
First example shows subtraction of two integers.
a=10 b=20 print ("Subtraction of two integers:") print ("a =",a,"b =",b,"a-b =",a-b) print ("a =",a,"b =",b,"b-a =",b-a)
Result −
Subtraction of two integers a = 10 b = 20 a-b = -10 a = 10 b = 20 b-a = 10
Example: Subtraction of Integer and Float Numbers
Subtraction of an integer and a float follows the same principle.
a=10 b=20.5 print ("subtraction of integer and float") print ("a=",a,"b=",b,"a-b=",a-b) print ("a=",a,"b=",b,"b-a=",b-a)
It will produce the following output −
subtraction of integer and float a= 10 b= 20.5 a-b= -10.5 a= 10 b= 20.5 b-a= 10.5
Example: Subtraction of Two Complex Numbers
In the subtraction involving a complex and a float, real component is involved in the operation.
a=10+5j b=20.5 print ("subtraction of complex and float") print ("a=",a,"b=",b,"a-b=",a-b) print ("a=",a,"b=",b,"b-a=",b-a)
It will produce the following output −
subtraction of complex and float a= (10+5j) b= 20.5 a-b= (-10.5+5j) a= (10+5j) b= 20.5 b-a= (10.5-5j)
Multiplication Operator (*)
The * (asterisk) symbol is defined as a multiplication operator in Python (as in many languages). It returns the product of the two operands on its either side. If any of the operands negative, the result is also negative. If both are negative, the result is positive. Changing the order of operands doesn't change the result
Example: Multiplication of Two Integers
a=10 b=20 print ("Multiplication of two integers") print ("a =",a,"b =",b,"a*b =",a*b)
It will produce the following output −
Multiplication of two integers a = 10 b = 20 a*b = 200
Example: Multiplication of Integer and Float Numbers
In multiplication, a float operand may have a standard decimal point notation, or a scientific notation.
a=10 b=20.5 print ("Multiplication of integer and float") print ("a=",a,"b=",b,"a*b=",a*b) a=-5.55 b=6.75E-3 print ("Multiplication of float and float") print ("a =",a,"b =",b,"a*b =",a*b)
It will produce the following output −
Multiplication of integer and float a = 10 b = 20.5 a-b = -10.5 Multiplication of float and float a = -5.55 b = 0.00675 a*b = -0.037462499999999996
Example: Multiplication With Complex Number
For the multiplication operation involving one complex operand, the other operand multiplies both the real part and imaginary part.
a=10+5j b=20.5 print ("Multiplication of complex and float") print ("a =",a,"b =",b,"a*b =",a*b)
It will produce the following output −
Multiplication of complex and float a = (10+5j) b = 20.5 a*b = (205+102.5j)
Division Operator (/)
The "/" symbol is usually called as forward slash. The result of division operator is numerator (left operand) divided by denominator (right operand). The resultant number is negative if any of the operands is negative. Since infinity cannot be stored in the memory, Python raises ZeroDivisionError if the denominator is 0.
The result of division operator in Python is always a float, even if both operands are integers.
Example: Division of Two Integers
a=10 b=20 print ("Division of two integers") print ("a=",a,"b=",b,"a/b=",a/b) print ("a=",a,"b=",b,"b/a=",b/a)
It will produce the following output −
Division of two integers a= 10 b= 20 a/b= 0.5 a= 10 b= 20 b/a= 2.0
Example: Division With Float Numbers
In Division, a float operand may have a standard decimal point notation, or a scientific notation.
a=10 b=-20.5 print ("Division of integer and float") print ("a=",a,"b=",b,"a/b=",a/b) a=-2.50 b=1.25E2 print ("Division of float and float") print ("a=",a,"b=",b,"a/b=",a/b)
It will produce the following output −
Division of integer and float a= 10 b= -20.5 a/b= -0.4878048780487805 Division of float and float a= -2.5 b= 125.0 a/b= -0.02
Example: Division With Complex Number
When one of the operands is a complex number, division between the other operand and both parts of complex number (real and imaginary) object takes place.
