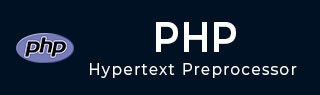
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Type Juggling
PHP is known as a dynamically typed language. The type of a variable in PHP changes dynamically. This feature is called "type juggling" in PHP.
In C, C++ and Java, you need to declare the variable and its type before using it in the subsequent code. The variable can take a value that matches with the declared type only.
Explicit type declaration of a variable is neither needed nor supported in PHP. Hence the type of PHP variable is decided by the value assigned to it, and not the other way around. Further, when a variable is assigned a value of different type, its type too changes.
Example 1
Look at the following variable assignment in PHP.
<?php $var = "Hello"; echo "The variable \$var is of " . gettype($var) . " type" .PHP_EOL; $var = 10; echo "The variable \$var is of " . gettype($var) . " type" .PHP_EOL; $var = true; echo "The variable \$var is of " . gettype($var) . " type" .PHP_EOL; $var = [1,2,3,4]; echo "The variable \$var is of " . gettype($var) . " type" .PHP_EOL; ?>
It will produce the following output −
The variable $var is of string type The variable $var is of integer type The variable $var is of boolean type The variable $var is of array type
You can see the type of "$var" changes dynamically as per the value assigned to it. This feature of PHP is called "type juggling".
Example 2
Type juggling also takes place during calculation of expression. In this example, a string variable containing digits is automatically converted to integer for evaluation of addition expression.
<?php $var1=100; $var2="100"; $var3=$var1+$var2; var_dump($var3); ?>
Here is its output −
int(200)
Example 3
If a string starts with digits, trailing non-numeric characters if any, are ignored while performing the calculation. However, PHP parser issues a notice as shown below −
<?php $var1=100; $var2="100 days"; $var3=$var1+$var2; var_dump($var3); ?>
You will get the following output −
int(200) PHP Warning: A non-numeric value encountered in /home/cg/root/53040/main.php on line 4
Type Casting vs Type Juggling
Note that "type casting" in PHP is a little different from "type juggling".
In type juggling, PHP automatically converts types from one to another when necessary. For example, if an integer value is assigned to a variable, it becomes an integer.
On the other hand, type casting takes place when the user explicitly defines the data type in which they want to cast.
Example
Type casting forces a variable to be used as a certain type. The following script shows an example of different type cast operators −
<?php $var1=100; $var2=(boolean)$var1; $var3=(string)$var1; $var4=(array)$var1; $var5=(object)$var1; var_dump($var2, $var3, $var4, $var5); ?>
It will produce the following output −
bool(true) string(3) "100" array(1) { [0]=> int(100) } object(stdClass)#1 (1) { ["scalar"]=> int(100) }
Example
Casting a variable to a string can also be done by enclosing in double quoted string −
<?php $var1=100.50; $var2=(string)$var1; $var3="$var1"; var_dump($var2, $var3); ?>
Here, you will get the following output −
string(5) "100.5" string(5) "100.5"