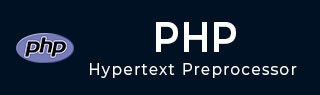
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP – Access Modifiers
In PHP, the keywords public, private and protected are known as the access modifiers. These keywords control the extent of accessibility or visibility of the class properties and methods. One of these keywords is prefixed while declaring the member variables and defining member functions.
Whether the PHP code has free access to a class member, or it is restricted from getting access, or it has a conditional access, is determined by these keywords −
Public − class members are accessible from anywhere, even from outside the scope of the class, but only with the object reference.
Private − class members can be accessed within the class itself. It prevents members from outside class access even with the reference of the class instance.
Protected − members can be accessed within the class and its child class only, nowhere else.
The principle of data encapsulation is the cornerstone of the object-oriented programming methodology. It refers to the mechanism of keeping the data members or properties of an object away from the reach of the environment outside the class, allowing controlled access only through the methods or functions available in the class.
To implement encapsulation, data members of a class are made private and the methods are made public.
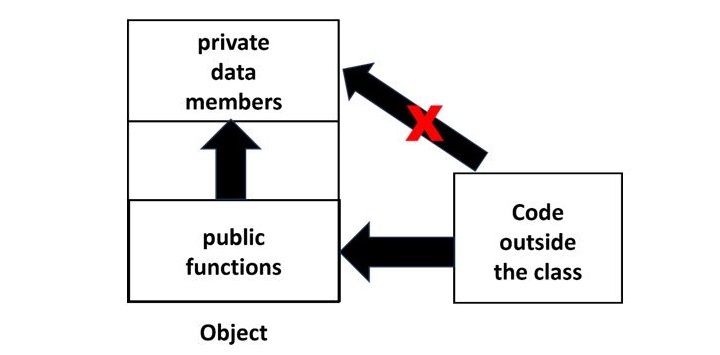
Public Members
In PHP, the class members (both member variables as well as member functions) are public by default.
Example
In the following program, the member variables title and price of the object are freely accessible outside the class because they are public by default, if not otherwise specified.
<?php class Book { /* Member variables */ var $price; var $title; /*Constructor*/ function __construct(string $param1="PHP Basics", int $param2=380) { $this->title = $param1; $this->price = $param2; } function getPrice() { echo "Title: $this->price \n"; } function getTitle() { echo "Price: $this->title \n"; } } $b1 = new Book(); echo "Title : $b1->title Price: $b1->price"; ?>
It will produce the following output −
Title : PHP Basics Price: 380
Private Members
As mentioned above, the principle of encapsulation requires that the member variables should not be accessible directly. Only the methods should have the access to the data members. Hence, we need to make the member variables private and methods public.
<?php class Book { /* Member variables */ private $price; private $title; /*Constructor*/ function __construct(string $param1="PHP Basics", int $param2=380) { $this->title = $param1; $this->price = $param2; } public function getPrice() { echo "Price: $this->price \n"; } public function getTitle() { echo "Title: $this->title \n;"; } } $b1 = new Book(); $b1->getTitle(); $b1->getPrice(); echo "Title : $b1->title Price: $b1->price"; ?>
Output
Now, the getTitle() and getPrice() functions are public, able to access the private member variables title and price. But, while trying to display the title and price directly, an error is encountered as they are not public.
Title: PHP Basics Price: 380 Fatal error: Uncaught Error: Cannot access private property Book::$title in hello.php:31
Protected Members
The effect of specifying protected access to a class member is effective in case of class inheritance. We know that public members are accessible from anywhere outside the class, and private members are denied access from anywhere outside the class.
The protected keyword grants access to an object of the same class and an object of its inherited class, denying it to any other environment.
Let us set the title member in Book class example to protected, leaving price to private.
class Book { /* Member variables */ private $price; protected $title; # rest of the code kept as it is } $b1 = new Book(); $b1->getTitle(); $b1->getPrice();
PHP allows the both the member variables to be accessed, as the object belongs to the same class.
Let us add a mybook class that inherits the Book class −
class mybook extends Book { # no additional members defined }
whose object is still able to access the member variables, as the child class inherits public and protected members of the parent class.
However, make mybook class as an independent class (not extending Book class) and define a getmytitle() function that tries to access protected title member variable of Book class.
class mybook { public function getmytitle($b) { echo "Title: $b->title <br/>"; } } $b1 = new mybook(); $b = new Book(); $b1->getmytitle($b);
As the getmytitle() function tries to print title of Book object, an error message showing Cannot access protected property Book::$title is raised.
Example
Try to run the following code −
<?php class Book { private $price; protected $title; function __construct(string $param1="PHP Basics", int $param2=380) { $this->title = $param1; $this->price = $param2; } public function getPrice(){ echo "Price: $this->price <br/>"; } public function getTitle(){ echo "Title: $this->title <br/>"; } } class mybook { public function getmytitle($b) { echo "Title: $b->title <br/>"; } } $b1 = new mybook(); $b = new Book(); $b1->getmytitle($b); ?>
It will produce the following output −
PHP Fatal error: Uncaught Error: Cannot access protected property Book::$title in /home/cg/root/97848/main.php:18
Hence, it can be seen that the protected member is accessible by object of same class and inherited class only. For all other environment, protected members are not accessible.
The accessibility rules can be summarized by the following table −
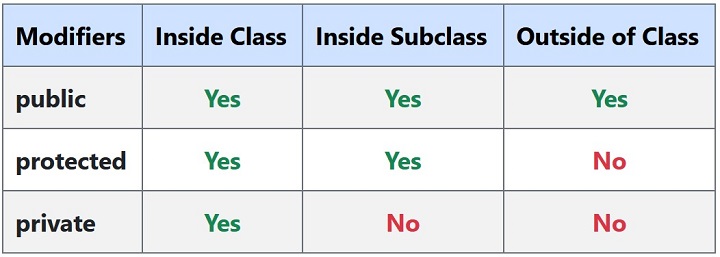