a=7.5+7.5j b=2.5 print ("Division of complex and float") print ("a =",a,"b =",b,"a/b =",a/b) print ("a =",a,"b =",b,"b/a =",b/a)
It will produce the following output −
Division of complex and float a = (7.5+7.5j) b = 2.5 a/b = (3+3j) a = (7.5+7.5j) b = 2.5 b/a = (0.16666666666666666-0.16666666666666666j)
If the numerator is 0, the result of division is always 0 except when denominator is 0, in which case, Python raises ZeroDivisionError wirh Division by Zero error message.
a=0 b=2.5 print ("a=",a,"b=",b,"a/b=",a/b) print ("a=",a,"b=",b,"b/a=",b/a)
It will produce the following output −
a= 0 b= 2.5 a/b= 0.0 Traceback (most recent call last): File "C:\Users\mlath\examples\example.py", line 20, in <module> print ("a=",a,"b=",b,"b/a=",b/a) ~^~ ZeroDivisionError: float division by zero
Modulus Operator (%)
Python defines the "%" symbol, which is known aa Percent symbol, as Modulus (or modulo) operator. It returns the remainder after the denominator divides the numerator. It can also be called Remainder operator. The result of the modulus operator is the number that remains after the integer quotient. To give an example, when 10 is divided by 3, the quotient is 3 and remainder is 1. Hence, 10%3 (normally pronounced as 10 mod 3) results in 1.
Example: Modulus Operation With Integer Values
If both the operands are integer, the modulus value is an integer. If numerator is completely divisible, remainder is 0. If numerator is smaller than denominator, modulus is equal to the numerator. If denominator is 0, Python raises ZeroDivisionError.
a=10 b=2 print ("a=",a, "b=",b, "a%b=", a%b) a=10 b=4 print ("a=",a, "b=",b, "a%b=", a%b) print ("a=",a, "b=",b, "b%a=", b%a) a=0 b=10 print ("a=",a, "b=",b, "a%b=", a%b) print ("a=", a, "b=", b, "b%a=",b%a)
It will produce the following output −
a= 10 b= 2 a%b= 0 a= 10 b= 4 a%b= 2 a= 10 b= 4 b%a= 4 a= 0 b= 10 a%b= 0 Traceback (most recent call last): File "C:\Users\mlath\examples\example.py", line 13, in <module> print ("a=", a, "b=", b, "b%a=",b%a) ~^~ ZeroDivisionError: integer modulo by zero
Example: Modulus Operation With Float Values
If any of the operands is a float, the mod value is always float.
a=10 b=2.5 print ("a=",a, "b=",b, "a%b=", a%b) a=10 b=1.5 print ("a=",a, "b=",b, "a%b=", a%b) a=7.7 b=2.5 print ("a=",a, "b=",b, "a%b=", a%b) a=12.4 b=3 print ("a=",a, "b=",b, "a%b=", a%b)
It will produce the following output −
a= 10 b= 2.5 a%b= 0.0 a= 10 b= 1.5 a%b= 1.0 a= 7.7 b= 2.5 a%b= 0.20000000000000018 a= 12.4 b= 3 a%b= 0.40000000000000036
Python doesn't accept complex numbers to be used as operand in modulus operation. It throws TypeError: unsupported operand type(s) for %.
Exponent Operator (**)
Python uses ** (double asterisk) as the exponent operator (sometimes called raised to operator). So, for a**b, you say a raised to b, or even bth power of a.
If in the exponentiation expression, both operands are integer, result is also an integer. In case either one is a float, the result is float. Similarly, if either one operand is complex number, exponent operator returns a complex number.
If the base is 0, the result is 0, and if the index is 0 then the result is always 1.
