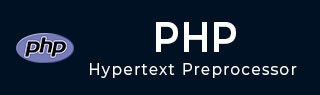
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP – Encryption
Early versions of PHP included mcrypt extension, that provided encryption/decryption capabilities. Due to lack of maintenance, the mycrypt extension has been deprecated and removed from PHP 7.2 version onwards. PHP now includes OpenSSL library that has an extensive functionality to support encryption and decryption features.
OpenSSL supports various encryption algorithms such as AES (Advanced Encryption Standard). All the supported algorithms can be obtained by invoking openssl_get_cipher_methods() function.
The two important functions in OpenSSL extension are −
openssl_encrypt() − Encrypts data
openssl_decrypt() − Decrypts data
The openssl_encrypt() Function
This function encrypts the given data with given method and key, and returns a raw or base64 encoded string −
openssl_encrypt( string $data, string $cipher_algo, string $passphrase, int $options = 0, string $iv = "", string &$tag = null, string $aad = "", int $tag_length = 16 ): string|false
The function has the following parameters −
Sr.No | Parameter & Description |
---|---|
1 | data The plaintext message data to be encrypted. |
2 | cipher_algo The cipher method. |
3 | passphrase The passphrase. If the passphrase is shorter than expected, padded with NULL characters; if the passphrase is longer than expected, it is truncated. |
4 | options options is a bitwise disjunction of the flags OPENSSL_RAW_DATA and OPENSSL_ZERO_PADDING. |
5 | iv A non-NULL Initialization Vector. |
6 | tag The authentication tag passed by reference when using AEAD cipher mode (GCM or CCM). |
7 | aad Additional authenticated data. |
8 | tag_length The length of the authentication tag. Its value can be between 4 and 16 for GCM mode. |
The function returns the encrypted string on success or false on failure.
The openssl_decrypt() Function
This function takes a raw or base64 encoded string and decrypts it using a given method and key.
openssl_decrypt( string $data, string $cipher_algo, string $passphrase, int $options = 0, string $iv = "", ?string $tag = null, string $aad = "" ): string|false
The openssl_decrypt() function uses the same parameters as the openssl_encrypt function.
This function returns the decrypted string on success or false on failure.
Example
Take a look at the following example −
<?php function sslencrypt($source, $algo, $key, $opt, $iv) { $encstring = openssl_encrypt($source, $algo, $key, $opt, $iv); return $encstring; } function ssldecrypt($encstring, $algo, $key, $opt, $iv) { $decrstring = openssl_decrypt($encstring, $algo, $key, $opt, $iv); return $decrstring; } // string to be encrypted $source = "PHP: Hypertext Preprocessor"; // Display the original string echo "Before encryption: " . $source . "\n"; $algo = "BF-CBC"; $opt=0; $ivlength = openssl_cipher_iv_length($algo); $iv = random_bytes($ivlength); $key = "abcABC123!@#"; // Encryption process $encstring = sslencrypt($source, $algo, $key, $opt, $iv); // Display the encrypted string echo "Encrypted String: " . $encstring . "\n"; // Decryption process $decrstring = ssldecrypt($encstring, $algo, $key, $opt, $iv); // Display the decrypted string echo "Decrypted String: " . $decrstring; ?>
It will produce the following output −
Before encryption: PHP: Hypertext Preprocessor Encrypted String: Decrypted String: