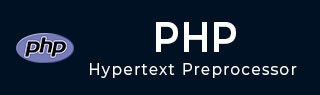
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Do…While Loop
The "do…while" loop is another looping construct available in PHP. This type of loop is similar to the while loop, except that the test condition is checked at the end of each iteration rather than at the beginning of a new iteration.
The while loop verifies the truth condition before entering the loop, whereas in "do…while" loop, the truth condition is verified before re entering the loop. As a result, the "do…while" loop is guaranteed to have at least one iteration irrespective of the truth condition.
The following figure shows the difference in "while" loop and "do…while" loop by using a comparative flowchart representation of the two.
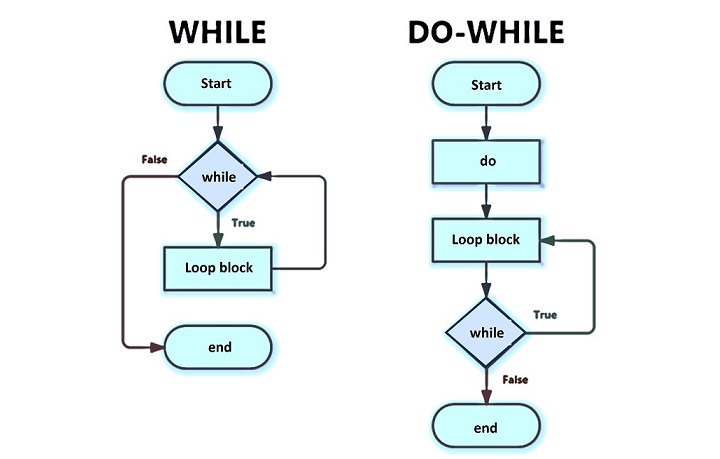
The syntax for constituting a "do…while" loop is similar to its counterpart in C language.
do { statements; } while (expression);
Example
Here is a simple example of "do…while" loop that prints iteration numbers 1 to 5.
<?php $i=1; do{ echo "Iteration No: $i \n"; $i++; } while ($i<=5); ?>
It will produce the following output −
Iteration No: 1 Iteration No: 2 Iteration No: 3 Iteration No: 4 Iteration No: 5
Example
The following code uses a while loop and also generates the same output −
<?php $i=1; while ($i<=5){ echo "<h3>Iteration No: $i</h3>"; $i++; } ?>
Hence, it can be said that the "do…while" and "while" loops behave similarly. However, the difference will be evident when the initial value of the counter variable (in this case $i) is set to any value more than the one used in the test expression in the parenthesis in front of the while keyword.
Example
In the following code, both the loops – while and "do…while" – are used. The counter variable for the while loop is $i and for "do…while" loop, it is $j. Both are initialized to 10 (any value greater than 5).
<?php echo "while Loop \n"; $i=10; while ($i<=5){ echo "Iteration No: $i \n"; $i++; } echo "do-while Loop \n"; $j=10; do{ echo "Iteration No: $j \n"; $j++; } while ($j<=5); ?>
It will produce the following output −
while Loop do - while Loop Iteration No: 10
The result shows that the while loop doesn’t perform any iterations as the condition is false at the beginning itself ($i is initialized to 10, which is greater than the test condition $i<=5). On the other hand, the "do…while" loop does undergo the first iteration even though the counter variable $j is initialized to a value greater than the test condition.
We can thus infer that the "do…while" loop guarantees at least one iteration because the test condition is verified at the end of looping block. The while loop may not do any iteration as the test condition is verified before entering the looping block.
Another syntactical difference is that the while statement in "do…while" terminates with semicolon. In case of while loop, the parenthesis is followed by a curly bracketed looping block.
Apart from these, there are no differences. One can use both these types of loops interchangeably.
Decrementing a "do…while" Loop
To design a "do…while" with decrementing count, initialize the counter variable to a higher value, use decrement operator (--) inside the loop to reduce the value of the counter on each iteration, and set the test condition in the while parenthesis to run the loop till the counter is greater than the desired last value.
Example
In the example below, the counter decrements from 5 to 1.
<?php $j=5; do{ echo "Iteration No: $j \n"; $j--; } while ($j>=1); ?>
It will produce the following output −
Iteration No: 5 Iteration No: 4 Iteration No: 3 Iteration No: 2 Iteration No: 1
Traverse a String in Reverse Order
In PHP, a string can be treated as an indexed array of characters. We can extract and display one character at a time from end to beginning by running a decrementing "do…while" loop as follows −
<?php $string = "TutorialsPoint"; $j = strlen($string); do{ $j--; echo "Character at index $j : $string[$j] \n"; } while ($j>=1); ?>
It will produce the following output −
Character at index 13 : t Character at index 12 : n Character at index 11 : i Character at index 10 : o Character at index 9 : P Character at index 8 : s Character at index 7 : l Character at index 6 : a Character at index 5 : i Character at index 4 : r Character at index 3 : o Character at index 2 : t Character at index 1 : u Character at index 0 : T
Nested "do…while" Loops
Like the for loop or while loop, you can also write nested "do…while" loops. In the following example, the upper "do…while" loop counts the iteration with $i, the inner "do…while" loop increments $j and each time prints the product of $i*j, thereby printing the tables from 1 to 10.
<?php $i=1; $j=1; do{ print "\n"; do{ $k = sprintf("%4u",$i*$j); print "$k"; $j++; } while ($j<=10); $j=1; $i++; } while ($i<=10); ?>
It will produce the following output −
1 2 3 4 5 6 7 8 9 10 2 4 6 8 10 12 14 16 18 20 3 6 9 12 15 18 21 24 27 30 4 8 12 16 20 24 28 32 36 40 5 10 15 20 25 30 35 40 45 50 6 12 18 24 30 36 42 48 54 60 7 14 21 28 35 42 49 56 63 70 8 16 24 32 40 48 56 64 72 80 9 18 27 36 45 54 63 72 81 90 10 20 30 40 50 60 70 80 90 100