Example of Exponent Operator
a=10 b=2 print ("a=",a, "b=",b, "a**b=", a**b) a=10 b=1.5 print ("a=",a, "b=",b, "a**b=", a**b) a=7.7 b=2 print ("a=",a, "b=",b, "a**b=", a**b) a=1+2j b=4 print ("a=",a, "b=",b, "a**b=", a**b) a=12.4 b=0 print ("a=",a, "b=",b, "a**b=", a**b) print ("a=",a, "b=",b, "b**a=", b**a)
It will produce the following output −
a= 10 b= 2 a**b= 100 a= 10 b= 1.5 a**b= 31.622776601683793 a= 7.7 b= 2 a**b= 59.290000000000006 a= (1+2j) b= 4 a**b= (-7-24j) a= 12.4 b= 0 a**b= 1.0 a= 12.4 b= 0 b**a= 0.0
Floor Division Operator (//)
Floor division is also called as integer division. Python uses // (double forward slash) symbol for the purpose. Unlike the modulus or modulo which returns the remainder, the floor division gives the quotient of the division of operands involved.
If both operands are positive, floor operator returns a number with fractional part removed from it. For example, the floor division of 9.8 by 2 returns 4 (pure division is 4.9, strip the fractional part, result is 4).
But if one of the operands is negative, the result is rounded away from zero (towards negative infinity). Floor division of -9.8 by 2 returns 5 (pure division is -4.9, rounded away from 0).
Example of Floor Division Operator
a=9 b=2 print ("a=",a, "b=",b, "a//b=", a//b) a=9 b=-2 print ("a=",a, "b=",b, "a//b=", a//b) a=10 b=1.5 print ("a=",a, "b=",b, "a//b=", a//b) a=-10 b=1.5 print ("a=",a, "b=",b, "a//b=", a//b)
It will produce the following output −
a= 9 b= 2 a//b= 4 a= 9 b= -2 a//b= -5 a= 10 b= 1.5 a//b= 6.0 a= -10 b= 1.5 a//b= -7.0
Precedence and Associativity of Python Arithmetic Operators
Operator(s) | Description | Associativity |
---|---|---|
** | Exponent Operator | Associativity of Exponent operator is from Right to Left. |
%, *, /, // | Modulus, Multiplication, Division, and Floor Division | Associativity of Modulus, Multiplication, Division, and Floor Division operators are from Left to Right. |
+, – | Addition and Subtraction Operators | Associativity of Addition and Subtraction operators are from Left to Right. |
The following table shows the precedence and associativity of the arithmetic operators in Python.
Complex Number Arithmetic
Arithmetic operators behave slightly differently when the both operands are complex number objects.
Addition and subtraction of complex numbers is a simple addition/subtraction of respective real and imaginary components.
a=2.5+3.4j b=-3+1.0j print ("Addition of complex numbers - a=",a, "b=",b, "a+b=", a+b) print ("Subtraction of complex numbers - a=",a, "b=",b, "a-b=", a-b)
It will produce the following output −
Addition of complex numbers - a= (2.5+3.4j) b= (-3+1j) a+b= (-0.5+4.4j) Subtraction of complex numbers - a= (2.5+3.4j) b= (-3+1j) a-b= (5.5+2.4j)
Multiplication of complex numbers is similar to multiplication of two binomials in algebra. If "a+bj" and "x+yj" are two complex numbers, then their multiplication is given by this formula −
(a+bj)*(x+yj) = ax+ayj+xbj+byj2 = (ax-by)+(ay+xb)j
For example,
a=6+4j b=3+2j c=a*b c=(18-8)+(12+12)j c=10+24j
The following program confirms the result −
a=6+4j b=3+2j print ("Multplication of complex numbers - a=",a, "b=",b, "a*b=", a*b)
To understand the how the division of two complex numbers takes place, we should use the conjugate of a complex number. Python's complex object has a conjugate() method that returns a complex number with the sign of imaginary part reversed.
>>> a=5+6j >>> a.conjugate() (5-6j)
To divide two complex numbers, divide and multiply the numerator as well as the denominator with the conjugate of denominator.
a=6+4j b=3+2j c=a/b c=(6+4j)/(3+2j) c=(6+4j)*(3-2j)/3+2j)*(3-2j) c=(18-12j+12j+8)/(9-6j+6j+4) c=26/13 c=2+0j
To verify, run the following code −
a=6+4j b=3+2j print ("Division of complex numbers - a=",a, "b=",b, "a/b=", a/b)
Complex class in Python doesn't support the modulus operator (%) and floor division operator (//).